An array is a collection of similar data types (like int, float, or char), which takes memory in a contiguous fashion in Primary memory locations.
We can declare an array in C as
int arr[50];
Where arr is an array with can hold 50 elements of integer types.
Two Type of array:
1). Single/One Dimensional array
2). Multi-Dimensional Array
1. One Dimensional Array
Declaration of one dimensional array:
An array can be declared with the bracket punctuators [ ], as shown in the below syntax:
Data_Type Array_Name[Number_Of_Elements]
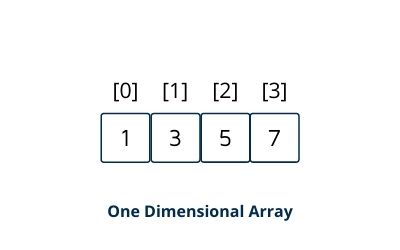
The below example shows a declaration of an array having the capacity to hold 50 elements of type integers and the name of that array is arr :
int arr[50];
Initializing the array
We can initialize an array in C by assigning value to each index one by one or by using a single statement as follows −
Example 1 :
Assign one value each time to the array
int arr[50];
arr[0]=12;
arr[1]= 43;
arr[2] = 14;
.
.
.
.
.
arr[49] = 54;
Example 2:
Using a single statement
int arr[5] = {10,32,11,45,38};
One Dimensional Array Program in C
#include<stdio.h>
int main () {
int arr[10];
int i,j;
for ( i = 0; i < 10; i++ ) {
arr[i] = i + 10;
}
for (j = 0; j < 10; j++ ) {
printf("arr[%d] = %d\n", j, arr[j] );
}
return 0;
}
Output
arr[0] = 10
arr[1] = 11
arr[2] = 12
arr[3] = 13
arr[4] = 14
arr[5] = 15
arr[6] = 16
arr[7] = 17
arr[8] = 18
arr[9] = 19
2. Multidimensional Arrays
We can say a Multidimensional array is an array of arrays. Two Dimensional arrays is also a multidimensional array. In a Multi-Dimensional array, elements of an array are arranged in the form of rows and columns.
Multidimensional array stores elements in tabular form which is also known as in row-major order or column-major order.
Declaration of Multi-dimensional Array
data_type array_name[size1][size2]....[sizeN];
data_type: It defines the type of data that can be held by an array. Here data_type can be int, char, float. etc.
array_name: Name of the array
size1, size2,… ,sizeN: Sizes of the dimensions
Two-dimensional array
Pictorial representation of a Two-dimensional array
number_of_arrays: number of arrays which flow in the z-axis
rows: number of the rows in the y-axis
columns: number of the columns flow in the x-axis
int arr1[10][20];
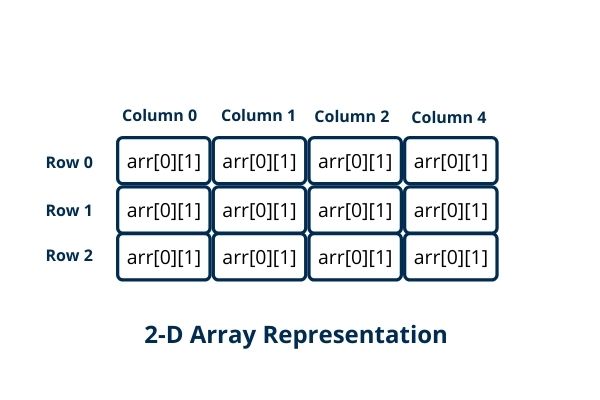
Two-dimensional array initialization
int arr[5][4]={
{1,2,3},
{2,3,4},
{3,4,5},
{4,5,6},
{5,6,7}
};
Two Dimensional Array Implementation in C
#include<stdio.h>
int main(){
int i=0,j=0;
int arr[5][4]={{1,2,3},{2,3,4},{3,4,5},{4,5,6},{5,6,7}};
for(i=0;i<5;i++){
for(j=0;j<4;j++){
printf("arr[%d] [%d] = %d \n",i,j,arr[i][j]);
}
}
return 0;
}
Output
arr[0] [0] = 1 arr[0] [1] = 2 arr[0] [2] = 3 arr[0] [3] = 0 arr[1] [0] = 2 arr[1] [1] = 3 arr[1] [2] = 4 arr[1] [3] = 0 arr[2] [0] = 3 arr[2] [1] = 4 arr[2] [2] = 5 arr[2] [3] = 0 arr[3] [0] = 4 arr[3] [1] = 5 arr[3] [2] = 6 arr[3] [3] = 0 arr[4] [0] = 5 arr[4] [1] = 6 arr[4] [2] = 7 arr[4] [3] = 0
Three dimensional array
Pictorial representation of a Three dimensional array
int arr2[10][20][30];
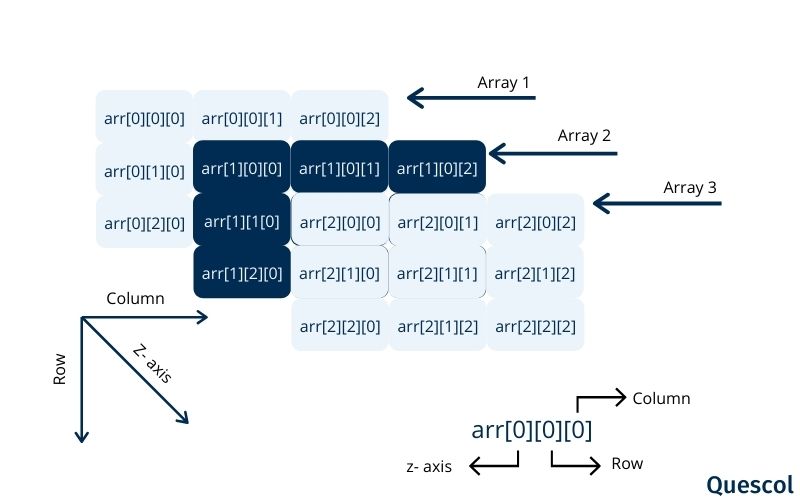
Three Dimensional Array array initialization
int arr[2][3][4] = {
{ {5, 6, 1, 9}, {10, -3, 91, 61}, {73, 32, 83, 22} },
{ {13, 43, 56, 36}, {25, 9, 93, 55}, {39, 21, 74, 6} }
};
Three-dimensional array Implementation in C
#include<stdio.h>
int main()
{
int i, j, k, arr[2][3][4];
printf("Enter 24 values for 3-D array: \n");
for(i = 0; i < 2; ++i) {
for (j = 0; j < 3; ++j) {
for(k = 0; k < 4; ++k ) {
scanf("%d", &arr[i][j][k]);
}
}
}
printf("\nDisplaying the values of 3-D array:\n");
for(i = 0; i < 2; ++i) {
for (j = 0; j < 3; ++j) {
for(k = 0; k < 4; ++k ) {
printf("arr[%d][%d][%d] = %d\n", i, j, k, arr[i][j][k]);
}
}
}
return 0;
}
Output
Enter 24 values for 3-D array: 1 2 3 4 5 6 7 8 9 1 2 3 4 5 6 7 8 9 12 3 4 5 6 7 Displaying the values of 3-D array: arr[0][0][0] = 1 arr[0][0][1] = 2 arr[0][0][2] = 3 arr[0][0][3] = 4 arr[0][1][0] = 5 arr[0][1][1] = 6 arr[0][1][2] = 7 arr[0][1][3] = 8 arr[0][2][0] = 9 arr[0][2][1] = 1 arr[0][2][2] = 2 arr[0][2][3] = 3 arr[1][0][0] = 4 arr[1][0][1] = 5 arr[1][0][2] = 6 arr[1][0][3] = 7 arr[1][1][0] = 8 arr[1][1][1] = 9 arr[1][1][2] = 12 arr[1][1][3] = 3 arr[1][2][0] = 4 arr[1][2][1] = 5 arr[1][2][2] = 6 arr[1][2][3] = 7