#include<stdio.h> int main() { int size1, size2, newSize, i, j; printf("C Program to merge two arrays \n"); printf("Enter the number of elements for First Array : \n"); scanf("%d", &size1); int arr1[size1]; printf("Enter the elements for First Array : \n "); for(i = 0; i < size1; i++) { scanf("%d", &arr1[i]); } printf("Enter the number of elements for Second Array : \n"); scanf("%d", &size2); //Array Size Declaration int arr2[size2]; //Array Declaration printf("Enter the elements for Second Array : \n"); for(i = 0; i < size2; i++) { scanf("%d", &arr2[i]); } newSize = size1 + size2; int newArr[newSize]; for(i = 0; i < size1; i++) { newArr[i] = arr1[i]; } for(i = 0, j = size1; j < newSize && i < size2; i++, j++) { newArr[j] = arr2[i]; } printf("New Array Elements After Merging \n", newSize); for(i = 0; i < newSize; i++) { printf(" %d \t ",newArr[i]); } return 0; }
Output
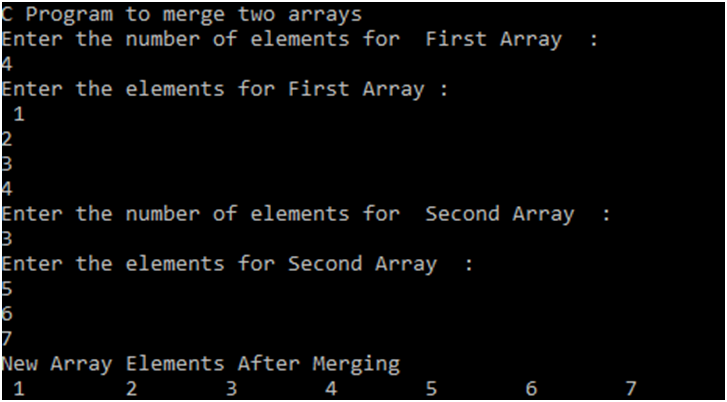
[wpusb]
Also Prepare Below Important Question
- Sum of digits of Given Number in Java
- Hibernate Interview Questions for 2+ years of experience
- 68 Most Important Microservices Interview Questions
- 60 Most Important Git Interview Questions
- 50+ Mostly asked Java Interview Questions for 6 Years Exp.
- 60+ Mostly Asked Java Interview Questions for 4 Years Exp
- 60+ Mostly asked Java Interview Question for 3+ Years
- 60+ Spring Boot interview questions for 4+ years Exp.
- 60+ Mostly Asked Spring Boot Interview Questions for 3+ Yrs
- Scenario Based Java 8 Coding Interview Questions (For Experienced)
- Python Program to add two numbers without addition operator
- Mostly Asked Java Interview Questions For 2 Yrs Experience
- Find All Pairs in Array whose Sum is Equal to given number Using Java
- Java Program to find GCD of two Numbers using Recursion
- Python Program to Separate Characters in a Given String
- Python Program to add two number using Recursion
- Python Program to Find Highest Frequency Element in Array
- Python Program to Merge two Arrays
- Perform left rotation by two positions in Array Using Python
- Python Program to Delete Element at Given Index in Array
Interview Questions Categories
C Programming Interview Preparation
Core Java Programming Interview Preparation
- Core Java Programming Coding Questions
- Core Java Pattern Programming Questions
- Core Java Programming Interview Questions