In this tutorial, we will learn to create an array (list in case of python) and rotate the elements stored in the array (list) by ‘n’ positions. The value of ‘n’ is also taken input by the user.
That means if our array (list) is:

After the user inputs the rotation value 3, then the array (list) becomes:

Problem Statement
Our program will first take the input of array (list) size and then the elements of the array (list). After that our program will rotate the elements of the array (list) by the value given by the user.
[elementor-template id=”5253″]
For example:
Case 1: if the user inputs 4 as array (list) size and the array (list) elements as 1,2,3,4. And if the user inputs 3 as the rotation value then, The output should be 2,3,4,1.
Case 2: if the user inputs 5 as array (list) size and the array (list) elements as 9,8,7,6,5. And if the user inputs 1 as the rotation value then, The output should be 5,9,8,7,6.
Our logic to perform right rotation of array (list) elements by n positions
- Our program will take input to fix the size of the array (list).
- Then our program will run for a ‘for loop’ to take the inputs from the user. The inputs will be the elements (or the content) of the array (list).
- Take another input ‘n’ from the user as the rotation value for array (list).
- Then, by using the concept of ‘nested loop’, our program will rotate the array (list) by ‘n’ positions.
- Print the final array (list)
[elementor-template id=”5257″]
Algorithm to perform right rotation on array (list) elements by n positions
Step 1: Start
Step 2: take an input from the user (let’s say size).
Step 3: Create an empty list and a variable sum with value assign is 0.
Step 4: for i in range(0,size):
elem=int(input(“Please give value for index “+str(i)+”: “))
arr.append(elem)
Step 5: for i in range(0,2):
temp=arr[size-1];
for j in range(size-1,-1,-1):
arr[j]=arr[j-1]
arr[0]=temp;
Step 6: print arr
Step 7: Stop
[elementor-template id=”5256″]
Python code to perform right rotation on array (list) elements by n positions
Output 1:
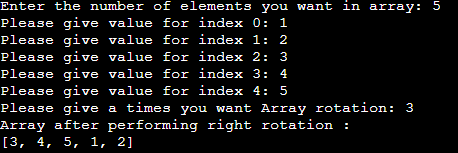
Explanation:
For the input array (list) : [1, 2, 3, 4, 5], and the user inputs the rotation value as 3, the for loop will rotate the array 3 times.
For 1st rotation the array becomes : [5, 1, 2, 3, 4].
For 2nd rotation the array becomes : [4, 5, 1, 2, 3] .
And finally for 3rd rotation the array becomes : [3, 4, 5, 1, 2]
Output 2:
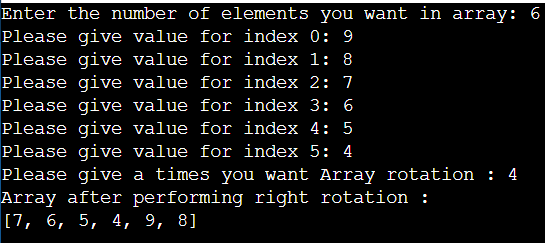
Explanation:
For the input array (list) : [9, 8, 7, 6, 5, 4], and the user inputs the rotation value as 3, the for loop will rotate the array 4 times.
For 1st rotation the array becomes : [4, 9, 8, 7, 6, 5].
For 2nd rotation the array becomes : [5, 4, 9, 8, 7, 6] .
For 3rd rotation the array becomes : [6, 5, 4, 9, 8, 7] .
And finally for the 4th rotation the array becomes : [7, 6, 5, 4, 9, 8]