If Your are struggling to find the most important and frequently asked face two face c interview questions, Then hold down and take a deep breath because you are at the right place.
I know that It is very tough for fresher students to prepare for an interview as well as finding some great bunch of right questions that cover the most important topics.
In this section, I have tried to cover all important and most asked c programming interview questions topics wise.
Let us start Preparing.
Basic C interview questions
Q1). What is C Programming Language?
Ans:
C is an imperative procedural Programming language. This is designed by Dennis Ritchie. C was originally developed by Dennis Ritchie between 1969 and 1973 at AT & T Bell Labs. C Language was first appeared in 1972. It was mainly developed as a system programming language to write OS (operating system).
Q2). Write some features of C Programming Language.
Ans: The features of the C programming Language are:
- Portability:It means that we can easily run programs written on one platform on the other platform. Ex- A sum of number programs written on Turbo C++ can run on Codeblocks.
- Simple: Simple is also a feature of C programming language because it is very easy to learn and understand the programming concepts and build the logics.
- Memory Management: Memory management is also a good features of c programming language. It supports both static as well as dynamic memory allocation. Using the concept of dynamic memory allocation we can allocate memory as per requirement and can free useless allocated memory space by calling free() function.
- Speed: The compilation and execution time of the c language is fast as compared to other programming languages like Python or Java.
- Case Sensitive: The meaning of both Upper and Lower case letters is different. EX: If we assign value 4 to lowercase x and try to access with uppercase X then we encounter error.
- Rich Library: C language has a lot of in-built functions which makes development fast.
Q3). What do you mean by Data Type in C?
Ans:
Data Type defines which type of value a variable can store. In C programming language each variable is associated with a data type and each data type requires different size of memory.
Some most usable data type:
char: char shows the variable of type char stores single character. char requires a one byte of memory.
Ex: char ch;
Here ch is variable and having data type “char”.
int: int shows the variable having data type int stores integer value. int requires a four byte of memory.
Ex: int i;
here i is variable of type integers.
float: float is used to store decimal numbers with single precision. float requires a four byte of memory.
Ex:float f;
Here f is variable of type float.
double: double is used to store decimal numbers with double precision.
Ex: double d;
Here d is variable of type double.
Q4). How many types of data type in C Language?
Ans:
C language supports 2 type of data types:
a). Primary data types:
Primary data types are known as fundamental data types.Some primary data types are integer(int), floating point(float), character(char) and void.
b).Derived data types:
Derived data types are derived from primary datatypes. Some derived data types are array, structure, union and pointer.
Q5). What is constant and variable in C .
Ans:
Constant: A constant is a value or an identifier whose value cannot be changed in a program. identifier also can be defined as a constant.
For example:
const double PI = 3.14
Here, PI is a constant. And the PI contains 3.14 for this program.
Variables
A variables is a value or an identifier whose value can be changed in a program. Variable is a container to hold data.
For example:
int score = 6;
Here, score is a variable of integer type and it contains value 6. The value of score can be change so here score is variable.
Q6) What is the use of printf() and scanf() functions?
Ans: printf(): The printf() function is used to print values on to the screen(console). Value may be anythings like integer, float, character and string.
scanf():The scanf() function is used to take input from the user.
Q7). What is the difference between the local variable and global variable in C.
Ans:
local variable: It is defined inside a function. It is accessible only in the function in which it is defined.
Global variable : It is defined outside of all the functions in a program. It is available to all the functions in the program.
Q8) Write format specifiers in C.
Ans: %d: It is used to print an integer value.
%s: It is used to print a string.
%c: It is used to display a character value.
%f: It is used to display a floating point value.
Q9) How many spaces a Tab has in C.
Ans: A Tab has 8 spaces.
Q10) What is static variable in C? What is its use?
Ans: static keyword is used to declared static variable. Static variables preserves their value outside of their scope. Hence, static variables preserve their previous value in their previous scope and are not initialized again in the new scope.Scope of the static variable is available in the entire program. So, we can access a static variable anywhere in the program.
The initial value of the static variable is zero. The static variable shares common value with all the methods.The static variable is initialized only once in the memory heap to reduce the memory usage.
Q11) Can we compile and execute a program without main() function?
Ans: Yes, we can compile and execute using macros.
#include <stdlib.h>
#include <stdio.h>
#define fun main
int fun(void)
{
printf("Quescol");
return 0;
}
Output:
Quescol
Q12) Difference between getc(), getchar(), getch() and getche().
Ans: All of these functions read a character from input and return an integer value. The integer is returned to accommodate a special value used to indicate failure. The value EOF is generally used for this purpose.
1). getc(): It reads a single character from a given input stream and on success returns the integer value. And on failure it returns EOF.
Syntax:
int getc(FILE *stream);
Example:
#include <stdio.h>
int main()
{
printf("%c", getc(stdin));
return(0);
}
Input: a (press enter key)
Output: a
2). getchar():
getchar() reads from standard input. it returns only single charater from input stream. Also getchar() is equivalent to getc(stdin).
Syntax:
int getchar(void);
Example:
#include <stdio.h>
int main()
{
printf("%c", getchar());
return 0;
}
Input: a(press enter key)
Output: a
3). getch():
getch() is a nonstandard function.It is present in conio.h header file. It is not a part of the C standard library. getch() reads a single character from keyboard. It does not use any buffer, so when we give a character as input it immediately returned without waiting for the enter key.
Syntax:
int getch();
Example:
#include <stdio.h>
#include <conio.h>
int main()
{
printf("%c", getch());
return 0;
}
Input: a
Output: Program terminates immediately. But when you go on DOS shell in Turbo C, you see single ‘a’ as output.
4). getche():
getche() is also a non-standard function which is present in conio.h header file. When you give a character as a input from the keyboard it displays immediately on output screen without pressing enter key.
Syntax:
int getche(void);
Example:
#include <stdio.h>
#include <conio.h>
int main()
{
printf("%c", getche());
return 0;
}
Input: a
Output: Program terminates immediately. But when you go on DOS shell in Turbo C, you see double ‘a’ as output i.e. aa.
Q13). What is typecasting?
Ans: The typecasting is a process of converting one data type into another data type. Suppose we want to store the floating type value to an int type, then we will use typecasting to convert floating point data type into an int data type explicitly.
Ex: int i = (int) 3.4
Q14). Write a program to print “hello world” without using a semicolon?
Ans:
#include<stdio.h>
void main(){
if(printf("hello world")){}
}
Output:
hello world
Q15). What is the difference between the = symbol and == symbol?
Ans:The single = symbol is an assignment operator which is used to assign value to a variable whereas the double == symbol is an relational operator which is used to compare the two values, is also called “equal to” or “equivalent to”.
Q16). What is C Token?
Ans: In c, Keywords, Constants, Special Symbols, Strings, Operators, Identifiers are referred as Tokens.
Q17). Where can we not use &(address operator in C)?
Ans: We cannot use ‘&’ on constants and on a variable which is declared using the register storage class.
Q18). What is l-value and r-value?
Ans: The term l-value and r-value are named because of their appearance in the expression.
L-value appeared on the left side of the assignment operator. Basically in general case variable is considered as L-value in which we are going to assign some values.
R-value appeared on the right side of the assignment operator.
Example: In this expression “x = 15”, x is considered as l-value and 15 is as r-value.
Q19). What is the difference between puts() and printf()?
Ans:
puts() | printf() |
1. The puts() function only allows you to print a string on the console output screen. | 1. The printf() function can print strings as well as mixed types of variables on the console output screen. |
2. The syntax for puts:
puts(str) | 2. The syntax for printf:
printf(str) |
3. The puts() function prints string and automatically adds one new character at the end of the string that is a new line character(n). | 3. The printf() function prints text, string, text+ value, etc, without adding extra character at the end. |
4. Its implementation is simpler than printf. | 4. Its implementation is complex as compared to puts. |
C interview questions on statements and loops
Q1). Explain IF-Else Statement in C Language.
Ans:IF-Else Statement works as a control flow statement in C. if statement is followed by an optional else statement.
Syntax :
if(boolean_expression) { /* statement s1 will execute if the boolean_expression is true */ } else { /* statement s2 will execute if the boolean_expression is false */ }
If the Boolean_expression is true, then the if block will be executed, otherwise, the else block will be executed.
Q2). What is a nested loop?
Ans:It is also a type of loop which runs within another loop is called nested loop.
Syntax :
for(initialization; condition; increment/decrement) { Statements for(initialization; condition; increment/decrement) { statements } }
Q3). Break and continue in C Language with example.
Ans:
1). break:
break statement terminates the loop when it is encountered. The break statement can be used with decision-making statements such as if…else and loop like for, while and do..while.
#include <stdio.h>
int main()
{
int i, n, sum = 0;
for(i=1; i <= 5; ++i)
{
printf("Enter a n%d: ",i);
scanf("%d",&n);
if(n > 6)
{
break;
}
sum += n;
}
printf("Sum = %d",sum);
return 0;
}
Output:
Enter a n1: 4
Enter a n2: 2
Enter a n3: 1
Enter a n4: 3
Enter a n5: 5
Sum = 15
2). continue :
The continue statement is used to skips some statements inside the loop. The continue statement is used with decision making statement such as if…else.
#include <stdio.h>
int main()
{
int i,n, sum = 0;
for(i=1; i <= 5; ++i)
{
printf("Enter a n%d: ",i);
scanf("%d",&n);
if(n < 5)
{
continue;
}
sum += n;
}
printf("Sum = %d",sum);
return 0;
}
Output:
Enter a n1: 3
Enter a n2: 4
Enter a n3: 1
Enter a n4: 5
Enter a n5: 7
Sum = 12
Q4). What are the Valid Places where the Programmer can apply Break Control Statement?
Ans:It is valid to be used inside a loop and Switch control statements.
Q5). Switch in C Language with Example.
Ans: A switch statement allows to test a variable for equality against a list of cases. It is good practice to use a switch statement than nested if…else . Because the switch is faster than nested if…else(not always).
#include <stdio.h>
int main () {
int division = 3;
switch(division) {
case 1 :
printf("1st Divisionn" );
break;
case 2 :
printf("2nd Divisionn" );
break;
case 3 :
printf("3rd Divisionn" );
break;
default :
printf("runner upn" );
}
return 0;
}
Output:
3rd Divisionn
Q6). While Loop in C Language with Example.
Ans: A while loop is used to execute a statement multiple times as long as a given condition is true.
#include <stdio.h>
int main () {
int a = 0;
while( a < 10 ) {
printf("value of a: %d \n", a);
a++;
}
return 0;
}
Output:
value of a: 0
value of a: 1
value of a: 2
value of a: 3
value of a: 4
value of a: 5
value of a: 6
value of a: 7
value of a: 8
value of a: 9
Q7). What is the difference between while (0) and while (1)?
Ans: The term while is a conditional-based loop, While(0) means the condition of the while loop is false so it will never execute a particular section of the code inside the program. Whereas while(1) is the opposite of while(0) and it will execute the particular section of code infinite times.
Q8). Difference b/w Entry controlled and Exit controlled loop?
Ans: In the Entry controlled loop first the loop condition is checked then after the body of the loop is executed whereas in the Exit controlled loop first the body of the loop is executed then after the condition is checked.
Entry Controlled Loops are : for, a while
Exit Controlled Loop is : do-while
Q9). Can we use a continue statement without using a loop?
Ans:
No, we cannot use continue statements without using a loop, it can be used within any loop whether its while , for , or Do-while loop. In case if we try to use continue without using loop, there will be a compile error i.e”Misplaced continue”.
Q10) Explain for Loop in C Language with an example.
Ans: A for loop is used to execute a statement for a specific number of times.
#include <stdio.h>
int main () {
int i;
for( i = 0; i < 10; i++ ){
printf("value of a: %dn", i);
}
return 0;
}
Output:
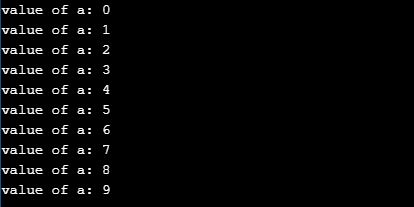
Q11). What is the difference between ++a and a++.
Ans:‘++a’ is called prefix increment. In this, first value of the variable “a” gets incremented and then assigned to the variable a. On the other hand “a++” is called post increment.The value stored in the variable “a” gets incremented after the execution of the particular line.
Also Prepare these c interview questions that are equally important:
Frequently Asked Pattern Programming In C
Important Coding Questions in C
C Interview questions on Functions
Q1). What is a function? Why to use function.
Ans:A function is a block of code which performs some specific task. Uses of C function are: functions helps to avoid the rewriting the same code again and again in our program. functions can be called from any place of our program and any number of times. functions helps to make code more readable and simple.
#include<stdio.h>
void hello(){
printf("hello c programming n");
}
int main(){
hello();
return 0;
}
Output:
hello c programming n
Q2). What is Recursion in C?
Ans: When a function calls itself, and this process is known as recursion. The function that calls itself is known as a recursive function.
#include<stdio.h>
int fibonacci(int);
int main(){
int n, i, k;
printf("Enter the number to print fibonacci series: ");
scanf("%d",&n);
for(i=0;i<n;i++) {
printf("%d ",fibonacci(i));
}
}
int fibonacci(int i){
if(i==0)
return 0;
else if(i==1)
return 1;
else
return (fibonacci(i-1)+fibonacci(i-2));
}
Output:

Q3). What are called and calling functions?
Ans: Parent function which calls any function is known as called function and the function which is being called is known as the calling function.
int main()
{
name_Func()
}
Here, name_Func() is called within the main() function, so main() is called function, while name_Func() is calling function.
Q4). Are exit () and return statements same in function definition?
Ans: No, both are different as we used exit() to immediately exit from the program, whereas return is used to return the control of the programs execution from the called function to the calling function.
Q5). When is the “void” keyword used in a function?
Ans: When we declare the function we have to decide whether we want some return value from the function or not, if we only want to print some value or statements on the screen we use void on the left side of the function name otherwise we place the data type of the value on the left side of function name.
Q6). Differentiate between call by value and call by reference.
Ans:
Factor | Call by Value | Call by Reference |
Safety | In call by value actual parameters are safe because operations are performed on formal parameter. | In call by value operations are performed on actual parameters so it is not safe here. |
Memory Location | In this separate memory locations are created for actual and formal parameters | In this actual and Formal parameters share the same memory space. |
Parameters | In this copy of actual parameters are passed. | In this actual parameters are passed. |
Q7). Explain rand() function in C. Write a program in C to generate a random number.
Ans: The rand() function in c is used to generate a random number.
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int x;
for(x=1;x<=5;x++)
{
printf("%d \n",rand());
}
return 0;
}
Output:
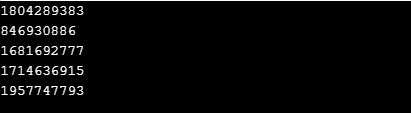
C Interview questions on Array and Pointer
Q1). What is an Array in C?
Ans:An Array is a collection of similar types of elements i.e. data having similar type of data type. Array takes memory in contiguous fashion to store data. We cannot change the size and type of arrays after its declaration.
Array are of two types:
One-dimensional array: One-dimensional array is an array that stores the elements one after the another in rowwise .
Syntax:
int arr[size];
Multidimensional array: Multidimensional array is a collection of more than one one-dimensional array.
Syntax:
int arr[size][size];
Q2) What is a Pointer in C?
Ans:
A pointer is a variable that contains the address of another variable. We use variable of pointer type when we want to store direct address of any variable’s memory locaion.
For example:
int *p,i;
p = &i;
The above syntax show that p is a pointer variable. And p can store the address of variable i.
One more things always keep in mind data type of pointer variable and variable must be same. Pointer variable can store only the similar type of datatype.
Example of pointer:
#include <stdio.h>
int main()
{
int *p;
int i=1;
p=&i;
printf("Address of 'i' variable is %p",p);
return 0;
}
Output:
Address of 'i' variable is 0x7ffdfe6dddec
Q3) What is a NULL pointer in C?
Ans:A pointer that doesn’t refer to any address of value but NULL is known as a NULL pointer. When we assign a ‘0’ value to a pointer of any type, then it becomes a Null pointer.
Q4) What is a far pointer in C?
Ans:A pointer which can access all the 16 segments of RAM is known as far pointer. A far pointer is a 32-bit pointer that obtains information outside the memory in a given section.
Q5) What is a dangling pointer in C?
Ans:A pointer that doesn’t refer to any address of value but NULL is known as a NULL pointer. When we assign a ‘0’ value to a pointer of any type, then it becomes a Null pointer.
Q6). What is Wild Pointers in C.
Ans: Such pointers in C which are Uninitialized in c code are called Wild Pointers. In general, these pointers are pointing to the arbitrary memory location and cause the program to crash.
Q7) can a pointer access the array?
Ans:Pointers can access the array by holding the base address of the array.
8). Why a[5] == 5[a]?
Ans: As we know that
a[b] == *(a+b)
so
a[2] == *(a+2)
therefore,
2[a] = *(2+a)
Here is basically commutative law which is followed by it.
C interview Questions on Structure and Unions
Q1) What is a Structure in C?
Ans:
The structure is a user-defined data type. The structure allows combining data items of different kinds. It occupies the sum of the memory of all members in structure. Structure members can be accessed only through structure variables.
Syntax of structure
struct structure_name
{
variable1;
variable2
.
.
variable n;
}[structure variables];
Let’s see a simple example
#include <stdio.h>
struct student
{
char name[5];
int roll_no;
}s1;
int main()
{
printf("Enter the name=");
scanf("%s",s1.name);
printf("Enter the roll_no=");
scanf("%d",&s1.roll_no);
printf("Name and Roll No of a student is: %s,%d",s1.name,s1.roll_no);
return 0;
}
Output:
Enter the name=quescol
Enter the roll_no=12
Name and Roll No of a student is: quescol,12
Q2). What is union in C?
Ans: The union is also user-defined data type. Union allows to combine data items of different kinds. The union doesn’t occupy the sum of the memory of all members. It holds only the memory of the largest member only. In a union, we can access only one variable at a time. It allocates one common space for all the members of a union.
Syntax of union struct union_name { variable1;variable2; . . variable n;}[union variables];
Let’s see a simple example
#include<stdio.h>
#include<string.h>
union Employee
{
char name[20];
float salary;
} emp;
int main()
{
strcpy(emp.name, "Prakash");
emp.salary = 28000;
printf("name = %s \n", emp.name);
printf("salary = %.1f", emp.salary);
return 0;
}
Output:
name =
salary = 28000.0
Q3). Difference between malloc() and calloc() functions?
Ans:
Malloc() | Calloc() |
1. The term malloc() stands for memory allocation. | 1. The term malloc() stands for contiguous allocation. |
2. It takes one argument, that is, the number of bytes. | 2. It takes two arguments i.e number of blocks and size of each block. |
3.syntax of malloc():
void *malloc(size_t n); Where n indicates allocates bytes of memory. If the allocation succeeds, a void pointer to the allocated memory is returned. Otherwise, NULL is returned. | 3.syntax of calloc():
void *calloc(size_t n, size_t size); Allocates a contiguous block of memory large enough to hold n elements of size bytes each. The allocated region is initialized to zero. |
4. It is faster than calloc. | 4. It is somewhat slower than malloc() as it takes extra steps to initialize the allocated memory by zero. |
Q4). What do you mean by a Nested Structure?
struct Date
{
int birth_date;
int birth_month;
int birth_year;
};
struct personal_recod
{
char name[25];
struct Date birth_date;
float salary;
}person;
C interview questions on file handling and memory allocation
Q1.) What is static memory allocation?
Ans: In static memory allocation, memory is allocated during compile time. And we cannot increase memory while executing the program. Array use the static memory allocation. lifetime of a variable in static memory is the lifetime of the program. It applies to global variables, file scope variables etc. Static memory is implemented using stacks or heap.
For example int arr[20]; The above example creates an array of integer type, and the size of an array is fixed, i.e., 20.
Q2) What is dynamic memory allocation?
Ans:In dynamic memory allocation, memory is allocated at runtime. We can increase memory while executing the program. The malloc() or calloc() function is used to allocate the memory at the runtime. Dynamic memory allocation is used in linked list.