In this tutorial, you will learn how to write C program to find largest and smallest number in an array.
Below are the approach which we will be follow to write our program:
- At first we will take inputs from the users in array.
- Then we select first element as a largest as well as smallest. It is an assumptions.
- Now we will traverse each elements of an array and compare with largest one (which we have selected first element).
- Now if we found element which largest than our assume value then we will replace old largest value with new one and follow the same process until we traverse all elements of an array.
- And for the smallest one we follow the same approach. If we found next smallest then we will replace it from old one and follow the same approach until we traverse all array elements.
How our program will behave?
Our program will take one array and on the basis of logic it will print greatest and smallest number of an array.
Suppose if we give an array as 34, 22, 1, 90, 114, 87
Then our program should give output as:
Largest number is 114
Smallest number is 1
C Program to find largest and smallest number in an array
#include<stdio.h>
#include<conio.h>
void LargestAndSmallest(int *arr,int size){
int i,largest=arr[0],smallest=arr[0];
for(i=1;i<size;i++){
if(arr[i]>largest){
largest =arr[i];
}
if(arr[i]<smallest){
smallest = arr[i];
}
}
printf("%d is largest and %d is smallest",largest,smallest);
}
int main(){
int n,i;
printf("enter the size of an array : ");
scanf("%d",&n);
int arr[n];
printf("please enter the elements of array n");
for(i=0;i<n;i++){
scanf("%d",&arr[i]);
}
LargestAndSmallest(arr,n);
getch();
}
Output:
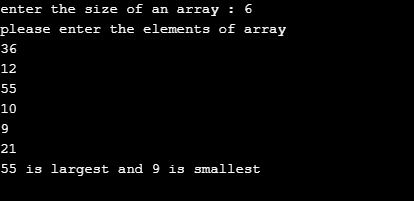
Explanation of the above
- In the above program we have one array arr of integer type and two variables n and i also of integer type in main() method.
- First we will ask user to enter the size of an array and then as per size we will ask user to insert value in array.
- We have a method LargestAndSmallest(arr,n). Inside its body all the logic of finding largest and smallest number of an array is written.
- In method’s LargestAndSmallest(int *arr,int size) body, there are three variable i, largest and smallest.
- And using for loop we are iterating each elements of an array and there are if statement inside the for loop to compare the two elements and decide which is smallest and which is largest.
- Using if statement, if we found largest value in array then we will assign it in largest variable. Similar if we found smallest then assign to smallest variable.
- And at last after the finding greatest and smallest we will print the greatest and smallest number using printf() function of C.
This was the all logic to find the greatest and smallest number in an array using C programming language.
I hope you have understand this concept well.