In this tutorial, you will learn how to write Java program to find the largest two numbers in a given array.
Below are the approach which we will be follow to write our program:
- In this program first we will take an array and take some elements as an input from users.
- Then we will iterate the loop and then will compare elements till the and during comparison we will find the greatest two elements in a given array.
- You can also follow a approach like first short an array in ascending order and then select top two distinct elements of an array.
How our program will behave?
As we have already seen above our logic to find the first two maximum number of a given array.
Our program will take an array as an input.
And on the basis of inputs it will compare each elements and on the basis of comparison it will print two greatest elements.
Java Program to find two largest number in an array
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the size of array: ");
int n = sc.nextInt();
int arr[] = new int[n];
System.out.println("Enter " +n+ " array elements ");
for(int i=0; i<n; i++) {
arr[i] = sc.nextInt();
}
Arrays.sort(arr);
for(int i=n-1;i>1;i--){
if(arr[i]!=arr[i-1]){
System.out.println(arr[i] + " and "+arr[i-1]+" is two maximum elements in array");
break;
}
}
}
}
Output:
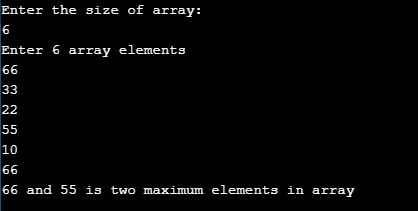