A Doubly Circular Linked List is a complex data structure that is formed with the combination of doubly linked list and circular linked list.
Definition
In a doubly circular linked list:
- Doubly Linked: Each node has two pointers, similar to a standard doubly linked list. One pointer points to the next node (
next
), and the other points to the previous node (prev
). - Circular: Unlike a standard doubly linked list, the last node’s
next
pointer doesn’t point toNULL
. Instead, it points back to the first node of the list. Similarly, the first node’sprev
pointer doesn’t point toNULL
but to the last node, forming a circular structure.
Node Structure Of Doubly Circular Linked List
A node in a doubly circular linked list typically contains the following components:
- Data: This part of the node stores the actual value or information that the node is supposed to hold. It can be of any data type like
int
,char
,float
, or even a user-defined data type. - Next Pointer: It points to the next node in the list. In the case of the last node, this pointer points back to the first node, making the list circular.
- Previous Pointer: It points to the previous node in the list. In the case of the first node, this pointer points to the last node, again ensuring the circular nature of the list.
Visual Representation Of Doubly Circular Linked List
A simple representation of a node in a doubly circular linked list can be visualized as:
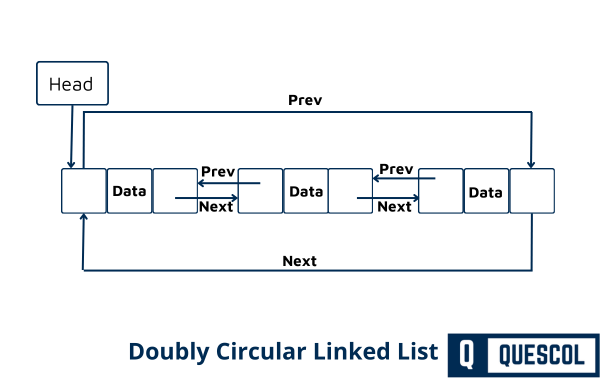
Here, each rectangular block represents a node with three parts: Prev
, Data
, and Next
. The arrows indicate the direction of the pointers, creating a circular loop.
Characteristics Of Doubly Circular Linked List
- No Null Pointers: There are no
NULL
pointers fornext
andprev
pointers in any of the nodes. Each node is always connected in both directions. - Traversal: The list can be traversed from any node in both forward and backward directions indefinitely, as it loops back onto itself.
- Flexibility: It inherits the flexibility of doubly linked lists in terms of insertion and deletion of nodes but adds the characteristic of easy circular traversal.
Advantages of Doubly Circular Linked Lists
Doubly Circular Linked Lists offer several advantages that make them suitable for specific applications:
- Two-Way Navigation: These lists allow traversal in both directions – forward and backward. This is particularly useful in scenarios where you might need to iterate over data in reverse order without needing to traverse from the beginning.
- Circular Nature: The circular nature facilitates going around the list without needing to find the end or beginning. This is beneficial in scenarios where you need to repeatedly cycle through the list.
- No Boundary Conditions: Unlike standard linked lists, there’s no need to check for
NULL
pointers, which simplifies certain operations. This eliminates the need for edge case handling for the start and end of the list. - Efficient Insertions and Deletions: Just like doubly linked lists, these structures allow for relatively efficient insertions and deletions, not just at the ends but also in the middle of the list.
- Dynamic Size: The list size is dynamic, making it flexible to grow or shrink as needed. This is advantageous over static data structures like arrays.
- Consistent Insertion and Deletion Time: Insertion and deletion operations have a consistent time complexity, irrespective of the position in the list.
Applications of Doubly Circular Linked Lists
Doubly Circular Linked Lists find their use in various applications where their unique properties are beneficial:
- Computer Applications:
- Music Players: For playlists where the songs cycle – when the end of the playlist is reached, it loops back to the beginning.
- Image Slideshow Applications: To cycle through images forward and backward without interruption.
- Gaming:
- Used in games that require circular traversal of elements, like board games where pieces move in a loop.
- Operating System:
- For managing resources in a circular fashion, like scheduling algorithms where processes are handled in a round-robin manner.
- Data Processing:
- In applications where data needs to be processed in a loop without a defined start or end.
- Browser Cache:
- Managing web pages in a cache where older entries are overwritten in a circular manner.
- Networking:
- In network applications for token ring protocols, where a token is passed in a network loop to control access.
- Multi-tasking in Embedded Systems:
- For task scheduling where the control needs to cycle through different tasks continuously.
The doubly circular linked list’s flexibility for bidirectional traversal and its circular nature make it a valuable tool in scenarios that require continuous looping and efficient element access in both directions.