In this tutorial, you will learn how to write Python program to find second largest number in an array.
To find the second largest element, First sort the given array in ascending order. Then select the second last element from the list.
It may happen similar number repeating two times, then also check two number should be different.
For example:
Example 1 : Array 2, 3, 4, 5
Second largest is 4
Example 2 : Array 2, 3, 4, 5, 6, 6
In this case 5 is second largest element. In this case we take care if last two or three elements is similar.
Python Program to print second largest number in an array
import array
arr = []
n = int(input("enter size of array : "))
for x in range(n):
x=int(input("enter element of array : "))
arr.append(x)
sorted_array = sorted(array.array('i', arr))
for i in range(len(arr)-1, 0, -1):
if sorted_array[i]!=sorted_array[i-1]:
print(f"second largest element is {sorted_array[i-1]}")
break
Output:
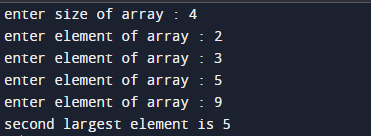