Below are the various examples of Rhombus patterns that are made using the combination of stars. This Rhombus pattern includes left and right inclined rhombus. And the program to print that Rhombus are written in C programming language.
And below C concepts are used to print that patterns
- For Loop
- While Loop
- if..else
1). Program to print right Inclined Solid Rhombus using star(*) in C
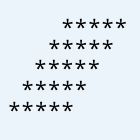
#include<stdio.h>
#include<conio.h>
int main()
{
int i,j,n,k;
printf("Enter the number of rows for Rhombus: ");
scanf("%d",&n);
for(i=0;i<n;i++){
for(k=1;k<n-i;k++){
printf(" ");
}
for(j=0;j<=n;j++){
printf("*");
}
printf("\n");
}
return 0;
}
Output
Enter the number of rows for Rhombus: 5
******
******
******
******
******
2). Program to print left inclined Solid Rhombus using star(*) in C
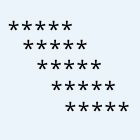
#include<stdio.h>
#include<conio.h>
int main()
{
int i,j,n,k;
printf("Enter the number of rows for Rhombus: ");
scanf("%d",&n);
for(i=n;i>=1;i--){
for(j=1;j<=n-i;j++){
printf(" ");
}
for(k=1;k<=n;k++){
printf("*");
}
printf("\n");
}
return 0;
}
Output
Enter the number of rows for Rhombus: 5
*****
*****
*****
*****
*****