Below are the various examples of diamond patterns that are made using the combination of stars. This diamond pattern includes full diamond, left and right half diamond, etc. And the program to print that diamonds are written in C programming language.
And below C concepts are used to print that patterns
- For Loop
- While Loop
- if..else
1). Program to print half pyramid pattern using star(*) in C
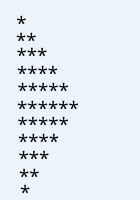
#include<stdio.h>
#include<conio.h>
int main(){
int i,j,k,n;
printf("Enter the number of row for half diamond:");
scanf("%d",&n);
for(i=1;i<=n;i++)
{
for(k=1;k<=i;k++){
printf("*");
}
printf("\n");
}
for(i=n;i>1;i--)
{
for(k=i;k>1;k--){
printf("*");
}
printf("\n");
}
return 0;
}
Output
Enter the number of row for half diamond:5
*
**
***
****
*****
****
***
**
*
2). Program to print left half diamond pattern using star(*) in C
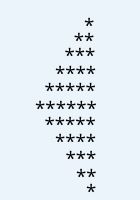
#include<stdio.h>
#include<conio.h>
int main(){
int i,j,k,n;
printf("enter the number of row for half diamond:");
scanf("%d",&n);
for(i=0;i<n;i++)
{
for(j=0;j<n-i;j++){
printf(" ");
}
for(k=0;k<=i;k++){
printf("*");
}
printf("\n");
}
for(i=n-1;i>0;i--)
{
for(j=n;j>=i;j--){
printf(" ");
}
for(k=i;k>0;k--){
printf("*");
}
printf("\n");
}
return 0;
}
Output
enter the number of row for half diamond:5
*
**
***
****
*****
****
***
**
*
3). Program to print full diamond pattern using star(*) in C
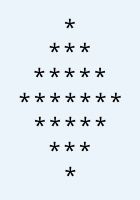
#include<stdio.h>
#include<conio.h>
int main(){
int i,j,k,n;
printf("enter the number of row for full diamond:");
scanf("%d",&n);
for(i=0;i<n;i++)
{
for(j=0;j<n-i-1;j++){
printf(" ");
}
for(k=0;k<2*i+1;k++){
printf("*");
}
printf("\n");
}
for(i=n-1;i>0;i--)
{
for(j=n-1;j>=i;j--){
printf(" ");
}
for(k=2*i-1;k>0;k--){
printf("*");
}
printf("\n");
}
return 0;
}
Output
enter the number of row for full diamond:5
*
***
*****
*******
*********
*******
*****
***
*