Welcome to our latest blog post, “Java Interview Questions for Freshers and Experienced: MNCs Recommended.”. If Your are struggling to find the most important and frequently asked face two face Java interview questions that generally asked in Big Companies during hiring of Java developers, Then hold down and take a deep breath because you are at the right place.
I know that It is very tough for fresher and experienced to prepare for an interview as well as finding some great bunch of right questions that cover the most important topics. This article is designed to help you prepare for interviews at some of the world’s top multinational companies (MNCs) like TCS, WIPRO, Accenture, Cognizant, KPMG, Amazon, and IBM, all of which are known for their rigorous hiring processes and high standards.
The below listed code is best for both 2+ years experienced, 3+ years experience and for more experienced as well. It covers all the basics and mid level questions that mostly asked in interview. All these questions I have collected by attending multiple Java developers interview in MNCs. With the below set of questions there are highly chance you can clear first round of interview for the java developer role.
Java interview Questions for Experienced MNCs
Java Basics & Miscellaneous
1. What is the purpose of the static keyword in Java?
The static keyword in Java is used for elements that should exist independently of any specific instances of a class. This means if a field, method, or code block is declared static, it can be accessed without creating an object of the class.
Example
class Main{
static int count = 0; // Static variable
Main() {
count++;
}
}
Here, count
is a static variable shared by all instances of Main
.
2. What is the purpose of the static block in Java?
A static block initializes static variables and executes when the class is first loaded. It’s run only once, no matter how many objects of the class are created.
Example:
class Main{
static int count;
static {
count = 10; // Static block
}
}
3. What are the different access modifiers in Java? Explain their significance.
ccess modifiers in Java control the accessibility of classes, methods, and variables.
- Public: Accessible from any other class.
- Protected: Accessible within the same package or subclasses.
- Default (no modifier): Accessible only within the same package.
- Private: Accessible only within the class it is declared.
Example:
public class Car {
private int year; // Private access
}
4. How does Java handle memory management and garbage collection?
Java uses garbage collection to manage memory, which automatically clears memory that is no longer in use, preventing memory leaks.
Types of garbage collectors include:
- Serial Collector: Used in small applications with limited resources.
- Parallel Collector: Used for medium to large applications that need faster performance.
- G1 Collector: Used in large applications with a large amount of memory and processors.
5. What is the difference between String, StringBuilder, and StringBuffer?
String
Immutable, once created cannot be changed. Useful for constant strings.
Example
public class Main {
public static void main(String[] args) {
String s = "Hello";
System.out.println("Original String: " + s); // Output: Hello
// Attempting to modify the original string
String modified = s.concat(" Java");
System.out.println("Modified String: " + modified);
// Output: Hello World
System.out.println("Original String after modification: " + s);
// Output: Hello
}
}
- When
s.concat(" Java")
is called, it does not change the original strings
. Instead, it returns a newString
object which is pointed to bymodified
. - The original string
s
remains unchanged as “Hello”, demonstrating its immutabilit
StringBuilder
Mutable, can be changed and is not thread-safe. Ideal for strings that require frequent modification in single-threaded contexts.
Example
public class Main {
public static void main(String[] args) {
StringBuilder sb = new StringBuilder("Hello");
sb.append(" Java");
System.out.println(sb.toString()); // Output: Hello World
}
}
Here, StringBuilder
allows appending strings without creating new objects, making it more memory efficient for frequently modified strings in a non-concurrent scenario.
StringBuffer
Mutable and thread-safe, suitable for strings modified by multiple threads.
Example
public class Main {
public static void main(String[] args) {
StringBuffer sbf = new StringBuffer("Hello");
sbf.append(" World");
System.out.println(sbf.toString()); // Output: Hello World
}
}
In this example, StringBuffer
is used like StringBuilder
, but it is synchronized to avoid issues in a multi-threaded environment. This synchronization, however, comes at the cost of performance compared to StringBuilder
.
6. Explain the concept of reflection in Java.
Reflection in Java allows programs to inspect and modify behavior of classes, interfaces, fields, and methods during runtime. This can be used for inspecting classes in a package, calling methods at runtime, or manipulating internal properties of classes.
Example
Class<?> clazz = Class.forName("java.util.ArrayList");
7. Explain the concept of a design pattern. Give examples of commonly used design patterns in Java.
A design pattern is a general, reusable solution to a commonly occurring problem within a given context in software design. It is not a finished design that can be transformed directly into code; rather, it is a description or template for how to solve a problem that can be used in many different situations. Design patterns are best practices given a name, making them easy to communicate and understand among developers.
Examples:
- Singleton Pattern: Ensures a class has only one instance.
- Observer Pattern: Allows a class to observe and react to changes in another class.
- Factory Pattern: Creates objects without exposing the creation logic.
8. Explain the concept of inner classes in Java. What are the different types of inner classes?
Inner classes are defined within another class.
Types of inner class:
- Member Inner Class: Non-static and can access the member variables of the outer class.
- Static Inner Class: Static and cannot access instance variables of the outer class directly.
- Local Inner Class: Defined within a block, typically inside a method.
- Anonymous Inner Class: Local inner class without a name, often used for implementing interface methods on the fly.
9. Explain the concept of Generics in Java. How does it provide type safety?
Generics in Java allow you to create classes, interfaces, and methods with placeholder types that are specified when they are instantiated or used. This provides type safety by ensuring that you use consistent types throughout your code, reducing runtime errors due to type mismatches.
Example:
List<String> list = new ArrayList<>();
list.add("hello"); // Only strings can be added, ensuring type safety
10. How does Java handle memory leaks? Explain strong and weak references.
Java handles potential memory leaks with its garbage collector, which frees memory used by objects that are no longer reachable. Using strong references, objects are kept in memory as long as their references exist. Weak references allow the garbage collector to reclaim an object’s memory when it is no longer in use, even if its references still exist.
Example
WeakReference<String> weakRef = new WeakReference<>(new String("Hello"));
11. Explain the concept of annotations in Java. How can you create and use custom annotations?
Annotations in Java are used to provide metadata about the code for the compiler and runtime processing. Custom annotations are defined using @interface
, and can specify methods that represent elements of the annotation.
Example
@interface TestInfo {
String createdBy() default "User";
String description();
}
12. Explain the concept of immutable objects in Java. Why are they important?
Immutable objects in Java are objects whose state cannot be changed once they are created. They are important because they are thread-safe and do not require synchronization, making them useful in concurrent applications.
Example
final class ImmutableValue {
private final int value;
public ImmutableValue(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
13. What are the principles of SOLID design in Java? Explain each principle.
SOLID principles help develop software that is easier to maintain and extend:
- Single Responsibility Principle: Each class should have one reason to change.
- Open/Closed Principle: Classes should be open for extension but closed for modification.
- Liskov Substitution Principle: Objects of a superclass should be replaceable with objects of subclasses without affecting the application.
- Interface Segregation Principle: Clients should not be forced to depend on interfaces they do not use.
- Dependency Inversion Principle: High-level modules should not depend on low-level modules but on abstractions.
14. What is the difference between System.out.println() and System.err.println()?
System.out.println() is used for general output, while System.err.println() is used for outputting error messages. Although both output to the console, System.err is typically used to log error messages and can be redirected separately from System.out.
15. What are the different types of class loaders in Java? Explain their roles.
Class loaders in Java load classes into the Java Virtual Machine.
The main types are:
- Bootstrap Class Loader: Loads core Java API classes.
- Extension Class Loader: Loads classes from the extensions directories.
- System Class Loader: Loads classes from the system classpath.
16. Explain the concept of caching in Java. How can you implement caching mechanisms?
Caching in Java involves storing frequently accessed data in a fast access area to speed up operations. Caching can be implemented using various data structures like HashMaps or third-party libraries such as EhCache, which provide advanced features like eviction policies, statistics, and disk overflow.
17. Explain the concept of AOP (Aspect-Oriented Programming) in Java. How can you use AOP frameworks like AspectJ?
Aspect-Oriented Programming (AOP) is a programming paradigm that aims to increase modularity by allowing the separation of cross-cutting concerns. It does so by adding additional behavior to existing code (an advice) without modifying the code itself, instead separately specifying which code is modified via a “pointcut” specification. This means that behaviors that cut across many parts of an application, such as logging, transaction management, or security, can be modularized into distinct aspects rather than spread throughout the code.
Key Concepts in AOP
- Aspect: A modularization of a concern that cuts across multiple classes. It encapsulates behaviors affecting multiple classes into reusable modules.
- Join Point: A point during the execution of a program, such as the execution of a method or the handling of an exception.
- Pointcut: A predicate that matches join points. Pointcuts determine whether a given join point should be advised by an aspect.
- Advice: Action taken by an aspect at a particular join point. Different types of advice include “before”, “after”, and “around” advice.
- Weaving: The process of linking aspects with other application types or objects to create an advised object. This can happen at compile time, load time, or at runtime.
Java Oops
1. What is the difference between method overloading and method overriding?
Method Overloading occurs when two or more methods in the same class have the same name but different parameters (different type, number, or both). It helps in increasing the readability of the program.
Method Overriding occurs when a subclass has a method with the same name and parameters as a method in its superclass. In overriding, the method in the subclass is used instead of the one in the superclass, allowing for dynamic method dispatch and polymorphism.
Example
// Method Overloading
class Display {
void show(int a) {
System.out.println(a);
}
void show(String a) {
System.out.println(a);
}
} //show method overloaded
// Method Overriding
class Animal {
void eat() {
System.out.println("This animal eats food.");
}
}
class Cat extends Animal {
void eat() {
System.out.println("Cat eats fish.");
}
}
//eat() method overridden
2. Explain the concept of Polymorphism in Java.
Polymorphism in Java is the ability of an object to take on many forms. It lets us perform a single action in different ways. Java supports polymorphism through method overriding (runtime polymorphism) and method overloading (compile-time polymorphism). It allows for actions to behave differently based on the actual object that is performing the action.
Example:
class Bird {
void fly() {
System.out.println("Bird flies.");
}
}
class Parrot extends Bird {
void fly() {
System.out.println("Parrot is flying.");
}
}
3. What is the difference between an abstract class and an interface? How have these concepts evolved in Java 8?
Abstract Class
An abstract class can have both concrete (methods with a body) and abstract (methods without a body) methods. It’s used when classes have some methods in common but also have some that require unique implementations per subclass.
Example
abstract class Vehicle {
abstract void go();
}
Interface
Initially, interfaces in Java could only have abstract methods (no method bodies). In Java 8, interfaces were enhanced to support default methods with a body, allowing for some implementation without breaking existing classes that use the interface.
Example
interface Call{
default void makeCall(int number) {
System.out.println("Calling to " + number);
}
}
4. What is the purpose of the this keyword in Java?
The this keyword in Java refers to the current instance of the class. It is commonly used to:
- Differentiate between class fields and parameters with the same names.
- Pass the current object as a parameter to another method.
- Invoke current class methods.
Example:
class Point {
int a, b;
Point(int a, int b) {
this.a = a;
this.b = b;
}
void display() {
System.out.println("Point: (" + this.a + ", " + this.b + ")");
}
}
5. How does Java handle static and dynamic binding? Explain the concept of virtual method invocation.
Static Binding: Also known as early binding, occurs when the method is resolved at compile time. It is used for methods marked as final or private, which cannot be overridden.
Dynamic Binding: Occurs when the method is resolved at runtime. Java uses this to support polymorphism. The method to be executed is determined based on the object’s runtime type.
Virtual Method Invocation: In Java, non-static methods are by default “virtual functions.” This means the method implementation that gets invoked is the one belonging to the runtime type of the object, not the type of the reference.
Example:
class Animal {
void eat() {
System.out.println("Animal eats.");
}
}
class Dog extends Animal {
void eat() {
System.out.println("Dog eats bone.");
}
}
class Test {
public static void main(String[] args) {
Animal myAnimal = new Dog();
myAnimal.eat(); // Dog's eat method is called, demonstrating virtual method invocation
}
}
Java Collection Interview Questions
1. Tell me something about HashMap in Java.
HashMap is a Collection class in Java whose implementation is based on the hash table data structure. HashMap class extends AbstractMap class and AbstractMap class implements the Map interface.
Some Important points about Hashmap:
- HashMap allows only one null key and multiple null values.
- HashMap does not maintain the order of insertion into the map.
- The initial size of the hashmap bucket is 16 which grows to 32 when the map entries cross 75%.
- HashMap uses hashCode() and equals() methods on keys to perform put and get operations. It internally checks whether the key already exists or not.
- Hashmap is not a thread-safe. This is the reason it won’t use in a multithreaded environment.
2. What are load Factor, Threshold, Rehashing, and collision in hashmap?
Load factor is the measure to decide when to increase the size of the Map. By default, the load factor is 75% of the capacity.
The threshold can be calculated by multiplying the current capacity and load factor.
Rehashing means, re-calculating the hash code of already stored entries. When entries in the hash table exceed the threshold, the Map is rehashed and increases the size of buckets.
Collision is a condition when a hash function returns the same bucket location for two different keys.
For example:
Suppose Our HashMap has an initial capacity is 16 and a load factor is 0.75.
Threshold will be 16 * 0.75 = 12,
It means after the 12th entry in the hashmap, the capacity of the hashmap will increase from 16 to 32. Its getting just double.
3. How does HashMap Internally work in Java?
A HashMap
in Java is a data structure that stores date in key-value pairs. It is useful for the fast retrieval and insertion of values based on their keys. It internally uses an array of buckets to store these key-value entries. In hashmap one key can have only one hash output. Two key can also result same output but one key can’t result two output.
General steps for how a HashMap works internally?
Hashing: When you insert a key-value pair into a HashMap
, the hash code of the key is computed using the hashCode()
method of the key object. This hash code is used to determine the index or bucket in the array where the entry will be stored.
Example
for Large Key :- hashcode(key) = key%m
For String key :- Weighted sum
Suppose key is “cat” then hashcode() will be (s[0]*x^0 + s[1]*x^1 + s[2]*x^2)%m
Indexing
The computed hash code is then used to find the index within the array of buckets. The index is typically obtained by performing a modulo operation on the hash code to ensure it fits within the array size.
Suppose if we have a hashtable of size 7 then
hashcode(key) = key%7
Collisions
Collisions occur when two or more keys produce the same hash code and hence map to the same bucket. To handle collisions, Java’s HashMap
uses a concept called chaining. Each bucket contains a linked list (or a balanced tree in later Java 8 versions) of key-value pairs that have the same hash code.
Insertion and Retrieval
When you insert a key-value pair, the HashMap
checks if there is already an entry in the bucket corresponding to the computed index or not. If there is not entry then add a new entry to the bucket. If collision occurs, the newly given entry will be added to the existing linked list (or tree) within the bucket. When you retrieve a value by its key, the HashMap
uses the hash code to find the correct bucket. If bucket found then it searches through the linked list (or tree) within that bucket to locate the desired key-value pair.
Resizing
To maintain efficiency, the HashMap
dynamically resizes its array of buckets when the number of entries crosses a certain threshold. This helps prevent too many collisions and ensures that the average number of entries per bucket remains relatively low.
Example of Hashmap
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
HashMap<String, Integer> hashMap = new HashMap<>();
hashMap.put("java", 1);
hashMap.put("python", 2);
hashMap.put("html", 3);
int javaValue = hashMap.get("java");
System.out.println("Value of 'java': " + javaValue);
boolean containsKey = hashMap.containsKey("python");
System.out.println("Contains 'python': " + containsKey);
hashMap.remove("html");
System.out.println("Size of HashMap: " + hashMap.size());
}
}
Output
Value of 'java': 1
Contains 'python': true
Size of HashMap: 2
4. Explain how hashcode will be calculated for String Key.
Hashmap has hashCode() method. This method is used to calculate the hashcode value of the String as an Integer.
Syntax of hascode:
public int hashCode()
Program
import java.io.*;
class Main {
public static void main(String[] args)
{
String str = "Name";
System.out.println(str);
int hashCode = str.hashCode();
System.out.println(hashCode);
}
}
Output
Name
2420395
Here we got Output 2420395 for String “Name”. Below is the way to calculate the hashcode of the string.
How hashcode is calculated for string key?
Below is the formula to calculate the hashcode value of a String:
s[0]*31^(n-1) + s[1]*31^(n-2) + … + s[n-1]
Here:
- s[i] is the ith character of the string
- ^ is a the exponential operator
- n shows the length of the string
5. What is the Difference between HashMap and LinkedHashMap?
- The difference between HashMap and LinkedHashMap is that LinkedHashMap maintains the insertion order of keys but HashMap doesn’t maintain the insertion order of keys.
- LinkedHashMap requires more memory than HashMap to keep the record of the insertion order. Basically, LinkedHashMap uses doubly LinkedList to keep track of the insertion order of keys.
- LinkedHashMap extends HashMap and implements the Map interface and Hashmap extends AbstractMap class and implements the Map interface.
- Hashmap was introduced in JDK 2.0 but LinkedHashMap was introduced in JDK 4.0.
Here is the Program of HashMap and LinkedHashMap.
Program on Hashmap
import java.util.HashMap;
public class Main {
public static void main(String[] args)
{
HashMap<String,String> map = new HashMap<>();
map.put("firstName","Interview");
map.put("lastName","Expert");
map.put("rollNo","1");
System.out.println(map.size());
if(map.containsKey("firstName")){
System.out.println(map.get("firstName"));
}
if(Integer.parseInt(map.get("rollNo"))<20){
System.out.println(map.get("lastName"));
}
}
}
Output:
3
Interview
Expert
Program Of LinkedHashMap
import java.util.LinkedHashMap;
public class Main {
public static void main(String[] args)
{
LinkedHashMap<String,String> map = new LinkedHashMap<>();
map.put("firstName","Interview");
map.put("lastName","Expert");
map.put("rollNo","1");
System.out.println(map.size());
if(map.containsKey("firstName")){
System.out.println(map.get("firstName"));
}
if(Integer.parseInt(map.get("rollNo"))<10){
System.out.println(map.get("lastName"));
}
}
}
Output:
3
Interview
Expert
6. What is Concurrent HashMap?
ConcurrentHashMap is a class of Collection Framework that provides a concurrent version of HashMap. It is a thread-safe i.e. multiple threads can access the single object of the map.
At a time any number of threads can perform the read operations without locking. But for write/update operation at a single time thread must lock the particular segment in which the thread wants to operate.
Functionality wise HashMap and ConcurrentHashMap are the same, except ConcurrentHashMap maintains concurrency. Hashmap Can have a null key and value but concurrentHashMap Cannot have null key and value pair.
ConcurrentHashMap uses a Hashtable data structure.
ConcurrentHashMap Example
import java.util.concurrent.ConcurrentHashMap;
import java.util.Map;
public class Main {
public static void main(String[] args)
{
Map<String,String> chm = new ConcurrentHashMap<>();
chm.put(null,null);
}
}
Output
Exception in thread "main" java.lang.NullPointerException
at java.base/java.util.concurrent.ConcurrentHashMap.putVal(ConcurrentHashMap.java:1011)
at java.base/java.util.concurrent.ConcurrentHashMap.put(ConcurrentHashMap.java:1006)
at Main.main(Main.java:7)
Here we will get a NullPointerException because we are trying to insert a null key value in the concurrent hashmap which is not allowed.
7. What is the Time Complexity of HashMap?
Usually, Time complexity of the Hashmap is O(1). But in some cases, it might change. Let’s assume a scenario in the hashmap when the hashcode will return the same index value for each entries. In that case at a single index of the hashtable, a singly linked list will create to store the key-value pair of the hashmap.
This is a worst-case scenario in HashMap which will be O(n) complexity to lookup. Here we have to walk through all entries in the same hash bucket. This situation is very rare.
There are some improvements done in Java 8 to improve the time complexity from O(n) to O(log n). Instead of using singly linked list, Hashmap in Java 8 uses self-balancing tree like RB tree and AVL tree.
8. Tell me some differences between ArrayList and LinkedList.
- ArrayList uses a dynamic array/Array object data structure to store the elements but LinkedList internally uses a doubly linked list to store the elements.
- ArrayList Manipulation is slow because it uses an array and if we remove any element from the array we have to shift other elements in memory. But LinkedList Manipulation is faster because it uses a doubly linked list so no shift is required when we remove any element.
- ArrayList is better for storing and accessing elements but LinkedList is better for manipulating elements.
- ArrayList takes memory in a contiguous fashion but the linked list does not takes memory in a contiguous.
9. When you will prefer ArrayList and when LinkedList in your RealTime projects?
As We know Accessing an element in ArrayList takes constant time [O(1)] and adding an element takes O(n) in the worst case. And in LinkedList inserting an element takes O(n) time and accessing also takes O(n) time but LinkedList needs some more memory than ArrayList.
Accessing elements in ArrayList is faster because it is index based. But Accessing elements in LinkedList is slower because we need to traverse each node. Insertion and deletion in Linked List are much faster because here we need to change the pointer of the node only. We need not shift the element as we do in ArrayList.
So here we are at the conclusion, if we need to perform more manipulation work on the element and memory is not a problem then we can prefer Linked List over ArrayList.
But When we require more searching of the element then we can choose ArrayList over LinkedList.
Time complexity with operations
For ArrayList<E>
- get(int index) takes O(1).
- add(E element) operation takes O(1) amortized, but in the worst case it will be O(n) since the array must be resized and copied.
- add(int index, E element) takes O(n) Complexity (with n/2 steps on average).
- remove(int index) operation takes O(n) (with n/2 steps on average).
- Iterator.remove() have O(n) Complexity (with n/2 steps on average).
- ListIterator.add(E element) operation takes O(n) (with n/2 steps on average)
For LinkedList<E>
- get(int index) operation takes O(n) , It will take O(1) when index = 0 or index = list.size() -1 .
- add(int index, E element) take O(n) and It will take O(1) when index = 0 or index = list.size() – 1
- remove(int index) takes O(n) and It will take O(1) when index = 0 or index = list.size() – 1.
10. What is the difference between HashSet and LinkedHashSet?
Ans:
- HashSet uses Hashtable to store the elements. LinkedHashSet uses HashTable and a doubly linked list to store and maintain the insertion order of the elements.
- HashSet extends AbstractSet class but LinkedHashSet extends HashSet.
- HashSet won’t maintain the insertion order of the element but LinkedHashSet maintains the insertion order of an element.
- HashSet takes less memory in comparison to LinkedHashSet because HashSet won’t maintain the insertion order but LinkedHashSet maintains the insertion order.
- HashSet provides faster performance than LinkedHashSet.
11. Differences between HashMap and HashSet.
Ans:
- Hashmap is the implementation of the Map interface But HashSet is the implementation of the Set interface.
- HashSet internally uses Hashmap for its implementation But HashMap Won’t.
- HashMap stores value in key-value pair But HashSet Stores only object no key-value pair.
- HashMap uses the put(k,v) method to add elements to the map whereas HashSet uses add(K) method.
- HashMap Allows duplicate values not key But HashSet won’t allow duplicate values.
- HashMap allows a single null key and multiple null values but HashSet allow only one null value.
12. What is the purpose of the equals() and hashCode() methods? Why should they be overridden together?
In Java, the equals() method is used to determine if two objects are equal based on their content, not their reference. The hashCode() method provides a way of computing a small integer signature from an object’s data that helps in efficient lookup in collections like HashMap.
When you override equals(), you should also override hashCode() because objects considered equal (by equals()
) must produce the same hash code. If they don’t, it can cause problems when using hash-based collections like HashMap
or HashSet
.
Example
class Student {
String name;
int age;
// Constructor
public Student(String name, int age) {
this.name = name;
this.age = age;
}
// Override equals method
@Override
public boolean equals(Object obj) {
if (this == obj) return true; // Check for reference equality
if (obj == null || getClass() != obj.getClass()) return false; // Check for null and ensure exact class match
Student student = (Student) obj; // Cast the object to Student
return age == student.age && Objects.equals(name, student.name); // Check if values are equal
}
// Override hashCode method
@Override
public int hashCode() {
return Objects.hash(name, age); // Calculate hash code based on name and age
}
}
Why Override Both?
It’s crucial to override both equals()
and hashCode()
because failing to do so can lead to inconsistent behavior when your objects are placed in any hash-based collection, such as HashMap
or HashSet
. If two objects are considered equal by the equals()
method but generate different hash codes, they might be mistakenly added as separate entries in a HashSet
, which contradicts the set’s properties.
13. What is the difference between a shallow copy and a deep copy?
A shallow copy of an object copies the values of the object’s properties. However, if the property values are references to other objects, the references are copied but not the objects they point to.
Conversely, a deep copy copies all fields, and if the fields contain references to other objects, it copies those objects as well. A deep copy is fully independent of the original object.
Example
class Rectangle {
Point origin;
int width;
int height;
// Shallow copy constructor
Rectangle(Rectangle other) {
this.origin = other.origin; // copies the reference
this.width = other.width;
this.height = other.height;
}
// Deep copy method
Rectangle deepCopy() {
Rectangle newRect = new Rectangle();
newRect.origin = new Point(this.origin.x, this.origin.y); // copies the object
newRect.width = this.width;
newRect.height = this.height;
return newRect;
}
}
14. What is the difference between a HashSet and a TreeSet?
A HashSet stores its elements in a hash table, which means it uses the hashCode()
method to retrieve its elements efficiently. Elements in a HashSet are unordered, and it provides faster operations like add, remove, and contains.
A TreeSet stores its elements in a red-black tree, maintaining them in sorted order according to their natural ordering or a specified comparator. TreeSet offers several methods to deal with the sorted nature of its elements, such as first()
, last()
, headSet()
, and tailSet()
.
Example
#HashSet Example
Set<Integer> hashSet = new HashSet<>();
hashSet.add(2);
hashSet.add(3);
hashSet.add(1);
System.out.println(hashSet); // Outputs [1, 2, 3] it could be in any order
#TreeSet Example
Set<Integer> treeSet = new TreeSet<>();
treeSet.add(2);
treeSet.add(3);
treeSet.add(1);
System.out.println(treeSet); // Outputs [1, 2, 3] in sorted order
15. How does Java handle concurrent modification exceptions? Explain the use of iterators and ConcurrentModificationException.
A ConcurrentModificationException is thrown when a collection is modified while iterating over it, except through its own iterator’s remove method. To safely modify a collection during iteration, use the iterator’s own methods for modifications.
Example 1: It will give ConcurrentModificationException
import java.util.*;
class Main {
public static void main(String[] args) {
List<String> list = new ArrayList<>(Arrays.asList("a", "b", "c", "d"));
Iterator<String> it = list.iterator();
while (it.hasNext()) {
String item = it.next();
System.out.println(item);
if (item.equals("b")) {
// Trying to modify the collection directly while iterating over it
list.remove(item); // This line will throw ConcurrentModificationException
}
}
System.out.println(list);
}
}
Output
a b Exception in thread "main" java.util.ConcurrentModificationException at java.base/java.util.ArrayList$Itr.checkForComodification(ArrayList.java:1042) at java.base/java.util.ArrayList$Itr.next(ArrayList.java:996) at Main.main(Main.java:7)
Example 2: It will not give ConcurrentModificationException
import java.util.*;
class Main {
public static void main(String[] args) {
List<String> list = new ArrayList<>(Arrays.asList("a", "b", "c", "d"));
Iterator<String> it = list.iterator();
while (it.hasNext()) {
String value = it.next();
if ("c".equals(value)) {
it.remove();
}
}
System.out.println(list);
}
}
Output
[a, b, d]
16. What is the difference between HashMap and HashTable?
HashMap is a part of Java’s collection since Java 1.2. It allows storing key-value pairs in hash tables. HashMap is not synchronized, which means it is not safe for multithreaded use without external synchronization. It allows one null key and multiple null values.
HashTable is a legacy class similar to HashMap, but it is synchronized, which makes it thread-safe for concurrent access without additional synchronization. However, its synchronized nature means it’s generally slower than HashMap. HashTable does not allow null keys or null values.
Example
Map<String, String> hashMap = new HashMap<>();
hashMap.put(null, "value"); // allowed
Map<String, String> hashTable = new Hashtable<>();
hashTable.put(null, "value"); // It will Throws NullPointerException
Multi-threading Questions
1). What are the Different ways to create threads in Java?
There are two ways to create threads in Java:
- By extending the Thread class
- By implementing a Runnable interface.
1). By Extending the Thread Class
class Main extends Thread{
public void run(){
System.out.println("thread is running");
}
public static void main(String args[]){
Main thread1 = new Main();
thread1.start();
}
}
Output:
Thread is running
2). By implementing a Runnable interface
class Main implements Runnable{
public void run(){
System.out.println("thread is running");
}
public static void main(String args[]){
Main obj=new Main();
// Here we are Using the constructor Thread(Runnable r)
Thread tobj =new Thread(obj);
tobj.start();
}
}
Output:
Thread is running
2. Difference between Thread and Runnable.
- Thread is a class used to create a thread Whereas Runnable is an interface that is used to create a thread.
- Thread has methods like start() and run() but Runnable has only one method run().
- Thread won’t allow multiple inheritance but Runnable allows multiple inheritance.
- Thread requires more memory but Runnable requires less memory.
- In Thread Each thread creates a unique object but in Runnable same object is shared with multiple threads.
3. Tell some Differences between Callable & Runnable in Java.
- Callable belongs to java.util.concurrent package whereas Runnable belongs to java.lang package.
- Thread will not create by passing a callable as a parameter but Runnable will create a thread by passing a runnable as a parameter.
- Callable has call() method whereas Runnable has the run() method.
- Callable might return some output but Runnable does not return anything.
- By invoking invokeAll() method bulk execution of the task is possible in callable but in runnable we can’t use it for bulk execution of the task.
4. What is the difference between sleep() & wait() methods in Java.
- Sleep() method belongs to the Thread class whereas Wait() method belongs to the Object class.
- Sleep() method is used to pause the execution of the current thread for a given time in Milliseconds. But the wait() method tells the thread to wait until another thread invoke’s the notify() or notifyAll() method for this object.
- Sleep() method will not release the lock on the object during Synchronization but Wait() method can release the lock on the object during Synchronization.
- Sleep() is a static method but wait() is not a static method.
- Sleep() has two overloaded methods sleep(long millis) and sleep(long millis, int nanos), Whereas Wait() has Three Overloaded methods wait(), wait(long timeout), wait(long timeout, int nanos).
Example of Sleep method
import java.lang.Thread;
class Main {
public static void main(String[] args)
{
try {
for (int i = 1; i <=10; i++) {
Thread.sleep(1000);
System.out.println(i);
}
}
catch (Exception e) {
System.out.println(e);
}
}
}
Output
1
2
3
4
5
6
7
8
9
10
5. What is the difference between the final, finally, and finalize keywords in Java?
final: The final keyword can be used with classes, methods, or variables. When used with a variable, it means the variable cannot be changed once initialized. When used with a method, it prevents the method from being overridden in a subclass. When used with a class, it prevents the class from being subclassed.
Example:
final int NUMBER = 9;
// NUMBER = 10; // This would cause a compiler error because NUMBER is final
finally: The finally block is part of Java’s exception handling. It always executes when the try block exits, regardless of whether an exception was thrown or caught. It’s typically used to close or release resources like files or databases.
Example:
try {
// code that might throw an exception
} catch (Exception e) {
// handle exception
} finally {
// code that will always run
}
finalize: The finalize method is called by the garbage collector on an object when garbage collection determines that there are no more references to the object. It’s generally used to clean up resources before the object is destroyed, though its use is discouraged in favor of other resource-management techniques.
Example:
protected void finalize() {
// cleanup code before garbage collection
}
6. Explain Synchronization mechanisms, thread pools, and the ForkJoinPool framework.
Synchronization mechanisms
These are tools in Java that control the access of multiple threads to a common resource. The synchronized keyword can be used to prevent more than one thread from executing a method or block at the same time.
Example:
public synchronized void accessResource() {
// only one thread can execute this at a time
}
Thread pools
A thread pool is a group of pre-instantiated, idle threads which stand ready to be given work. This prevents the overhead of creating a new thread for every task. The Java ExecutorService is a higher-level replacement for working directly with threads and provides methods for managing termination and methods that can produce a Future
for tracking progress of one or more asynchronous tasks.
Example:
ExecutorService executor = Executors.newFixedThreadPool(3);
executor.submit(() -> {
System.out.println("Running in a thread pool!");
});
executor.shutdown();
ForkJoinPool framework
This is an implementation of ExecutorService that helps leverage multi-processor capabilities by dividing (forking) tasks into smaller parts, processing them in parallel, and then combining (joining) the results.
Example:
ForkJoinPool forkJoinPool = new ForkJoinPool();
forkJoinPool.submit(() -> {
// perform some parallel computation
});
forkJoinPool.shutdown();
7. What is the purpose of the volatile keyword in Java?
The volatile keyword in Java is used to indicate that a variable’s value will be modified by different threads. Declaring a variable volatile ensures that its value is read from and written to main memory directly. This prevents threads from caching the variable’s value and ensures that all reads and writes are done in a thread-safe manner.
Example:
volatile boolean running = true;
public void stopRunning() {
running = false; // Changes made here will be visible to other threads immediately
}
8. Explain the concept of Serialization and Deserialization in Java.
Serialization is the process of converting an object’s state to a byte stream, thus making it easy to save it to a file, send it over a network, or store in a database. Deserialization is the reverse process where the byte stream is converted back into a replica of the original object.
Example:
import java.io.*;
public class SerializeExample {
public static void serialize(Object obj, String filename) throws IOException {
FileOutputStream fileOut = new FileOutputStream(filename);
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(obj);
out.close();
fileOut.close();
}
public static Object deserialize(String filename) throws IOException, ClassNotFoundException {
FileInputStream fileIn = new FileInputStream(filename);
ObjectInputStream in = new ObjectInputStream(fileIn);
Object obj = in.readObject();
in.close();
fileIn.close();
return obj;
}
}
9. What is the purpose of the ThreadLocal class in Java?
The ThreadLocal class in Java provides thread-local variables. Each thread that accesses one of these variables via its get or set method has its own, independently initialized copy of the variable. ThreadLocal instances are typically private static fields in classes that wish to associate state with a thread.
Exceptional Handling in Java
1. What is Exceptional Handling in Java?
In Java, Exception Handling is a mechanism to handle runtime errors and protect the program from abnormal termination. It helps to maintain the normal flow of the application in case any exceptions come.
There are multiple types of Exceptions such as ClassNotFoundException, IOException, and SQLException.
java.lang.Throwable class is the root class of the Java Exception hierarchy. It is inherited by two subclasses Exception and Error. The hierarchy of Java Exception classes is given below:
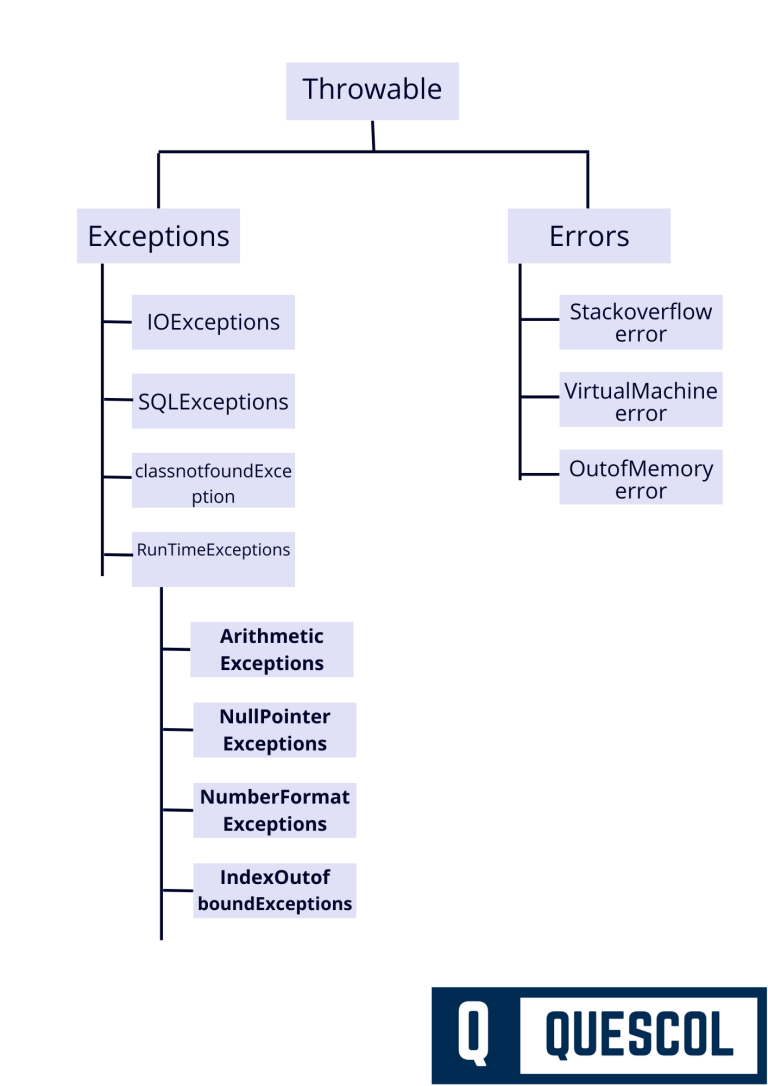
2. Difference between Checked and Unchecked Exceptions.
- Checked exception occurs at compile time whereas the Unchecked exception occurs at run time.
- Checked Exception is checked by the compiler but the Unchecked exception is not.
- Checked Exception can be handled at compile time but Unchecked Exception can be handled at runtime.
- Checked Exception is a sub-class of the Exception class but Unchecked Exception is not a part of the Exception class.
- Some Checked Exceptions are ClassNotFoundException, IOException, SQLException, etc. Some Unchecked Exceptions are ArithmeticException, NullPointerException, ArrayIndexOutOfBoundExceptions.
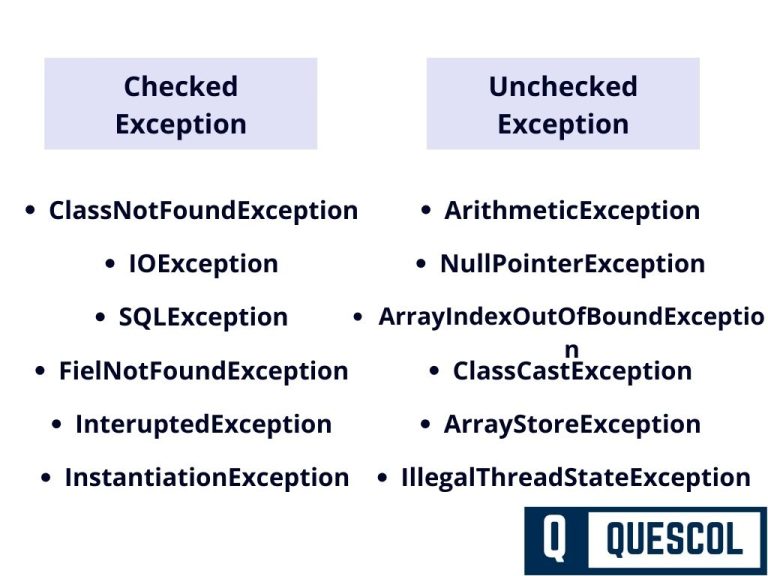
3). What is the difference between an Error and an Exception?
Aspect | Error | Exception |
---|---|---|
Definition | An Error indicates serious problems that a reasonable application should not try to catch. | An Exception is an event that disrupts the normal flow of the program and can be caught and handled by the application. |
Recoverability | Errors are typically not recoverable as they are often severe and indicate problems with the system itself, such as system crashes or resource exhaustion. | Exceptions are often recoverable using try-catch blocks, allowing the application to continue running. |
Control Flow | When an error occurs, it is usually beyond the application’s control to handle it effectively (e.g., OutOfMemoryError , StackOverflowError ). | Exceptions can often be controlled or anticipated in the flow of an application (e.g., FileNotFoundException , ParseException ). |
Type of Issue | Errors are typically external to the application and reflect underlying problems with the environment in which the application is running. | Exceptions are generally conditions that the application itself might anticipate and recover from (e.g., invalid user input, file not found). |
Examples | OutOfMemoryError, NoClassDefFoundError, StackOverflowError | IOException, SQLException, NullPointerException, ArithmeticException |
Hierarchy | Error class extends the Throwable class directly. | Exception class also extends the Throwable class but includes specific subcategories like RuntimeException (unchecked) and checked exceptions. |
public class ErrorExample {
public static void main(String[] args) {
try {
throw new StackOverflowError("Example StackOverflowError");
} catch (Error e) {
System.err.println("Caught Error: " + e.getMessage());
// Attempt to log error details, perform cleanup, or restart components if appropriate
}
}
}
4. How many ways you can print exceptions in Java?
There are multiple ways to print the exceptions in Java. Here we will see 3 methods to print the exceptions.
1). printStackTrace()
2). toString()
3). getMessage()
1). printStackTrace()
printStackTrace method prints exception information printStackTrace() is a method of Java.lang.Throwable class. It is used to print the details of exceptions like class name and line number where the exception occurred.
Program to demonstrate printStackTrace() method
import java.io.*;
class Main {
public static void main (String[] args) {
int a=5, b=0;
try{
System.out.println(a/b);
}
catch(ArithmeticException e){
e.printStackTrace();
}
}
}
Output:
java.lang.ArithmeticException: / by zero at Main.main(Main.java:6)
2). toString()
toString() method is also used to print exceptions. It prints exception information in the format of Name of the exception along with a description of the exception.
Program to demonstrate toString() method
import java.io.*;
class Main {
public static void main (String[] args) {
int a=5, b=0;
try{
System.out.println(a/b);
}
catch(ArithmeticException e){
System.out.println(e.toString());
}
}
}
Output:
java.lang.ArithmeticException: / by zero
3). getMessage()
getMessage() method prints only the description of the exception message.
Program to demonstrate getMessage() method
import java.io.*;
class Main {
public static void main (String[] args) {
int a=5, b=0;
try{
System.out.println(a/b);
}
catch(ArithmeticException e){
System.out.println(e.getMessage());
}
}
}
Output:
/ by zero
5. What is the process to create custom Exception in Java?
Here we are showing two examples to show how you can create your own custom exception class in Java.
Program 1: Create Custom Exception
class CustomException extends Exception{
public CustomException(String ex){
super(ex);
}
}
public class Main{
public static void main(String ...a){
int age = 13;
try{
if(age<18){
throw new CustomException("no valid Age");
}else{
System.out.println("Age is valid");
}
}
catch(CustomException e){
System.out.println("Exception occured: " + e);
}
System.out.println("Rest Code is running");
}
}
Output
Exception occured: CustomException: no valid Age
Rest Code is running
Program 2: Create Custom Exception
class CustomException extends Exception {
String message;
CustomException(String str) {
message = str;
}
public String toString() {
return ("Custom Exception Occurred : " + message);
}
}
public class Main {
public static void main(String args[]) {
try {
throw new CustomException("This is CustomException");
} catch(CustomException e) {
System.out.println(e);
}
}
}
Output
Custom Exception Occurred : This is CustomException
6. Write the difference between Throw and Throws.
Throw vs Throws
- throw keyword is used inside the function or the block of code to throw an exception explicitly when an exception occurs. Whereas throws keyword is used with method signature with exception means exception might be thrown by the function during the execution if an exception occurs.
- throw keyword is followed by an instance of Exception that will be thrown whereas throws keyword is followed by class names of Exceptions to be thrown.
- throw keyword can be used to throw only one exception at a time but in throws keywords, we can use multiple Exception classes separated by a comma.
- throw keyword is used to propagate unchecked Exceptions whereas the throws keyword is used to propagate checked Exceptions.
Program of using throw keyword
public class Main{
public void checkAge(int age){
if(age<18)
throw new ArithmeticException("Age is not valid for voting");
else
System.out.println("Age is valid for voting");
}
public static void main(String args[]){
Main obj = new Main();
obj.checkAge(10);
System.out.println("Execution continue");
}
}
Output
Exception in thread "main" java.lang.ArithmeticException: Age is not valid for voting
at Main.checkAge(Main.java:4)
at Main.main(Main.java:10)
Program of using throws keywords
public class Main{
public int division(int a, int b) throws ArithmeticException{
int result = a/b;
return result;
}
public static void main(String args[]){
Main obj = new Main();
try{
System.out.println(obj.division(15,0));
}
catch(ArithmeticException e){
System.out.println("We can't divide number by zero");
}
}
}
Output
We can't divide number by zero
7. What is Serialization and why do we use it?
Serialization is used to convert the object into a byte stream so that it can be sent over the network. Deserialization is the reverse process where the byte stream is converted into an actual Java object.
Serialization is required because Network infrastructure and Hard disk or any other hardware components can understand bits and bytes only but not the JAVA objects.
So we use Serialization as a translation to translate our Java object’s values/states to bytes to send it over the network or save it.
SerialVersionUID is used here for identity purposes. It must be static, final, and of type long.
Java Program for Serialization and Deserialization
import java.io.*;
public class Main {
public static void main(String[] args) {
Student student = new Student("quescol", 2);
byte[] bytes = convertObjectToBytes(student);
System.out.println(bytes);
Student s = (Student) convertBytesToObject(bytes);
System.out.println(s);
}
public static byte[] convertObjectToBytes(Object obj) {
ByteArrayOutputStream boas = new ByteArrayOutputStream();
try (ObjectOutputStream ois = new ObjectOutputStream(boas)) {
ois.writeObject(obj);
return boas.toByteArray();
} catch (IOException ioe) {
ioe.printStackTrace();
}
throw new RuntimeException();
}
public static Object convertBytesToObject(byte[] bytes) {
InputStream is = new ByteArrayInputStream(bytes);
try (ObjectInputStream ois = new ObjectInputStream(is)) {
return ois.readObject();
} catch (IOException | ClassNotFoundException ioe) {
ioe.printStackTrace();
}
throw new RuntimeException();
}
}
class Student implements Serializable {
private String name;
private int age;
public Student( String name, int id) {
this.age = age;
this.name = name;
}
}
Output
[B@7d4793a8
Student@721e0f4f
8. What is the volatile and Transient keyword in Java?
- A volatile keyword is used in a multithreading environment. When two threads are performing reading and writing operations on the same variable, a volatile keyword is used. It flushes the changes directly to the main memory instead of the CPU cache. A transient keyword is used in serialization. Variable where transient is used, cannot be part of the serialization and deserialization.
- Volatile variable is not initialized with any default value whereas transient variables are initialized with a default value during deserialization.
- Volatile variable can be used with the final keyword whereas Transient cannot be used with the final keyword.
- Volatile variable can be used with static keyword whereas Transient cannot be used with the static keyword.
9. How you will create an Immutable class in Java?
In Java, an Immutable class means once the object is created in the class, its fields cannot be changed or modified.
All the wrapper classes like Boolean, Byte, Long, Short, Integer, Float, Double, Char, and String are immutable.
Steps to create an immutable class in Java
- First Declare the class with the final keyword so it can’t be extended.
- Make all the classes private. It will not allow direct access.
- Don’t make any setter methods for variables.
- Make all mutable fields final so that their value can be assigned only once.
- Initialize all fields value using the constructor.
Program of Immutable Java Class
final class Student{
private String name;
private int roll;
Student(String name, int roll) {
this.name = name;
this.roll = roll;
}
public String getName() {
return name;
}
public int getRoll() {
return roll;
}
}
class Main {
public static void main(String[] args) {
Student obj = new Student("quescol", 1);
System.out.println("Name: " + obj.getName());
System.out.println("Roll: " + obj.getRoll());
}
}
Output
Name: quescol
Roll: 1
10. How you will Create a Singleton class in Java.
Singleton class is a class in oops that can have only one object at a time and can be globally accessible.
It Saves memory because Only a single instance is created and reused again and again.
Single Class is mostly used in the multi-threaded system, database application, logging, caching, configuration settings, etc.
There are two forms of singleton design pattern
Early Instantiation: In this Singleton design pattern object will create at the load time.
Lazy Instantiation: In this Singleton design pattern object will create according to the requirement.
Early Instantiation Program
class Singlton {
//Instance will be created at load time
private static Singlton obj=new Singlton();
private Singlton(){}
public static Singlton getSinglton(){
return obj;
}
}
class Main {
public static void main(String[] args) {
Singlton s1;
s1= Singlton.getSinglton();
Singlton s2;
s2= Singlton.getSinglton();
System.out.println(s1);
System.out.println(s2);
}
}
Output
Singlton@1e81f4dc
Singlton@1e81f4dc
Lazy Instantiation example
class Singlton {
private static Singlton singlton;
private Singlton(){
}
public static Singlton getInstance() {
if(null == singlton){
singlton = new Singlton();
}
return singlton;
}
}
class Main {
public static void main(String[] args) {
Singlton s1;
s1= Singlton.getInstance();
Singlton s2;
s2= Singlton.getInstance();
System.out.println(s1);
System.out.println(s2);
}
}
Output
Singlton@3b22cdd0
Singlton@3b22cdd0
Java 8 Questions
1. Explain the concept of lambda expressions in Java 8.
Lambda expression is a function without a name. It is also known as an anonymous function as it does not have type information by itself. Lambda expression is executed on-demand. It is used with collection to perform operations like iteration, filter, extracting data, etc. The return type of the anonymous function is the same as that of the body expression.
Lambda expressions have a compact syntax, consisting of parameter list, an arrow (->
), and a body. The body can be an expression or a block of statements.
Syntax of lambda expressions
(parameters) -> expression
(parameters) -> { statements }
2. Explain the concept of anonymous classes in Java.
Anonymous classes in Java are a form of inner class without a named identifier. They are typically used for instantiating simple objects needed for a short duration, often to implement an interface or extend a class without creating a separate, named class.
The syntax of an anonymous class
new superclass_or_interface() {
// class body
};
3. What is the difference between the Comparable and Comparator interfaces?
In Java, both the Comparable and Comparator interfaces are used to define the order of objects, but they serve different purposes and are used in different contexts.
Comparable Interface
- Purpose: The
Comparable
interface is used to define the default natural ordering of objects of a particular class. - Method: It requires the implementation of a single method, compareTo(Object obj).
Comparable Example
public class Employee implements Comparable<Employee> {
private int id;
public int compareTo(Employee other) {
return Integer.compare(this.id, other.id);
}
}
Comparator Interface
- Purpose: The
Comparator
interface is used to define an ordering, but not the natural ordering. It allows the definition of multiple different orderings for a class. - Method: It requires the implementation of the
compare(Object obj1, Object obj2)
method.
Comparator Example
public class AgeComparator implements Comparator<Employee> {
public int compare(Employee e1, Employee e2) {
return Integer.compare(e1.getAge(), e2.getAge());
}
}
4. Explain the concept of method references in Java 8.
Method References are a shorthand notation of Lambda Expressions to call methods directly. They are used to make the code cleaner and more readable when a lambda expression does nothing but call an existing method.
Example
List<String> names = Arrays.asList("Som", "Vinay", "Charlie");
names.forEach(System.out::println); // Method reference
5. What is the purpose of the default keyword in Java interfaces?
The default keyword in Java interfaces allows you to define methods with a body in interfaces. Before Java 8, interfaces could only have method signatures without bodies. The default method can be executed if the classes that implement the interface do not provide their own implementation.
Example
interface Display{
default void show() {
System.out.println("Show method calling");
}
}
6. Explain the concept of functional interfaces in Java 8. Give examples.
A functional interface in Java 8 is an interface that contains exactly one abstract method. They are intended to be used primarily with lambda expressions. Java 8 includes many built-in functional interfaces like Runnable, Callable, and others in the java.util.function package.
Example
Functional interface
@FunctionalInterface
public interface Operation{
// An abstract method that takes an integer and returns an integer.
int operation(int x);
}
TestSimpleFunction.java
public class TestSimpleFunction {
public static void main(String[] args) {
// Create an instance of Operation using a lambda expression.
Operations cube = x -> x * x * x;
// Using the functional interface to perform an operation.
int result = cube.operation(5);
System.out.println("Cube of 5 is: " + result);
}
}
7. Explain the concept of CompletableFuture.
The CompletableFuture class in Java is part of the java.util.concurrent package, introduced in Java 8 as an enhancement to its Future API. It implements the Future and CompletionStage interfaces and offers a rich, flexible, and asynchronous programming framework.
CompletableFuture is designed to represent a future result of an asynchronous computation. This means it acts as a placeholder for the result, which will become available at a future point in time.
CompletableFuture provides methods for composing and chaining multiple stages of asynchronous tasks. This allows you to perform further operations once the initial computation is done, or even combine multiple asynchronous computations
Here is an interview clearing guide to help you prepare effectively
- Review Core Concepts: Ensure you have a strong understanding of core Java concepts, including object-oriented programming, exception handling, collections, multithreading, and I/O operations.
- Data Structures and Algorithms: Refresh your knowledge of data structures like arrays, linked lists, stacks, queues, trees, graphs, and algorithms like searching, sorting, and dynamic programming.
- Java Libraries and Frameworks: Familiarize yourself with commonly used Java libraries and frameworks such as JDBC, Servlets, JSP, Spring, Spring Boot, Hibernate, and JavaFX. Understand their key features and usage.
- Design Patterns: Study commonly used design patterns in Java and understand their implementation, advantages, and use cases.
- JVM Internals: Gain a basic understanding of JVM internals, garbage collection algorithms, memory management, and performance optimization techniques.
- Practice Coding: Solve coding problems related to Java on platforms like LeetCode or HackerRank. Practice implementing data structures, algorithms, and solving real-world programming challenges.
- Mock Interviews: Arrange mock interviews with friends or colleagues to simulate the interview environment. Receive feedback on your performance and work on improving weak areas.
- Stay Updated: Keep up with the latest trends and updates in the Java ecosystem. Stay informed about new Java versions, features, frameworks, and libraries.
- Prepare Questions: Be prepared to ask relevant questions to the interviewer about the company, project, or team to show your interest and engagement.
- Communication and Confidence: Practice presenting your ideas confidently and be prepared to explain your thought process during technical discussions. Pay attention to your communication skills and body language.
Remember, interview success not only depends on your technical knowledge but also on your problem-solving skills, ability to think critically, and your attitude towards learning and growth. Approach the interview with confidence, be enthusiastic, and showcase your ability to adapt and collaborate.