In this tutorial, you will learn how to write C program to delete duplicate elements in an array.
Below are the approach which we will be follow to write program:
- In this program first we will take an array and insert some elements in it.
- And then we will count the occurrence of each elements of an array.
- Print only those elements which has occurs only one time.
- Not print those elements which has occurred more than one times.
How our program will behave?
Our program will take an array as an input.
And then count the occurrence of each elements. After the count it will print only those elements as an output which has occurs only one time.
Program to remove duplicate elements from array using C
#include<stdio.h>
#include<conio.h>
#include<stdlib.h>
#define MAX 100
void nonDuplicate(int *arr,int size){
int i,j=0;
int ar[MAX] = {0};
for(i=0;i<size;i++){
ar[arr[i]]++;
if(ar[arr[i]]==1){
printf("%dn",arr[i]);
}
}
}
void main(){
int size,i,*arr;
printf("Please enter the array size (not max to 100) : n");
scanf("%d", &size);
arr=(int*)malloc(sizeof(int)*size);
printf("please enter the array elements n");
for(i=0; i<size; i++){
scanf("%d",&arr[i]);
}
printf("After removing duplicate elements:n");
nonDuplicate(arr, size);
getch();
}
Output:
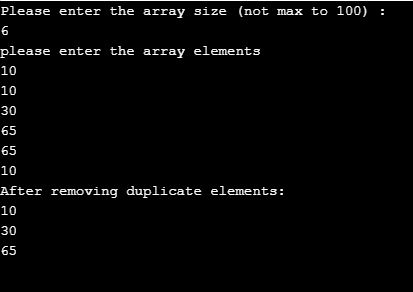
Explanation of above program to remove duplicate elements from array in C
- In the above program we have taken two int variable size, i and one integer pointer variable arr to point the array inside main() method.
- size variable will take the size of array given by user and arr will be array which size is calculated by malloc function dynamically at run time.
- Now value will be assigned to each index of an array which is given by user using scanf function of C.
- We have a function called nonDuplicate(arr,size) which will take array arr and size of array as an input.
- The body of function nonDuplicate(int *arr, int size) has all the logic of removing all the duplicate elements from the array.
- Method
- nonDuplicate(int *arr, int size) have two variables i and j. And int array ar to hold count of the occurrence of array element.
- And for loop is to iterated the input and count the occurrence of each array elements. And same time print elements which occurs first time.
- If same element will occur next time which we can check using if statement where we are comparing the occurrence.
- Then not print that element because we have already printed.
- If I elaborate, if statememt will print only those elements of an array which has occurs only one time and skip those which has occurs more than one times.
This is the logic behind removing the duplicate element of an array which has occurs more than one times using C program.
We are not removing from array, But our logic is printing element only first time.
I hope it is clear to all.