Below are the various examples of Rectangle patterns that are made using the combination of stars. This Rectangle pattern includes Solid and hollow Rectangle. And the program to print that Rectangle are written in C programming language.
And below C concepts are used to print that patterns
- For Loop
- While Loop
- if..else
1). Program to print Solid Rectangle using star(*) in C
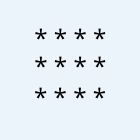
#include<stdio.h>
#include<conio.h>
void main(){
int i, j, rows, columns;
printf("This is Rectangle program \n");
printf("Enter number of rows: ");
scanf("%d", &rows);
printf("Enter number of columns: ");
scanf("%d", &columns);
for(i=1; i<=rows; i++)
{
for(j=1; j<=columns; j++)
{
printf("*");
}
printf("\n");
}
getch();
}
Output
This is Rectangle program
Enter number of rows: 3
Enter number of columns: 5
*****
*****
*****
2). Program to print hollow Rectangle using star(*) in C
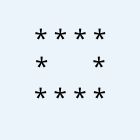
#include<stdio.h>
#include<conio.h>
void main()
{
int i, j, rows, columns;
printf("Hollow Reactangle Program \n");
printf("Enter number of rows: ");
scanf("%d", &rows);
printf("Enter number of columns: ");
scanf("%d", &columns);
for(i=1; i<=rows; i++)
{
for(j=1; j<=columns; j++)
{
if(i==1 || i==rows || j==1 || j==columns)
{
printf("*");
}
else
{
printf(" ");
}
}
printf("\n");
}
getch();
}
Output
Hollow Reactangle Program
Enter number of rows: 4
Enter number of columns: 7
*******
* *
* *
*******