Below are the various examples of pyramid patterns that are made using the combination of stars and the combination of numbers. This pyramid includes an inverted pyramid, full pyramid, left and right half pyramid, etc. And the program to print that pyramids are written in C programming language.
And below C concepts are used to print the patterns
- For Loop
- While Loop
- if..else
1). Program to print half pyramid pattern using star(*) in C
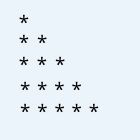
#include<stdio.h>
#include<string.h>
int main()
{
int i,j,n;
printf("Enter the number of rows for half pyramid: ");
scanf("%d",&n);
for(i=1;i<=n;i++){
for(j=1;j<=i;j++){
printf("*");
}
printf("\n");
}
return 0;
}
Output
Enter the number of rows for half pyramid: 5
*
**
***
****
*****
2). Program to print an Inverted half pyramid pattern using star(*) in C
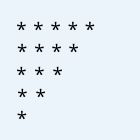
#include<stdio.h>
#include<string.h>
int main()
{
int i,j,n;
printf("Enter the number of rows for half pyramid: ");
scanf("%d",&n);
for(i=n;i>=1;i--){
for(j=i;j>=1;j--){
printf("*");
}
printf("\n");
}
return 0;
}
Output
Enter the number of rows for half pyramid: 5
*****
****
***
**
*
3). Program to print right half pyramid pattern using star(*) in C
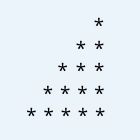
#include<stdio.h>
#include<string.h>
int main()
{
int i,j,n,k;
printf("Enter the number of rows for half pyramid: ");
scanf("%d",&n);
for(i=n;i>=1;i--){
for(j=i;j>=2;j--){
printf(" ");
}
for(k=1;k<=n-i+1;k++){
printf("*");
}
printf("\n");
}
return 0;
}
Output
Enter the number of rows for half pyramid: 5
*
**
***
****
*****
4). Program to print inverted right half pyramid pattern using star(*) in C
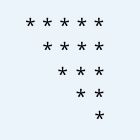
#include<stdio.h>
#include<string.h>
int main()
{
int i,j,n,k;
printf("Enter the no. of rows for Inv. half pyramid: ");
scanf("%d",&n);
for(i=n;i>=1;i--){
for(j=1;j<=n-i;j++){
printf(" ");
}
for(k=1;k<=i;k++){
printf("*");
}
printf("\n");
}
return 0;
}
Output
Enter the no. of rows for Inv. half pyramid: 5
*****
****
***
**
*
5). Program to print full pyramid pattern using star(*) in C
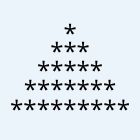
#include<stdio.h>
#include<string.h>
int main()
{
int i,j,n,k;
printf("Enter the number of rows for pyramid: ");
scanf("%d",&n);
for(i=n;i>=1;i--){
for(j=i;j>=2;j--){
printf(" ");
}
for(k=1;k<=2*(n-i+1)-1;k++){
printf("*");
}
printf("\n");
}
return 0;
}
Output
Enter the number of rows for pyramid: 5
*
***
*****
*******
*********
6). Program to print an inverted full pyramid pattern using star(*) in C
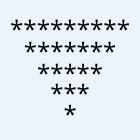
#include<stdio.h>
#include<string.h>
int main()
{
int i,j,n,k;
printf("Enter rows for Inv. Full Pyramid Pattern: ");
scanf("%d",&n);
for(i=n;i>=1;i--){
for(j=1;j<=n-i;j++){
printf(" ");
}
for(k=1;k<=2*i-1;k++){
printf("*");
}
printf("\n");
}
return 0;
}
Output
Enter rows for Inv. Full Pyramid Pattern: 5
*********
*******
*****
***
*
7). Program to print half pyramid pattern using numbers in C
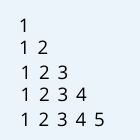
#include<stdio.h>
#include<string.h>
int main()
{
int i,j,n,k;
printf("Enter no. of rows for half pyramid: ");
scanf("%d",&n);
for(i=1;i<=n;i++){
for(j=1;j<=i;j++){
printf("%d",j);
}
printf("\n");
}
return 0;
}
Output
Enter no. of rows for half pyramid: 6
1
12
123
1234
12345
123456
8). Program to print inverted half pyramid pattern using numbers in C
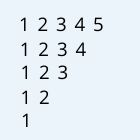
#include<stdio.h>
#include<string.h>
int main()
{
int i,j,n,k;
printf("Enter no. of rows for half pyramid: ");
scanf("%d",&n);
for(i=0;i<n;i++){
for(j=1;j<=n-i;j++){
printf("%d",j);
}
printf("\n");
}
return 0;
}
Output
Enter no. of rows for half pyramid: 5
12345
1234
123
12
1