Below are the various examples of Star Hyphen Combination Patterns. This Combination includes the left half-diamond pattern, the right half-diamond pattern using a star and hyphen, etc. And the program to print that pattern are written in C programming language.
And below C concepts are used to print that patterns
- For Loop
- While Loop
- if..else
1). Program to Print left half Diamond pattern using Star(*) and Hyphen(-) in C
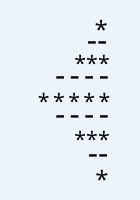
#include<stdio.h>
#include<conio.h>
void main(){
int i,j,k,n;
printf("enter the value n:");
scanf("%d",&n);
for(i=0;i<n;i++)
{
for(j=0;j<n-i;j++){
printf(" ");
}
if(i%2==0){
for(k=0;k<=i;k++){
printf("*");
}
}else{
for(k=0;k<=i;k++){
printf("-");
}
}
printf("\n");
}
for(i=n-1;i>0;i--)
{
for(j=n;j>=i;j--){
printf(" ");
}
if(i%2==0){
for(k=i;k>0;k--){
printf("-");
}
}else{
for(k=i;k>0;k--){
printf("*");
}
}
printf("\n");
}
getch();
}
Output
enter the value n:5
*
--
***
----
*****
----
***
--
*
2). Program to print Right Half Diamond Pattern using Star(*) and Hyphen(-) in C
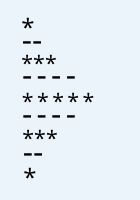
#include<stdio.h>
#include<conio.h>
void main(){
int i,j,k,n;
printf("enter the value of n:");
scanf("%d",&n);
for(i=1;i<=n;i++)
{
if(i%2==0){
for(k=1;k<=i;k++){
printf("-");
}
}else{
for(k=1;k<=i;k++){
printf("*");
}
}
printf("\n");
}
for(i=n;i>1;i--)
{
if(i%2==0){
for(k=i;k>1;k--){
printf("*");
}
}
else{
for(k=i;k>1;k--){
printf("-");
}
}
printf("\n");
}
getch();
}
Output
enter the value of n:6
*
--
***
----
*****
------
*****
----
***
--
*
3). Program to print Triangle Pattern using Star(*) and Hyphen(-) in C
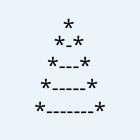
#include<stdio.h>
#include<conio.h>
void main()
{
int i,j,n,k;
printf("enter the value of n:");
scanf("%d",&n);
for(i=0;i<n;i++){
for(k=1;k<n-i;k++){
printf(" ");
}
printf("*");
for(j=0;j<=i-1;j++){
printf("-");
}
for(j=1;j<i;j++){
printf("-");
}
if(i>0){
printf("*");
}
printf("\n");
}
getch();
}
Output
enter the value of n:5
*
*-*
*---*
*-----*
*-------*
4). Program to print Full Diamond Pattern Using Star(*) and Hyphen(-) in C
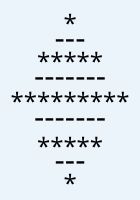
#include<stdio.h>
#include<conio.h>
void main(){
int i,j,k,n;
printf("enter the value of n:");
scanf("%d",&n);
for(i=1;i<=n;i++)
{
for(j=1;j<=n-i;j++){
printf(" ");
}
if(i%2==1){
for(k=1;k<=2*i-1;k++){
printf("*");
}
}else{
for(k=1;k<=2*i-1;k++){
printf("-");
}
}
printf("\n");
}
for(i=n;i>=1;i--)
{
for(j=n;j>=i;j--){
printf(" ");
}
if(i%2==1){
for(k=2*i-2;k>1;k--){
printf("-");
}
}else{
for(k=2*i-2;k>1;k--){
printf("*");
}
}
printf("\n");
}
getch();
}
Output
enter the value of n:5
*
---
*****
-------
*********
-------
*****
---
*