To write a program in C to find sum of array elements, We will just iterate the array elements one by one using for loop or while loop and add each element. This program is very easy and logic is also very simple.
Program in C to find the sum of array elements
#include <stdio.h> int main() { int i, size, sum=0; printf("C Program to find the sum of array elements\n"); printf("First enter number of elements you want in Array\n"); scanf("%d", &size); int arr[size]; for(i = 0; i < size; i++) { printf("Please give value for index %d : ",i); scanf("%d",&arr[i]); } for (i = 0; i < size; i++) sum=sum+arr[i]; printf("Sum of array element=%d",sum); return 0; }
Output
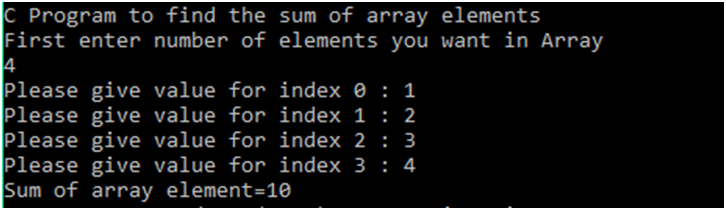
[wpusb]