In this tutorial, we are going to learn to write C Program to delete a given element from an array. To delete the given element we will compare the given element with the array elements. If we find a match then will delete the element from the array otherwise will check for the next array element. This process will continue till the end of the array.
Our problem statement
Suppose we have an array that has elements a={1,2,3,4,5}. We want to delete element 3 from the array. So after deleting element 3 from the array, the new array would be a={1,2,4,5}.
Here we are deleting the element from an array, not deleting the elements from the array index.
Our approach to to delete given element from array
- We will take the value in the array
- Now we will compare the element which we want to delete from array with all array elements.
- To iterate array we are using for loop in c and to compare elements we are using equal(==) operator and if statement.
- We have also handled the scenario, suppose if we have element that is repeated multiple times. We will delete the all occurrence as well.
C Program to delete given element from array
#include <stdio.h> #include <stdlib.h> int main() { int i, size, value,j,temp; printf("C Program to delete given element from array\n"); printf("Enter size of the array : "); scanf("%d", &size); int arr[size]; temp=size; for(i = 0; i < size; i++) { printf("Please give value for index %d : ",i); scanf("%d",&arr[i]); } printf("Enter the element to delete : "); scanf("%d", &value); for(i=0; i<size; i++) { if(arr[i]==value){ for(j=i; j<size-1; j++){ arr[j] = arr[j+1]; } size--; i--; ; } } if(temp==size){ printf("No element %d found in array ",value); exit(0); } printf("Rest elements of array after deleting %d are\n",value); for(i=0; i<size; i++) { printf("%d\n", arr[i]); } return 0; }
Output
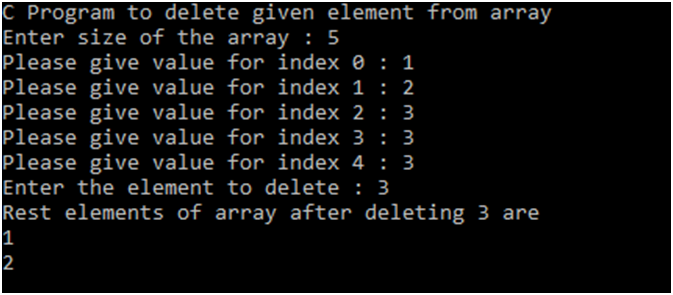
[wpusb]