linked list insertion program in C at beginning
[c]
#include<conio.h>
#include<stdio.h>
#include<stdlib.h>
struct node{
int data;
struct node *next;
};
struct node *head=NULL;
struct node* createNode(){
struct node *newNode = (struct node *)malloc(sizeof(struct node));
return (newNode);
}
void insertNodeAtBegin(){
struct node *newNode, *temp;
newNode=createNode();
printf("enter the data you want to insert at begining in the list:");
scanf("%d",&newNode->data);
if(head==NULL){
head=newNode;
}
else{
temp=head;
head=newNode;
head->next=temp;
}
}
void viewList(){
struct node* temp=head;
if(temp==NULL){
printf("list is empty");
}
else{
while(temp->next!=NULL)
{
printf("%d\t",temp->data);
temp=temp->next;
}
printf("%d \t",temp->data);
}
}
int menu(){
int choice;
printf("\n 1. Give value to insert at begining");
printf("\n 2. Travesre/View List");
printf("\n 3. exit");
printf("\n Please enter your choice: \t");
scanf("%d",&choice);
return(choice);
}
void main(){
while(1){
switch(menu()){
case 1:
insertNodeAtBegin();
break;
case 2:
viewList();
break;
case 3:
exit(0);
default:
printf("invalid choice");
}
getch();
}
}
[/c]
Output:
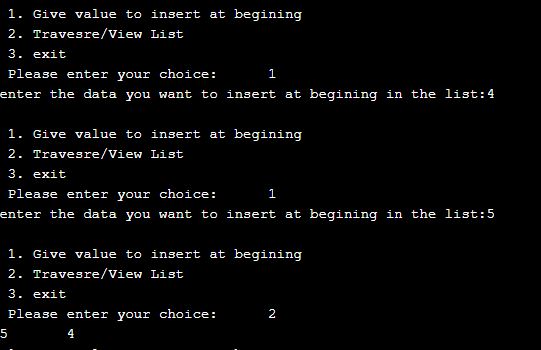