In this tutorial you will learn how to write a program in C programming language to add two numbers without using addition operator.
Actually, It is not that much complex as it seems by visibility.
How our program will behave?
As if we give two numbers as an input like 25 and 20 then this program should return 45 as an output without using of an addition operator.
Program to add two numbers without using + operator
#include<stdio.h>
#include<conio.h>
int main() {
int num1, num2, i;
printf("please enter first number: ");
scanf("%d",&num1);
printf("please enter second number: ");
scanf("%d",&num2);
for(i=1;i<=num2;i++){
num1++;
}
printf("sum = %d", num1);
getch();
}
Output:
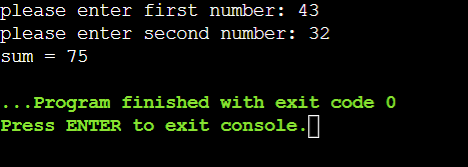
Explanation of the above program
- Above is a program to add two numbers without using addition operator.
- We have 3 variables num1, num2 and i.
- We will take input from user and store value in num1 and num2.
- Now we will increase of num1 using for loop. And for loop will call till the count of second number. And in each call we will increase value of num1 by 1.
- Following this approach we can easily calculate sum of two number.
- Final result will store in num1 after addition.
I hope it is now clear to you.