In this tutorial, you will learn how to write C program to find second largest number in an array.
Below are the approach which we will be follow to write our program:
- We will define an array and take some elements as an input from users to insert in array.
- Then we will iterate array using for loop and compare array elements to find second largest element.
- You can also follow a approach like first sort an array in ascending order and then select the second greatest number in array from last.
- Program is very similar to the find the largest two numbers in a given array. You may refer it
How our program will behave?
As we have already seen above our logic to find the second maximum number of an array.
Our program will take an array as an input.
And on the basis of inputs it will compare each elements and on the basis of comparison it will print second greatest elements from the array.
Program to print second largest number in an array
#include<stdio.h>
#include<conio.h>
#include<stdlib.h>
void secondLargest(int *arr,int size){
int i,firstlargest=0,secondlargest=0;
for(i=0;i<size;i++){
if(arr[i]>firstlargest){
secondlargest=firstlargest;
firstlargest=arr[i];
}
}
printf("%d is second largest",secondlargest);
}
void main(){
int size,i,*arr;
printf("Enter number of Elements in an array n");
scanf("%d", &size);
arr=(int*)malloc(sizeof(int)*size);
printf("please enter the array elements n");
for(i=0; i<size; i++){
scanf("%d",&arr[i]);
}
secondLargest(arr,size);
getch();
}
Output:
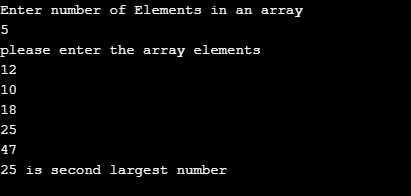
Explanation of above program to find first two maximum number of an array
- In the above program we have taken two int variable size, i and one integer pointer variable arr to declare an array inside main() method.
- size variable will take the size of array given by user and arr will be array which size is calculated by malloc function dynamically at run time.
- Now value will be assigned to each index of an array which is given by user using scanf() function of C.
- We have a function called secondLargest(arr,size) which will take array arr and size of array as an input.
- The body of function secondLargest(int *arr, int size) has all the logic of finding second largest number of an array.
- Method of secondLargest(int *arr, int size) have three variables i, firstlargest and secondlargest variable.
- And for loop is to iterated the input array of secondlargest() method.
- In for loop using if statement we are comparing the two elements of an array and then decide which one is greatest and second greatest.
- If we find greatest then if statement will satisfy the condition and replace the old largest with new largest.
- Program is similar to finding the largest two numbers in a given array. Here we only printing the second largest number as per our program requirement.
This was the all logic behind finding second largest element of given array.