In this tutorial, we are going to learn a python program to find the factorial of the number given as input from the user.
Problem Statement
For any numbers that are input by the user, we have to calculate the factorial of that numbers.
For example:
Case1: If the user input the 5.
then the output should be 120 ( 1*2*3*4*5= 120).
Case2: If the user input the 6.
then the output should be 720 (1*2*3*4*5*6 =720).
Factorial of a number
The factorial of a number is the multiplication of each and every number till the given number except 0.
For example:
Case1: factorial of number 4 is the multiplication of every number till number 4, i.e. 1*2*3*4
Case 2: factorial of number 3 is the multiplication of every number till number 3, i.e. 1*2*3
NOTE:
- The factorial of 0 is 1
- The factorial of any negative number is not defined.
- The factorial only exits for whole numbers.
Let’s discuss some more about functions that we are going to use in our program.
Python functions used in our program
Range() function
It returns the sequence of a given number between a given range. Range() function is a Python built-in function that is used when the user needs to perform an action-specific number of times. It is commonly used with looping statements.
It takes 3 arguments
1. start: it is theinteger starting from which the series of integers is to be returned.
2. stop: it is the integer earlier than which the collection of integers is to be lower back.
3. step: it is the integer that determines the increment among each integer within the collection.
‘for’ loop Statement
The ‘for loop’ is a looping statement in python which is used to iterate over a sequence or any other iterable objects such as lists, tuples, dictionaries, strings, etc.
Flow Chart for ‘for loop’
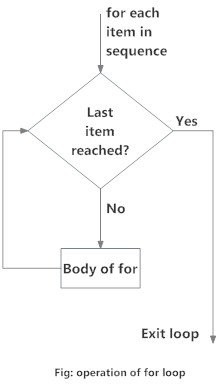
- There can be any number of ‘elif’ block but there must be a condition assigned for each and every ‘elif’ statement.
- There must be a ‘if’ statement with a condition assigned and there must not be more than 1 ‘if’ statement.
- ‘else’ statement is optional.
Our logic to calculate the factorial
- Our program will take an integer input from the user which should be a whole number.
- If the number input is negative then print ‘The factorial can’t be calculated’.
- Then use for loop with range function to define the sequence of numbers which we will use to calculate the factorial.
- After that, we will store the value of the factorial in a variable and finally print the value of the variable.
Algorithm to calculate the factorial
Step 1: Start
Step 2: take input from the user for finding the factorial.
Step 3: Create a variable ‘factorial’ and assign the value 1.
Step 4: if(number<0):
print ‘cannot be calculated.
elif ( number == 1):
print 1
else:
for i in range(1, number+1):
factorial*=i
Step 5: print factorial
Step 6: Stop
Python Code to calculate the factorial
Output 1:

Explanation:
The input number from the user to find the factorial is 5. The calculated value of the factorial is 1*2*3*4*5.
Output 2:

Explanation:
The input number from the user to find the factorial is 8. The calculated value of the factorial is 1*2*3*4*5*6*7*8.