In this tutorial you will learn writing program for how to remove a character from a string in java.
Before start writing this program you should know how to work with String and character in Java.
Also Read This: C program to remove a character from String.
How this Java program will behave?
This program will take a String as an Input and also a character as an Input.
This input is a character that we want to remove from a given String.
For Example: I have given a String “Quescol” as an input and also a character ‘e’ as an input.
We will get Output as “Quscol”.
Function to remove character from String in Java
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner sc= new Scanner(System.in);
System.out.print("Please give a String : ");
String str= sc.nextLine();
Scanner sc1= new Scanner(System.in);
System.out.print("Please give a Char : ");
String ch= sc1.nextLine();
System.out.println(charToRemove(str, ch));
}
public static String charToRemove(String str, String ch){
return str.replace(ch," ");
}
}
Output:
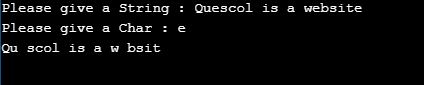