In this tutorial, you will learn how to write C Program to find middle element of a linked list in single pass.
Below are the approach which we will be follow to write our program:
- So first of all we will take some inputs from the users and then we will try to find the middle of a linked list.
- It is very easy to decide middle if we have odd number of nodes in count in linked list like 5. Means 3rd node is middle node.
- But if we have even number of node in count like 4. then we will consider 3rd element as a middle in our program.
How our program will behave?
Our program will take one node as an input and add to linked list.
After taking the node program will give options to select options what you want to perform.
This program has 4 operations. You can select any one as per your requirement.
This 4 operation are:
- Add value to the list at end, it is to insert node in the list.
- Traverse/View list. It is for showing complete list.
- This 3rd option is for finding middle of a singly linked list.
- to exit the program.
Program to find middle element of a linked list in single pass
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 | #include<conio.h> #include<stdio.h> #include<stdlib.h> struct node{ int data; struct node *next; }; struct node *head=NULL; struct node* createNode(){ struct node *newNode = (struct node *)malloc(sizeof(struct node)); return (newNode); } void insertNodeAtEnd(){ struct node *temp,*ptr; temp=createNode(); printf("enter the data you want to insert:"); scanf("%d",&temp->data); temp->next=NULL; if(head==NULL) head=temp; else{ ptr=head; while(ptr->next!=NULL){ ptr=ptr->next; } ptr->next=temp; } } void viewList(){ struct node* temp=head; if(temp==NULL){ printf("List is empty. Please insert some data in list n"); } else{ printf("Our list is : n"); while(temp->next!=NULL) { printf("%dt",temp->data); temp=temp->next; } printf("%d t",temp->data); } } void findMiddle(){ struct node* t= head; int elements=0; while(t){ t=t->next; elements++; } int middle = elements/2; t=head; for (int i = 1; i < middle + 1; i++) t = t->next; printf("middle number of list is :n "); printf("%d",t->data); } int menu(){ int choice; printf("n 1.Add value to the list at end"); printf("n 2.Travesre/View List"); printf("n 3.Print Middle element of a list "); printf("n 4.exit"); printf("n Please enter your choice: t"); scanf("%d",&choice); return(choice); } void main(){ while(1){ switch(menu()){ case 1: insertNodeAtEnd(); break; case 2: viewList(); break; case 3: findMiddle(); break; case 4: exit(0); default: printf("invalid choice"); } getch(); } } |
Output:
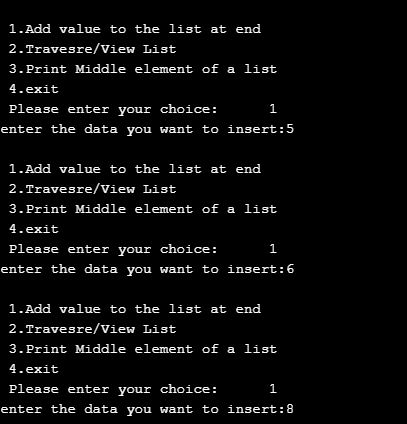
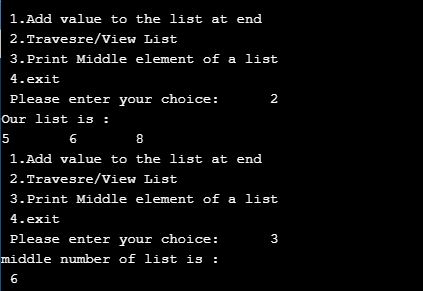
Explanation of above program to find middle element of a linked list in single pass
- In the above program we have menu() function to print the options of operations each times, and inside main() function we have one switch case statement with 4 cases and one default.
- Also we have 3 functions to perform operations on linked list. insertNodeAtEnd() function is responsible to insert the node in a linked list if already created, otherwise create new Linked List.
- viewList() function is responsible to print each elements of a linked list.
- findMiddle() function is responsible to find the middle element of a linked list and then print it.