In this tutorial, you will learn how to write Java program to print the first duplicate number of an array.
This program is going to be very simple. Below are the approach which we will be follow to achieve our solution:
- In this program we have to check if any first number is duplicate then print that number and stop the program.
- To check it we will compare the one array element with the next element. If it matches then print it.
- We will use the concept of for loop and if-else statement to achieve our desired output.
How our program will behave?
As we have already seen above our logic to find the first duplicate number in Java.
Our program will take an array as an input.
And on the basis of inputs it will compare each elements with the next. If match found then print that number as a duplicate number otherwise go to next index and perform same operation.
Program to find first duplicate number in Java
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the size of array: ");
int n = sc.nextInt();
int arr[] = new int[n];
System.out.println("Enter " +n+ " array elements between 0 to "+(n-1));
for(int i=0; i<n; i++) {
arr[i] = sc.nextInt();
}
for(int i=0; i<n-1; i++) {
if(arr[i]==arr[i+1]){
System.out.println(arr[i] +" is first duplicate number");
break;
}
if(i==(n-2)){
System.out.println("No duplicate number");
}
}
}
}
Output:
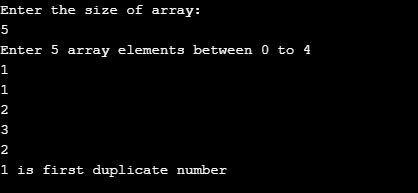