In this tutorial we are going to learn writing program in c to separate the characters in a given string. Basically separating means we are just going to print characters one by one from the given string.
How separate character C program will work?
- Our program will take a string as an input. Now we will iterate the string using for loop.
- In each iteration we will print one character at a time.
- This is the whole task we are going to perform in the program.
C Program to separate characters in String
#include <stdio.h> #include <string.h> int main() { char str[256]; int alpha=0, digit=0, specialChar = 0,i; printf("C program to separate character \n"); printf("Please Enter a String : "); scanf("%[^\n]", str); int len = strlen(str); printf("Printing Character of string\n"); for(i=0; i<len; i++) { printf("%c \n",str[i]); } return 0; }
Output
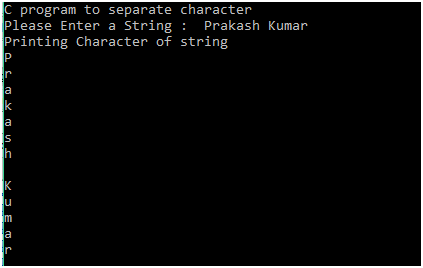
[wpusb]