In this tutorial, we will learn to create an array (list in case of python) and rotate the elements stored in the array (list) by two positions using python.
That means if our array (list) is:

After two rotations:

Problem Statement
Our program will first take the input of array (list) size and then the elements of the array (list). Then, our program will rotate the elements of the array (list) by 2.
For example:
Case 1: if the user inputs 4 as array (list) size and the array (list) elements as 1,2,3,4.
The output should be 3, 4, 1, 2.
Case 2: if the user inputs 5 as array (list) size and the array (list) elements as 9,8,7,6,5.
The output should be 6,5,9,8,7.
[elementor-template id=”5257″]
Our logic to perform right rotation on array (list) elements by two positions
- Our program will take input to fix the size of the array (list).
- Then our program will run for a ‘for loop’ to take the inputs from the user. The inputs will be the elements (or the content) of the array (list).
- Then, by using the concept of ‘nested loop’, our program will rotate the array (list) by 2 positions.
- Print the final array (list)
[elementor-template id=”5256″]
Algorithm to perform right rotation on array (list) elements by two positions
Step 1: Start
Step 2: take an input from the user (let’s say size).
Step 3: Create an empty list and a variable sum with value assign is 0.
Step 4: for i in range(0,size):
elem=int(input(“Please give value for index “+str(i)+”: “))
arr.append(elem)
Step 5: for i in range(0,2):
temp=arr[size-1];
for j in range(size-1,-1,-1):
arr[j]=arr[j-1]
arr[0]=temp;
Step 6: print arr
Step 7: Stop
Python code to perform right rotation on array (list) elements by two positions
Output :
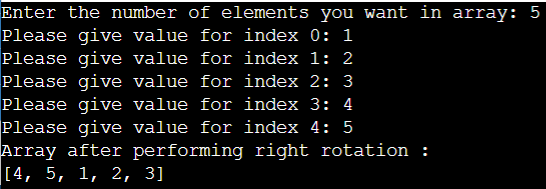
Explanation:
For the input array (list) : [1, 2, 3, 4, 5], the for loop will rotate the array 2 times.
For 1st rotation the array becomes : [5, 1, 2, 3, 4].
And finally at 2nd rotation the array becomes : [4, 5, 1, 2, 3]