In this tutorial, you will learn how to write Python program to find largest and smallest number in an array.
To find largest and smallest in an array we find compare each elements to each other and find which one is greatest and which one is smallest.
In this program we have taken two variable largest and smallest which will hold number.
Python Program to find largest and smallest number in an array
arr = []
n = int(input("enter size of array : "))
for x in range(n):
x=int(input("enter element of array : "))
arr.append(x)
largest = arr[0]
smallest = arr[0]
for i in range(n):
if arr[i]>largest:
largest = arr[i]
if arr[i]<smallest:
smallest = arr[i]
print(f"largest element in array is {largest}")
print(f"smallest element in array is {smallest}")
Output:
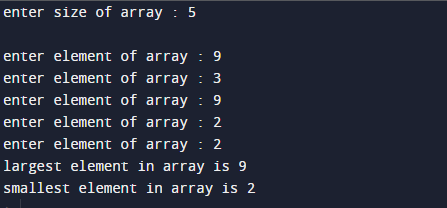