Support this post with a reaction:
Below Java concepts are used to print that patterns
- For Loop
- While Loop
- if..else
1). Program to print Half Pyramid Pattern using Star(*) in Java
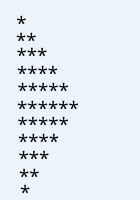
import java.util.*;
class Main{
public static void main(String ...a){
int i,j,k;
Scanner sc= new Scanner(System.in);
System.out.print("enter the number of row for half diamond: ");
int n= sc.nextInt();
for(i=1;i<=n;i++)
{
for(k=1;k<=i;k++){
System.out.print("*");
}
System.out.println("");
}
for(i=n;i>1;i--)
{
for(k=i;k>1;k--){
System.out.print("*");
}
System.out.println("");
}
}
}
Output
enter the number of row for half diamond: 5
*
**
***
****
*****
****
***
**
*
2). Program to print left half diamond pattern using star(*) in Java
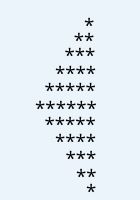
import java.util.*;
class Main{
public static void main(String ...a){
int i,j,k;
Scanner sc= new Scanner(System.in);
System.out.print("Enter the number of row for half diamond: ");
int n= sc.nextInt();
for(i=0;i<n;i++)
{
for(j=0;j<n-i;j++){
System.out.print(" ");
}
for(k=0;k<=i;k++){
System.out.print("*");
}
System.out.println("");
}
for(i=n-1;i>0;i--)
{
for(j=n;j>=i;j--){
System.out.print(" ");
}
for(k=i;k>0;k--){
System.out.print("*");
}
System.out.println("");
}
}
}
Output
Enter the number of row for half diamond: 5
*
**
***
****
*****
****
***
**
*
3). Program to print full diamond pattern using star(*) in Java
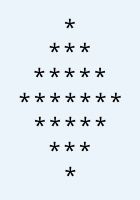
import java.util.*;
class Main{
public static void main(String ...a){
int i,j,k;
Scanner sc= new Scanner(System.in);
System.out.print("enter the number of row for full diamond: ");
int n= sc.nextInt();
for(i=0;i<n;i++)
{
for(j=0;j<n-i-1;j++){
System.out.print(" ");
}
for(k=0;k<2*i+1;k++){
System.out.print("*");
}
System.out.println("");
}
for(i=n-1;i>0;i--)
{
for(j=n-1;j>=i;j--){
System.out.print(" ");
}
for(k=2*i-1;k>0;k--){
System.out.print("*");
}
System.out.println("");
}
}
}
Output
enter the number of row for full diamond: 5
*
***
*****
*******
*********
*******
*****
***
*