In this page we have explained some most commonly asked Spring Boot interview question in MNCs from freshers and Experienced. If Your are struggling to find the most important and frequently asked face two face Spring Boot interview questions, Then hold down and take a deep breath because you are at the right place.
I know that It is very tough for fresher candidate as well as experienced candidate while working in company or preparing for college curriculum to prepare for an interview or to finding some great bunch of right questions that cover the most important topics.
In this section, I have tried to cover all important and most asked Spring Boot programming interview questions topics wise.
Let us start Preparing.
Q1). Explain the Spring Boot Key Components?
Ans:
There are four important key components of spring-boot are
- Spring Boot auto-configuration.
- Spring Boot CLI.
- Spring Boot starter POMs.
- Spring Boot Actuators.
There are two more Spring Boot components
- Spring initializr: To quick start new Spring Boot projects
- Spring Boot IDEs: We have multiple Spring Boot IDEs for example : Eclipse IDE, IntelliJ IDEA, Spring STS Suite etc.
Q2). Tell Some List of Sprint Boot Actuator EndPoints
Ans: Some of Spring Boot Actuator End Point are:
- auditevents: This endpoint Exposes the audit events information for the current application.
- beans: Displays the list of all the Spring beans of application.
- health: Display the health information of spring boot application.
- caches: Exposes the all available caches.
- env: Exposes the all properties from Spring’s ConfigurableEnvironment.
- metrics: It Shows the ‘metrics’ information for the current application.
There are some more Spring Actuator endpoints are loggers, mappings, sessions, shutdown, flyway, conditions, etc
Q3). Tell us some benefits of Spring Boot over Spring?
Ans: Spring boot framework is the extension of spring framework to help developers to start coding with low configuration. It eliminates the boilerplate configurations required for setting up a Spring application.
Spring Boot comes with multiple starter dependencies for different Spring modules.
Some commonly used dependencies are
- spring-boot-starter-web
- spring-boot-starter-data-jpa
- spring-boot-starter-security
- spring-boot-starter-test
- spring-boot-starter-thymeleaf
- spring-boot-starter-actuator
Features provided by spring boot but spring doesn’t
- Starter POM
- Auto Configuration
- Component Scanning
- Embedded server
- InMemory DB
- Actuators
- Version Management
Q4). What is the starter dependency of the Spring boot Module?
Ans:
Some of Spring Boot Starter Dependencies are
1). Spring Boot Starter web: Important features of spring-boot-starter-web are It is compatible for web development and Provides Auto configuration. This spring-boot-starter-web dependency pulls all dependencies which is used in the Spring Boot web development.
Below is the code to add Spring Boot Starter web in Spring Boot project using POM.xml.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
2). Spring Boot Starter Actuator : This dependency is used to monitor and manage the Spring Boot application. Below is the code to add Spring Boot Starter Actuator in Spring Boot project using POM.xml.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency>
3). Spring Boot Starter Security: This dependency is used in Spring Boot application to add the Security in Spring Boot Application.
Below is the code to add Spring Boot Starter Security in Spring Boot project using POM.xml.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency>
4). Spring Boot Starter ThymeLeaf : Spring Boot Starter ThymeLeaf Dependency is used to create a web application. It provides a good support for serving a XHTML/HTML5 in web applications.
Below is the code to add Spring Boot Starter ThymeLeaf in Spring Boot project using POM.xml.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency>
5). Spring Boot Starter Test: This dependency is used in Spring Boot Application to write the Unit Test cases of Java methods. Below is the code to add Spring Boot Starter Test in Spring Boot project using POM.xml.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> </dependency>
Q5). What is Spring Boot dependency management?
Ans: Spring Boot dependency management system manages dependencies and configuration automatically. We need not to specify the version of the dependencies in our configuration file. Spring Boot automatically upgrades all dependencies added in the configuration file when we update the Spring Boot version.
Q6) How does Spring Boot work Internally?
Ans:
When we Create Spring Boot Application we add the starter Dependencies in it. This Starter Dependencies automatically configures Spring Boot application based on the dependencies you have added to the project. We add @EnableAutoConfiguration annotation for the configuration.
Suppose if you have not configured any database connection, Spring Boot auto-configures an in-memory database.
The entry point of the spring boot application is the class containing @SpringBootApplication annotation and the main method.
@ComponentScan annotation scans all the components included in the Spring Boot Application automatically.
Q7). Explain Auto Configuration in Spring Boot.
Ans:
Spring Boot Auto Configuration , configures Spring application based on the dependencies we have added in the project.
For Autoconfiguration we will add @EnableAutoConfiguration annotation or @SpringBootApplication annotation in main class file. This annotation will automatically configure Spring Boot application and its dependencies.
Example to use @EnableAutoConfiguration
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
@EnableAutoConfiguration
public class SpringBootApplicationDemo {
public static void main(String[] args) {
SpringApplication.run(SpringBootApplicationDemo.class, args);
}
}
@SpringBootApplication
Class that contains @SpringBootApplication annotation denotes the entry point of the Spring Boot Application. This class should have the main method to run the Spring Boot application. @SpringBootApplication annotation includes Auto- Configuration, Component Scan, and Spring Boot Configuration.
If we add @SpringBootApplication annotation in java class, we need not to add any other annotation like @EnableAutoConfiguration, @ComponentScan and @SpringBootConfiguration.
We can say @SpringBootApplication = @EnableAutoConfiguration + @ComponentScan + @SpringBootConfiguration.
Example to use @SpringBootApplication
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootApplicationDemo {
public static void main(String[] args) {
SpringApplication.run(SpringBootApplicationDemo.class, args);
}
}
Q8) How does a Spring Boot Application get started?
Ans:
Spring Boot application Looks for the class having @SpringBootApplication annotation with the main method. This method serves as an entry point, which invokes the SpringApplication.run method to start the application.
@SpringBootApplication
public class SpringBootApplicationDemo{
public static void main(String[] args) {
SpringApplication.run(SpringBootApplicationDemo.class);
}
}
Q9) Tell some commonly used Spring Boot CLI commands.
Ans:
Some commonly used Spring Boot CLI Commands Are:
-run, -test, -install, -uninstall, -grap, -jar, -war, –init, -shell, -help etc
Q10) Tell Some Basic Annotations Used in Spring Boot?
Ans:
The Spring Boot annotations reside in its org.springframework.boot.autoconfigure package and its sub-packages.
Some Common Spring Boot Annotations are
1). @EnableAutoConfiguration – It makes Spring Boot look for auto-configuration beans on its classpath and automatically apply them.
2). @SpringBootApplication – This annotation is used to denote the main class of a Boot Application. This annotation combines @Configuration, @EnableAutoConfiguration, and @ComponentScan annotations with their default attributes.
Some More annotations of Spring Boot are
For Application Basic Setup
- @SpringBootApplication
- @ComponentScan
- @Configuration
- @EnableAutoConfiguration
For Request Response
- @GetMapping
- @PostMapping
- @RequestMapping
For Component Types
- @Repository
- @Service
- @Component
- @Controller
Q11) What is Spring Boot dependency Management?
Ans:
Spring Boot manages dependencies and configuration automatically. We don’t need to mention the version of the dependencies in our configuration. We can say Dependency Management as it is a way to manage dependencies efficiently in one place. When we update the Spring Boot version Spring Boot upgrades all dependencies automatically.
Q12) How you can Change the Port of the embedded Tomcat server in Spring Boot?
Ans:
There are two ways to change the default port of the server in Spring Boot
1). Using application.properties file
server.port = 8081
In the application.properties file just change the default value of the port to the value that you wanted to be. Here we have given 8081 as a new port value.
2). Using application.yaml file
Server:
Port:8081
Also, you can change the port value from application.yaml by changing the default value of the port to the value that you wanted to be. Here we have also given 8081 as a new port value.
Q13). How you can change the Tomcat server to any other Server in Spring Boot?
Ans:
To Replace the embedded tomcat server in Spring Boot first we have to exclude the default server from the pom.xml. And to add a new server we will add the new dependency.
Example
To exclude tomcat from starter dependency add the below code in pom.xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
And to include a new server add as a dependency. Below is an example to add a jetty server.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jetty</artifactId>
</dependency>
Q14) What is @RestController annotation in Sprint boot?
Ans:
@RestController annotation is a combination of @Controller and @ResponseBody annotation. It is used to make restful web services and also it converts the response to JSON or XML. This annotation is used at the class level and it allows the class to handle the requests made by the client.
Q15). What is the difference between @Controller and @RestController?
Ans:
- With @controller annotation class is responsible for preparing a model Map with data to be displayed by the view But @RestController annotation class is responsible for creating Restful controllers.
- @controller only behaves as a controller, we need to add @responsebody annotation with a method to return value and write it to the HTTP response. But @RestController annotation is a combination of @controller and @responsebody which by default converts return as HTTP Response.
- @Controller is used to mark classes as Spring MVC Controller whereas @RestController annotation mark class as RESTful Web services.
- In @Controller, we need to use @ResponseBody on every handler method but in @RestController we don’t need to use @ResponseBody on every handler method.
- @Controller annotation was added in Spring 2.0 version whereas @RestController annotation was added in Spring 4.0 version.
Q16). What is the difference Between RequestMapping and GetMapping?
Ans:
- @RequestMapping annotation is used with GET, POST, PUT, and other request methods. @getMapping is an extension of @RequestMapping that helps you to improve on the clarity of requests.
- @RequestMapping annotation is used on a class level but the @GetMapping is used on method-level.
Q17). What is Actuator in Spring Boot?
Ans: An actuator in Spring Boot is a feature that helps you to monitor and manage your application when you push it to production. These actuators include auditing, health, CPU usage, HTTP hits, metric gathering, and many more that are automatically applied to your application.
Q18). What is the process to enable Actuator in the Spring boot application?
Ans:
To enable the spring actuator feature, we need to add the dependency of “spring-boot-starter-actuator” in pom.xml.
Enable Actuator in the Spring boot application
<dependency>
<groupId> org.springframework.boot</groupId>
<artifactId> spring-boot-starter-actuator </artifactId>
</dependency>
Q19). Tell some endpoint actuator-provide to monitor the Spring boot application?
Ans:
Actuators provide below pre-defined endpoints to monitor our application –
- Health
- Info
- Beans
- Mappings
- Configprops
- Httptrace
- Heapdump
- Threaddump
- Shutdown
Q20). What is the use of Profiles in spring boot?
Ans:
To Complete the development process of an application or we can say during the development of any application, we deal with multiple environments such as dev, test, and Prod. Sometimes we need specific configurations at each environment level. Suppose we are using an embedded H2 database for dev but for prod, we might have proprietary Oracle.
A profile is a set of configuration settings. Spring Boot allows to the definition of profile-specific property files in the form of application-{profile}.properties. It automatically loads the properties in an application.properties file for all profiles, and the ones in profile-specific property files only for the specified profile.
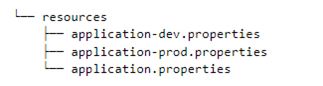
Q21). What is dependency Injection in Spring Boot?
Ans:
The process of injecting dependent objects into the target class is called dependency injection. Suppose our one class is dependent on another class object. In that case, we will provide the other class object to our class. This process is known as dependency injection.
There are 3 ways to inject the dependencies
1. Constructor Injection
This method involves injecting dependencies through the class constructor. It is the recommended approach by the Spring team because it allows you to declare your dependencies as final, ensuring they are not subsequently altered.
@Component
public class MyService {
private final MyRepository myRepository;
@Autowired // Optional in recent Spring versions for single-constructor scenarios
public MyService(MyRepository myRepository) {
this.myRepository = myRepository;
}
}
2. Setter Injection
With setter injection, dependencies are injected through setter methods in the class. This method is useful when working with optional dependencies or when you need to reconfigure beans after they’ve been constructed.
@Component
public class MyService {
private MyRepository myRepository;
@Autowired
public void setMyRepository(MyRepository myRepository) {
this.myRepository = myRepository;
}
}
3. Field Injection
Field injection involves marking a class field with @Autowired
to let Spring inject the dependency directly into the class without explicit setter or constructor.
@Component
public class MyService {
@Autowired
private MyRepository myRepository;
}
Q22). What is an IOC container?
Ans: IoC Container is a framework for implementing automatic dependency injection. It manages object creation and its life-time and also injects dependencies into the class.
Q23). Tell the steps how you will create a spring boot project using Spring Initializer.
Ans:
Spring Initializr is a web tool provided by Spring. Using this tool we can create Spring boot projects by providing project details and giving the jar dependencies details needed for our project.
Steps to create a spring boot project
- First, go to the browser and browse for spring intializr.
- Now Choose the project type maven. Give the details like project name, Group and Artifact details, etc. Now select the required dependencies for the projects. Click on Generate Project and it will download a project to your system.
- After the download of the project extracts this project into your system.
- Open your IDE like eclipse, IntelliJ or STS and import the downloaded and extracted project file using the import project option in IDE.
- After the import project will take some time to internal build and download the dependencies.
- Now you are done with the creation of the spring boot project.
Q24). Tell me the Advantages of the YAML file Over Properties file.
Ans:
- The advantage of the YAML file over the properties file in Spring Boot is, that YAML Stores data in a hierarchical format but the properties file does not.
- YAML file makes easy to read and debug the file for developers.
- The SpringApplication class supports the YAML file as an alternative to properties whenever you use the SnakeYAML library on your classpath.
- One more great advantage of YAML is, suppose we have different deployment profiles such as development, testing, or production, so instead of creating new files for each environment, we can place all the details in a single YAML file. Which is not possible in the case of the properties file.
Q25). Tell some difference between @RequestParam vs @PathVariable Annotations.
Ans:
@RequestParams extract values from the query string and @PathVariables extract values from the URI path.
@PathVariable
Suppose our url structure is http://localhost:8080/springproject/xyz
Then we will use @pathVariable annotation like below to extract some value from the URLs
@GetMapping("/springproject/{id}")
@ResponseBody
public String getIdUsingPathVariable (@PathVariable String id) {
return "ID: " + id;
}
Here id will be print as “xyz”
Or we can also write
@GetMapping("/springproject/{id}")
@ResponseBody
public String getIdUsingPathVariable (@PathVariable("id") String id) {
return "ID: " + id;
}
@RequestParam
Suppose our url structure is http://localhost:8080/springproject?id=xyz
Then we will use @ RequestParam annotation like below to extract some value from the URLs
@GetMapping("/foos")
@ResponseBody
public String getIdUsingRequestParam(@RequestParam("id") String id) {
return "ID: " + id;
}
Here id will be return as “xyz”.
Some More Difference are:
1). @RequestParam encoded the value from url but @PathVariable extracting values from the URI path is not encoded.
@PathVariable
Example:
If the url will be like http://localhost:8080/springproject/x+y
Output will be x+y
Here url value is not decoded.
@RequestParam Example:
http://localhost:8080/springproject?id=x+y
Output will be x y
Here url value is decoded
2). We can make @RequestParam and @PathVariable optional with the help of required attribute.
Below is a Program
@GetMapping("/optional/{id}")
@ResponseBody
public String getIdUsingPathVariable(@PathVariable(required = false) String id) {
return "ID: " + id;
}
For Url http://localhost:8080/optional/xyz
Output will be xyz
And for Url http://localhost:8080/optional/
Ouput will be null
Q26). Tell something about Spring @GetMapping, @PostMapping, @PutMapping, @DeleteMapping and @PatchMapping
Ans:
@GetMapping
- It is a shortcut for @RequestMapping(method = RequestMethod.GET).
- It is used to get single or all values from the database in Spring Application.
@PostMapping
- It is a shortcut for @RequestMapping(method = RequestMethod.POST)
- Method that has @PostMapping annotation is responsible to Create single or multiple entries in the database.
- It is used to create single or multiple value in the database in Spring Application.
@PutMapping
- It is a shortcut for @RequestMapping(method = RequestMethod.PUT)
- Method that has @PutMapping annotation is responsible to update the data in the database.
@PatchMapping
- It is a shortcut for @RequestMapping(method = RequestMethod.PATCH)
- @PatchMapping annotation is used when we want to apply a partial update on the database.
@DeleteMapping
- It is a shortcut for @RequestMapping(method =RequestMethod.DELETE)
- It is used to Delete single or multiple value from the database in the Spring Application.
Q27). What is Thymeleaf in Spring Boot?
Ans: Thymeleaf is a server-side Java-based template engine. It is used for both web and standalone environments. It is capable of processing HTML, XML, JavaScript, CSS and even plain text.
Q28). Tell some embedded containers supported by Spring Boot.
Ans:
There are three embedded containers supported by Spring Boot are :
- Tomcat (used by default)
- Undertow
- Jetty