In this tutorial, you will learn how to write Bubble sort program in C with explanation.
Before going on logic of bubble sort or program first lets understand how bubble sort works? What is the space and time complexity of it?
What is Bubble Sort?
Bubble sort is very easy and basic sorting technique. In Bubble sorting each adjacent pair elements compare each other and swap if its order is wrong otherwise left as it is.
Now for next loop same process will continue again until it array gets sorted
Lets see how its works
Suppose In array we have 4, 2, 5, 8, 1, 6 elements.
Below I have shown how elements gets sorted using bubble sort in each steps.
Pass 1: 2, 4, 5, 1, 6, 8
Pass 2: 2, 4, 1, 5, 6, 8
Pass 3: 2, 1, 4, 5, 6, 8
Pass 4: 1, 2, 4, 5, 6, 8
Our approach to write bubble sort program in C
Below are the approach which we will be follow to write our program:
- At the first we will give random number to an array which we want to sort.
- For loop will help us to iterate the each elements of an array so that we can perform our comparison to sort and arrange array elements in ascending order.
- In bubble sort we have to compare each elements with its adjacent and swap elements if its order is not in ascending order.
- We will use two for loop. One for loop for paas and second for loop to compare its adjacent element and swap the element if order is not in ascending order.
- And if all elements sorted in ascending order then print the elements.
Complexity
Best Case : O(n)
Average and Worst Case : O(n^2)
How our program will behave?
Our program will take an unsorted or sorted array as an input and will give sorted array which is in ascending order as an output after applying bubble sort.
For Example :
suppose if i give array elements as 5, 6, 3, 1, 9
Then in output it should give
1, 3, 5, 6, 9
C Program to sort an array in ascending order using Bubble sort
#include<stdio.h>
#include<conio.h>
void main(){
int arr[100],i,j,n,key;
printf("This is bubble sort n");
printf("enter the size of an array:");
scanf("%d",&n);
for(i=0;i<n;i++){
scanf("%d",&arr[i]);
}
for(i=0;i<n;i++)
{
for(j=0;j<n;j++){
if(arr[j]>arr[j+1])
{
key=arr[j];
arr[j]=arr[j+1];
arr[j+1]=key;
}
}
}
printf("After sorting: n");
for(i=0;i<n;i++){
printf("%d t",arr[i]);
}
getch();
}
Output:
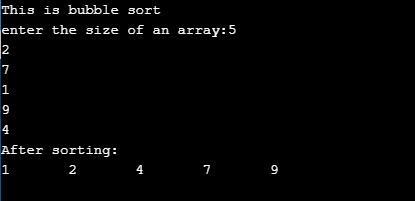
Explanation of above program to sort an array using bubble sort
- In the above program we have one array arr of int type, and 4 variables i, j, n, key.
- Now using for loop we will take input from user to put in array.
- key variable is used to swap the array element if right element is smaller then left element as per condition.
- And At last we can print sorted array after iterating elements of an array.