In this tutorial, we are going to learn writing the python program to calculate the simple interest from given principal, rate of interest, and time.
Problem Statement
For the inputs given by the user, calculate the simple interest.
For example:
Case1: If the user has given input, the principal value = 1900, rate of interest = 2 and time = 2,
Then the output should be ’76.0′.
Case2: If the user input the principal value = 700, rate of interest = 3 and time = 10,
Then the output should be ’210.0′.
[elementor-template id=”5253″]
Our logic to calculate simple interest
- Our program will take principal, rate of interest and time value from the user.
- By using the values given by the user, our program will calculate the value of simple interest and return the simple interest as the output of our program.
The common formula to calculate simple interest is :
Simple interest = (principal x rate of interest x time)/100
[elementor-template id=”5256″]
Algorithm to calculate simple interest
Step 1: Start
Step 2: Take the values of principal, rate of interest, and time values input from the user.
Step 3: Use the formula of simple interest( Simple interest = (principal x rate of interest x time)/100 ) to calculate the simple interest value.
Step 4: print the simple interest value.
Step 5: Stop
Python code to calculate simple interest
[elementor-template id=”5257″]
Output 1:
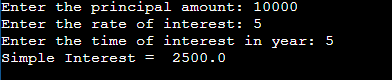
Explanation:
For the input value of the principal, the rate of interest and the time simple interest is calculated using the formula
Simple interest = (10000 * 5 *5)/100
Simple interest = 2500.0
Output 2:
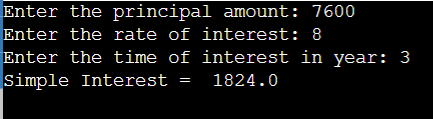
Explanation:
For the input value of the principal, the rate of interest and the time simple interest is calculated using the formula
Simple interest = (7600 * 8 *3)/100
Simple interest = 1824.0