In this tutorial, you will learn writing program for how to remove duplicates from an array java.
Below are the approach which we will be follow to write our program:
- In this program first we will take an array and insert some elements in it.
- And then we will count the occurrence of each elements of an array.
- And print only those elements which has occurs only one times.
- And will not print those elements which has occurred more than one times.
How our program will behave?
Our program will take an array as an input.
And then we will count the occurrence of each elements. After the count it will print only those elements as an output which has occurs only one time.
Program to remove duplicate elements from array in Java
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the size of array: ");
int n = sc.nextInt();
int arr[] = new int[n];
int ar[] = new int[100];
Arrays.fill(ar,0);
System.out.println("Enter " +n+ " array elements between 0 to 100 ");
for(int i=0; i<n; i++) {
arr[i] = sc.nextInt();
}
System.out.println("Array elements after removing duplicates");
for(int i=0;i<n;i++){
ar[arr[i]]++;
if(ar[arr[i]]==1){
System.out.println(arr[i]);
}
}
}
}
Output:
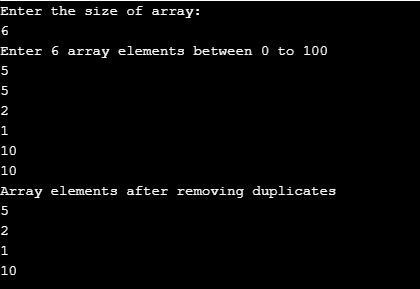