In this tutorial, you will learn how to write C program to find 3rd element from the last of a singly linked list.
Below are the approach which we will be follow to write our program:
- This very simple program. We just Count the total number of node and then print 3rd node element from the last.
How our program will behave?
In this program we will create linked list by taking node data as an input from users.
This program has 4 operations. You can select any one as per your requirement.
This 4 operation are:
- Add value to the list at end, it is to insert node in the list.
- Traverse/View list. It is for showing complete list.
- find the 3rd element from the last of linked list. This is our main operation which we want to perform in this program.
- to exit the program.
C Program to find 3rd last element of singly linked list
#include<conio.h>
#include<stdio.h>
#include<stdlib.h>
struct node{
int data;
struct node *next;
};
struct node *head=NULL;
struct node* createNode(){
struct node *newNode = (struct node *)malloc(sizeof(struct node));
return (newNode);
}
void insertNodeAtEnd(){
struct node *temp,*ptr;
temp=createNode();
printf("enter the data you want to insert:");
scanf("%d",&temp->data);
temp->next=NULL;
if(head==NULL)
head=temp;
else{
ptr=head;
while(ptr->next!=NULL){
ptr=ptr->next;
}
ptr->next=temp;
}
}
void viewList(){
struct node* temp=head;
if(temp==NULL){
printf("List is empty. Please insert some data in list n");
}
else{
printf("Our list is : n");
while(temp->next!=NULL)
{
printf("%dt",temp->data);
temp=temp->next;
}
printf("%d t",temp->data);
}
}
void get3rdElement(){
struct node* t= head;
int elements=0;
while(t){
t=t->next;
elements++;
}
if(elements<3){
return;
}else{
t=head;
for (int i = 1; i < elements - 3 + 1; i++)
t = t->next;
printf("3rd element of linked list is : n");
printf("%d",t->data);
}
}
int menu(){
int choice;
printf("n 1.Add value to the list at end");
printf("n 2.Travesre/View List");
printf("n 3.Print 3rd element of a list ");
printf("n 4.exit");
printf("n Please enter your choice: t");
scanf("%d",&choice);
return(choice);
}
void main(){
while(1){
switch(menu()){
case 1:
insertNodeAtEnd();
break;
case 2:
viewList();
break;
case 3:
get3rdElement();
break;
case 4:
exit(0);
default:
printf("invalid choice");
}
getch();
}
}
Output:
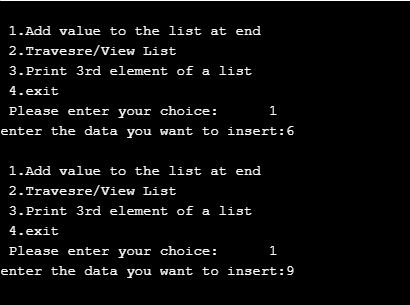
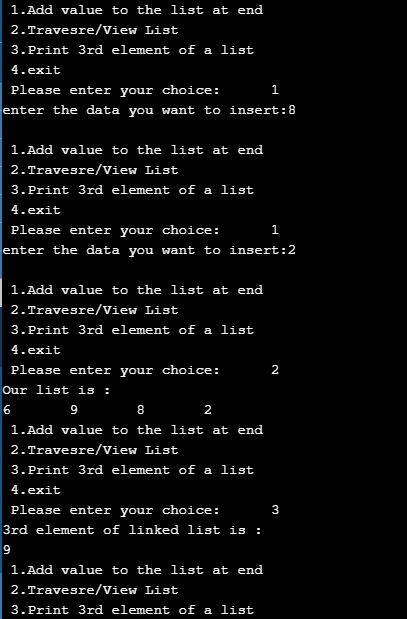
Explanation of above program to reverse of a singly linked list in c
- In the above program we have menu() function to print the options of operations each times, and inside main() function we have one switch case statement with 4 cases and one default.
- Also we have 3 functions to perform operations on linked list. insertNodeAtEnd() function is responsible to insert the node in a linked list if already created, otherwise create new Linked List.
- viewList() function is responsible to print each elements of a linked list.
- get3rdElement() function is responsible to print 3rd element from the last of a given linked list.