What is a Circular linked list?
A circular linked is very similar to a singly linked list, the only difference is in the singly linked list the last node of the list point to null but in the circular linked list the last node of the list point to the address of the head. This type of list is known as a circular linked list.
We can traverse all nodes starting from the head and stop when the next node is pointing to the head which indicates we have reached the last node.
Below is the figure of how circular linked list looks
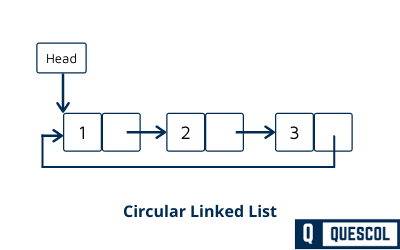
While traversing the circular linked list, we have to break the loop if we find the next of the last node is equal to the head other we can go into an infinite loop.
Advantages of Circular Linked Lists
- Efficient Circular Operations: Imagine a race track; cars can keep lapping without ever needing to stop. Circular Linked Lists let information be processed in a loop, perfect for cyclic tasks.
- Easy Insertion and Deletion: Adding or removing items is like inserting or removing a piece from a puzzle; it can be done anywhere with minimal adjustment.
- No Need for Backtracking: To revisit items, you don’t need to turn around; just keep going, and you’ll get back to where you started.
Disadvantages of Circular Linked Lists
- Complexity in Traversal: Knowing when to stop is tricky since it loops forever. Special conditions are needed to break the loop.
- Error Prone: A small mistake in connecting the last item back to the first can break the loop, making it hard to navigate.
Applications of Circular Linked Lists
- Computer Applications:
- Task Scheduling: Operating systems use circular lists to manage tasks that need constant attention, like checking if user input is received or if an app needs updating.
- Memory Management: Some algorithms use circular lists to keep track of memory slots in use and available.
- Games:
- Many multiplayer board games use circular linked lists to manage player turns, ensuring everyone gets their turn in a loop without needing complex tracking systems.
- Applications Requiring Cyclic Operations:
- Music Playlists: Music players use circular lists to loop playlists or repeat a single song.
- Digital Signage: Advertisements displayed on digital billboards often loop through a set of images or messages using a structure similar to circular linked lists.
Real-World Example of Circular Linked List
A real-world example of a circular linked list is the use of a carousel at an airport baggage claim. Imagine each piece of luggage on the carousel as a node in a circular linked list. The carousel continuously moves in a loop, allowing passengers to pick up their luggage. Just like in a circular linked list, where you can traverse from the last node back to the first seamlessly, the luggage on the carousel moves in a circular path, making it accessible to passengers again if they missed it the first time.
This system efficiently manages the collection of items (in this case, luggage) in a cyclic manner, ensuring that passengers have continuous access to their baggage’s without the need for the luggage to be redirected or for passengers to move to a different location to collect missed items.
Conclusion
Circular Linked Lists offer a unique way to store and manage data, especially for tasks that naturally loop or cycle. While they can be a bit more complex to set up and manage, their advantages make them invaluable for specific applications where their circular nature and efficient insertions and deletions play a critical role. Whether in computing, gaming, or everyday applications, these lists demonstrate the power of data structures in making our digital and real-world experiences smoother and more efficient.