In this tutorial we will learn about the doubly linked list. There is some changes done in singly linked list to build doubly linked list. In singly linked lists, where each element/node points to the next element/node. But in doubly linked list, each elements points to its next node along with the previous node. In the world of programming and data structures doubly linked list is also a way to store and manipulate the data.
In this tutorial, we’ll explore what doubly linked lists are, and also will explore their structure, and understand their advantages and applications. This complete tutorial will be very helpful for beginners to understand the concept and write answers during interviews and semester exams.
What is a Doubly Linked List?
A doubly linked list is an advanced type of linked list where each node is interconnected in both directions. Unlike a singly linked list, where each node only holds a reference to the next node, in a doubly linked list each node contain two references – one to the next node and another to the previous one. This dual-linking offers a significant advantage in navigating through the list, as it allows movement in both forward and backward directions.
This distinction is crucial when comparing it to singly linked lists. In a singly linked list, if you need to traverse backward, you would have to start from the beginning and move forward until you reach the desired node. However, in a doubly linked list, you can move directly to the previous node without this limitation, making certain operations more efficient.
Node Structure of Doubly Linked Lists
Each node in a doubly linked list typically contains three components:
- Data: This part of the node stores the value or the information that the node is supposed to hold.
- Next Pointer: It points to the next node in the list. In the last node of the list, this pointer is null, indicating the end of the list.
- Previous Pointer: It points to the previous node in the list. Similarly, in the first node, this pointer is null, indicating the start of the list.
The structure can be visually represented as follows:
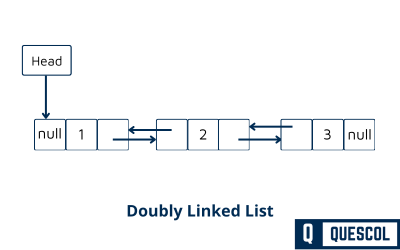
Advantages of Doubly Linked Lists
Doubly linked lists are a smart choice for many tasks because of their unique features. Here are some of their key benefits:
- Two-Way Navigation: The biggest benefit of doubly linked lists is moving both ways through the list – forward and back. This is really handy for things like flipping through songs in a playlist or moving through pages in a document.
- Easy Add and Remove: Adding or removing items is simpler in doubly linked lists compared to singly linked lists. In singly linked lists, you often have to start from the beginning to find the right spot. But with doubly linked lists, each item knows about the one before it, making these tasks quicker and easier, especially in the middle of the list.
- No Backtracking Needed: In doubly linked lists, each item keeps a link to the one before it. This means you don’t need extra steps to keep track of where you’ve been, a common need in singly linked lists.
- Flexible for More Uses: These lists are really adaptable. They’re great for building complex structures, like queues where you might add or take away items from either end.
Applications of Doubly Linked Lists
Doubly linked lists are really useful in many areas:
- In Navigation Systems: They’re great for things like web browser history or in document editors. Here, you often need to go back and forth between different pages or states, and doubly linked lists make this easy.
- For Undo Features: Many programs, like word processors or design software, use these lists to manage undo and redo actions. Each change is a new item in the list, making it simple to move through your edit history.
- In Playlists and Galleries: They’re also used in apps where you need to move through items, like music playlists or photo galleries. Their ability to go both ways makes browsing through these items a breeze.
- In Gaming: Games often use them too. For example, in managing inventories or game elements that change a lot, like adding or removing items as you play.
Conclusion
Doubly linked lists stand out as a dynamic and flexible data structure. They excel in navigation and adaptability, outshining singly linked lists and arrays. Their two-way traversal capability and the ease with which they handle adding and removing elements make them a top choice for many software development tasks. For beginners, getting to know doubly linked lists – how they’re structured and used – is a key step in mastering essential data structures. These structures are crucial for solving problems effectively in programming.