In this tutorial, we will learn to write a C program to print the given array in reverse order. To print the array in reverse order we will just start printing the array starting from the last index to index 0.
Here we are basically not reversing the array, We have to just print the array in reverse order.
How does print array in reverse order program work?
- Our program first ask the size of array. After giving the size it will ask for the array elements to insert.
- Now after taking the elements, We will start traversing the array from last index to index 0 using for loop.
- This is the all task we are going to perform.
C program to print array in reverse order
#include<stdio.h> int main() { int n, i; printf("enter the size of an array : "); scanf("%d",&n); int arr[n]; //To take the input in array for(i = 0; i < n; i++) { printf("Please give value for index %d : ",i); scanf("%d",&arr[i]); } printf("Array printing in reverse order is:\n"); //for loop to print array in reverse order for(i=n-1;i>=0;i--) { printf("%d\n",arr[i]); } return 0; }
Output
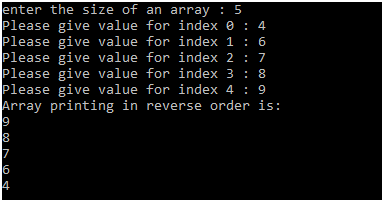
[wpusb]