In this tutorial we will learn writing program in python to create an array (list in case of python) and return the reverse of elements stored in the array (list).
Problem Statement
Our program will first take the input of array (list) size and then the elements of the array (list). And return the reverse of the input array (list).
For example:
Case 1: if the user inputs 4 as array (list) size and the array (list) elements as 1,2,3,4.
The output should be 4,3,2,1.
Case 2: if the user inputs 5 as array (list) size and the array (list) elements as 9,8,7,6,5.
The output should be 5,6,7,8,9.
Our logic to print an array(list) in reverse order
- Our program will take input to fix the size of the array (list).
- Then our program will run for a ‘for loop’ to take the inputs from the user. The inputs will be the elements (or the content) of the array (list).
- Then, our program will reverse the elements using the range function in for loop.
Algorithm to print an array(list) in reverse order
Step 1: Start
Step 2: take input from the user (let’s say size).
Step 3: Create an empty list.
Step 4: for i in range(0,size):
elem=int(input(“Please give value for index “+str(i)+”: “))
arr.append(elem)
Step 5: print the reversed list.
for i in range(size-1, -1, -1):
print(arr[i],end=’ ‘)
Step 6: Stop
Python code to print an array(list) in reverse order
Output 1:
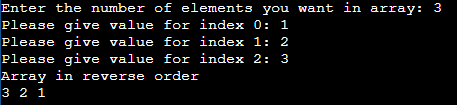
Explanation:
The input list is [1, 2, 3 ] which is given by the user. The range() function with arguments (size-1,-1,-1) will read the elements of the array from the end of the list. So the output will be printed from the last element of the list. so the output will be 3 2 1.
Output 2:
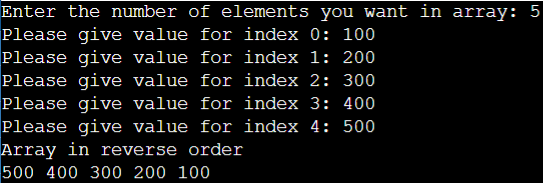
Explanation:
The input list is [100, 200, 300, 400, 500 ] which is given by the user. The range() function with arguments (size-1,-1,-1) will read the elements of the string from the end of the list. So the output will be printed from the last element of the list. So the output will be 500 400 300 200 100.