In this tutorial, we will learn to write C program to insert an element at the given location in an array. To insert an element at a given location first we have to go to that location and then shift each element towards the right. Or we can also do something like shifting the elements towards right till we haven’t reached the location where we have to reach.
Programming logic to insert an element in array at given location
- Our program will take size of the array first and then elements to insert in the array.
- Now it will ask the new element and location where you want to insert in the array.
- To insert an element at a given location first we will shift the elements toward right by one position till we reach the location where we have to insert element.
- Once we reached at the location, then we will insert the element at the given location.
C Program to insert element at a given location in array
#include <stdio.h> void main() { int loc, i, n, value,ch; printf("C Program to insert element at end of Array\n"); printf("First enter number of elements you want in Array\n"); scanf("%d", &n); int arr[n]; for(i = 0; i < n; i++) { printf("Please give value for index %d : ",i); scanf("%d",&arr[i]); } printf("Please give a number to insert \n"); scanf("%d", &value); printf("Please enter the location to insert an new element\n"); scanf("%d", &loc); for (i = n - 1; i >= loc - 1; i--){ arr[i+1] = arr[i]; } arr[loc-1] = value; printf("After Insertion new Array is \n"); for (i = 0; i <= n; i++) printf("%d\n", arr[i]); }
Output
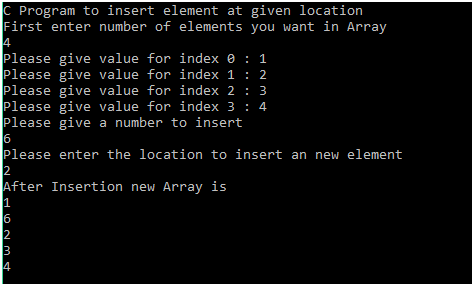
[wpusb]