In this tutorial, we will learn writing python program to create an array (list in case of python) and return the reverse of elements stored in the array (list).
Problem Statement
Our program will first take the input of array (list) size and then the elements of the array (list) by the user. After applying some logic it will return the reverse of the input array (list).
[elementor-template id=”5253″]
For example:
Case 1: if the user inputs 4 as array (list) size and the array (list) elements as 1,2,3,4.
The output should be 4,3,2,1.
Case 2: if the user inputs 5 as array (list) size and the array (list) elements as 9,8,7,6,5.
The output should be 5,6,7,8,9.
Our logic to print an array(list) in reverse order using while loop
- Our program will take input to fix the size of the array (list).
- Create two empty lists.
- Then our program will run for a ‘for loop’ to take the inputs from the user. The inputs will be the elements (or the content) of the array (list).
- Then our program will use a while loop to add elements from the last of the list.
Algorithm to print an array(list) in reverse order using while loop
Step 1: Start
Step 2: take an input from the user (let’s say size).
Step 3: Create two empty lists.
Step 4: Add elements to the list
Step 5: startIndex = 0;
lastIndex = size – 1;
while lastIndex>=0:
revArr.append(arr[lastIndex])
startIndex+=1
lastIndex-=1
Step 6: Print reversed list i.e. revArr
Step 7: Stop
[elementor-template id=”5257″]
Python code to print an array(list) in reverse order using while loop
output 1:
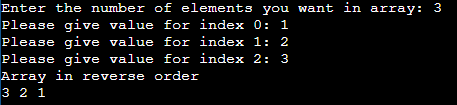
[elementor-template id=”5256″]
output 2:
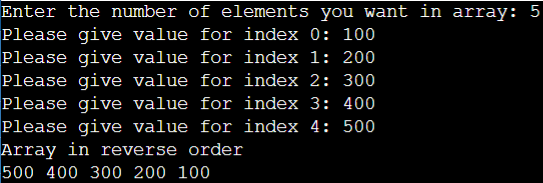