Below Java concepts are used to print that patterns
- For Loop
- While Loop
- if..else
1). Program to print Solid Rectangle using Star(*) in Java
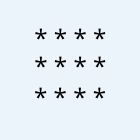
import java.util.*;
class Main{
public static void main(String ...a){
int i,j;
System.out.println("This is Rectangle program");
Scanner sc= new Scanner(System.in);
System.out.print("enter the number of rows: ");
int rows= sc.nextInt();
Scanner sc1= new Scanner(System.in);
System.out.print("enter the number of columns: ");
int columns= sc1.nextInt();
for(i=1; i<=rows; i++)
{
for(j=1; j<=columns; j++)
{
System.out.print("*");
}
System.out.println("");
}
}
}
Output
This is Rectangle program
enter the number of rows: 3
enter the number of columns: 5
*****
*****
*****
2). Program to print hollow Rectangle using star(*) in Java
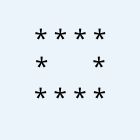
import java.util.*;
class Main{
public static void main(String ...a){
int i,j;
System.out.println("Hollow Reactangle Program");
Scanner sc= new Scanner(System.in);
System.out.print("enter the number of rows: ");
int rows= sc.nextInt();
Scanner sc1= new Scanner(System.in);
System.out.print("enter the number of columns: ");
int columns= sc1.nextInt();
for(i=1; i<=rows; i++)
{
for(j=1; j<=columns; j++)
{
if(i==1 || i==rows || j==1 || j==columns)
{
System.out.print("*");
}
else
{
System.out.print(" ");
}
}
System.out.println("");
}
}
}
Output
Hollow Reactangle Program
enter the number of rows: 4
enter the number of columns: 8
********
* *
* *
********
What did you think?
Similar Reads
-
Find middle element of a linked list in single pass
Linked lists are a fundamental data structure used in computer science for organizing and managing data. A linked list is… -
Python Program to Find Last 3rd element in Singly Linked List
In this tutorial, we are going to learn the writing python program to Find 3rd element of Linked List from… -
Most important JavaScript Interview Questions To Prepare
In this Page we have collected and explained Most important Javascript Interview Questions and Answers for begineers, freshers as well… -
Sum of digits of Given Number in Java
In this tutorial we will learn writing Java program to calculate the sum of its digit. We will also see… -
Hibernate Interview Questions for 2+ years of experience
Certainly! Here's a list of commonly asked interview questions on Hibernate for candidates with 2+ years of experience: Basic Hibernate… -
68 Most Important Microservices Interview Questions
Certainly, here's an extended list of 50 commonly asked interview questions on microservices for candidates with 2+ years of experience:… -
60 Most Important Git Interview Questions
Certainly! Here is a list of commonly asked interview questions on Git for candidates with fresher or having of experience… -
50+ Most important Java Interview Questions for 5+ Years Exp
1. Explain the SOLID principles in Java. Provide examples of how you have applied these principles in your projects. SOLID… -
60+ Spring Boot interview questions for 4+ years Exp.
1. What is Spring Boot and how does it differ from the Spring framework? Spring Boot is a framework designed… -
60+ Mostly Asked Spring Boot Interview Questions for 3+ Yrs
Here is a list of 60+ Spring Boot interview questions for candidates with 3+ years of experience: 1. What is…