Below C concepts are used to print that patterns
- For Loop
- While Loop
- if..else
1). Program to print half pyramid pattern using star(*) in Java
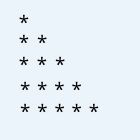
import java.util.*;
class Main{
public static void main(String ...a){
int i=0,temp=0,j;
Scanner sc= new Scanner(System.in);
System.out.print("Enter the number of rows for half pyramid: ");
int n= sc.nextInt();
for(i=1;i<=n;i++){
for(j=1;j<=i;j++){
System.out.print("*");
}
System.out.println("");
}
}
}
Output
Enter the number of rows for half pyramid: 5
*
**
***
****
*****
2). Program to print Inverted half Pyramid Pattern using Star(*) in Java
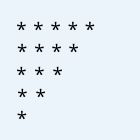
import java.util.*;
class Main{
public static void main(String ...a){
int i=0,temp=0,j;
Scanner sc= new Scanner(System.in);
System.out.print("Enter the number of rows for half pyramid: ");
int n= sc.nextInt();
for(i=n;i>=1;i--){
for(j=i;j>=1;j--){
System.out.print("*");
}
System.out.println("");
}
}
}
Output
Enter the number of rows for half pyramid: 5
*****
****
***
**
*
3). Program to print Right Half Pyramid Pattern using Star(*) in Java
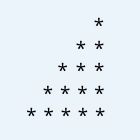
import java.util.*;
class Main{
public static void main(String ...a){
int i=0,temp=0,j,k;
Scanner sc= new Scanner(System.in);
System.out.print("Enter the number of rows for half pyramid: ");
int n= sc.nextInt();
for(i=n;i>=1;i--){
for(j=i;j>=2;j--){
System.out.print(" ");
}
for(k=1;k<=n-i+1;k++){
System.out.print("*");
}
System.out.println("");
}
}
}
Output
Enter the number of rows for half pyramid: 5
*
**
***
****
*****
4). Program to print Inverted Right half Pyramid Pattern using star(*) in Java
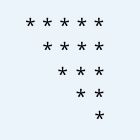
import java.util.*;
class Main{
public static void main(String ...a){
int i=0,temp=0,j,k;
Scanner sc= new Scanner(System.in);
System.out.print("Enter the number of rows for half pyramid: ");
int n= sc.nextInt();
for(i=n;i>=1;i--){
for(j=1;j<=n-i;j++){
System.out.print(" ");
}
for(k=1;k<=i;k++){
System.out.print("*");
}
System.out.println("");
}
}
}
Output
Enter the number of rows for half pyramid: 5
*****
****
***
**
*
5). Program to Print full Pyramid pattern using Star(*) in Java
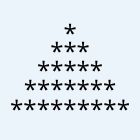
import java.util.*;
class Main{
public static void main(String ...a){
int i=0,temp=0,j,k;
Scanner sc= new Scanner(System.in);
System.out.print("Enter the number of rows for pyramid: ");
int n= sc.nextInt();
for(i=n;i>=1;i--){
for(j=i;j>=2;j--){
System.out.print(" ");
}
for(k=1;k<=2*(n-i+1)-1;k++){
System.out.print("*");
}
System.out.println("");
}
}
}
Output
Enter the number of rows for pyramid: 5
*
***
*****
*******
*********
6). Program to print Inverted full Pyramid pattern using Star(*) in Java
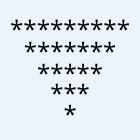
import java.util.*;
class Main{
public static void main(String ...a){
int i=0,temp=0,j,k;
Scanner sc= new Scanner(System.in);
System.out.print("Enter rows for Inv. Full Pyramid Pattern: ");
int n= sc.nextInt();
for(i=n;i>=1;i--){
for(j=1;j<=n-i;j++){
System.out.print(" ");
}
for(k=1;k<=2*i-1;k++){
System.out.print("*");
}
System.out.println("");
}
}
}
Output
Enter rows for Inv. Full Pyramid Pattern: 5
*********
*******
*****
***
*
7). Program to print half pyramid pattern using numbers in Java
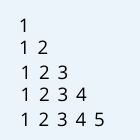
import java.util.*;
class Main{
public static void main(String ...a){
int i,j,k;
Scanner sc= new Scanner(System.in);
System.out.print("Enter no. of rows for half pyramid: ");
int n= sc.nextInt();
for(i=1;i<=n;i++){
for(j=1;j<=i;j++){
System.out.print(j);
}
System.out.println("");
}
}
}
Output
Enter no. of rows for half pyramid: 5
1
12
123
1234
12345
8). Program to print Inverted half Pyramid Pattern using numbers in Java
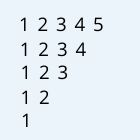
import java.util.*;
class Main{
public static void main(String ...a){
int i=0,temp=0,j,k;
Scanner sc= new Scanner(System.in);
System.out.print("Enter the number of rows for half pyramid: ");
int n= sc.nextInt();
for(i=0;i<n;i++){
for(j=1;j<=n-i;j++){
System.out.print(j);
}
System.out.println("");
}
}
}
Output
Enter the number of rows for half pyramid: 5
12345
1234
123
12
1