In this tutorial, we are going to learn writing a python program to calculate the Highest Common Factor of two numbers. In this program we will use iteration concept.
Problem Statement
For any two numbers that are inputs given by the user, we have to calculate and print the h.c.f. of that numbers.
For example:
Case1: If the user inputs the numbers 4 and 6.
then the output should be ‘2’.
Case2: If the user inputs the numbers 5 and 7.
then the output should be ‘1’.
Our logic to find h.c.f. of two numbers
- Our program will take two inputs numbers from the user.
- Using the ‘if-else’ conditional statement, we find the minimum of two input integers and store it in the ‘minimum’ variable.
- We will use ‘for loop’ with ‘range’ function with the argument, 1 as start, and minimum+1 as the stop.
- Inside the ‘for loop’ block, we find the highest factor which is common to both input numbers.
- Print the HCF as the output of our program.
Algorithm to find h.c.f. of two numbers
Step 1: Start
Step 2: take two integer inputs from the user and store them in num1 and num2 variable
Step 3:if num1>num2:
minimum = num2
else:
minimum = num1
Step 4: for i in range (1, minimum+1):
if ((num1%i==0) and (num2%i==0)):
hcf = i
Step 5: print hcf
Step 6: Stop
Python code to find h.c.f. of two numbers
Output 1:
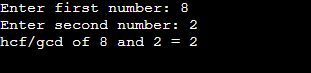
Explanation:
For inputs 8 and 2, the program is finding the highest factor that is common in both 8 and 2.
The factor of 8 is 1,2,4,8 and the factor of 2 is 1,2. The highest factor common in both is 2.
Output 2:
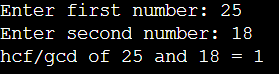
Explanation:
For inputs 25 and 18, the program is finding the highest factor that is common in both 25 and 18.
The factor of 25 is 1,5,25 and the factor of 18 is 1,2,3,6,9,18. The highest factor common in both is 1.