In this tutorial, we are going to learn writing python program to find the factorial of the number using recursion method. We will take a number from the users as and input and our program will print the factorial as an output.
Problem Statement
For any numbers that are input by the user, we have to calculate the factorial of that numbers.
For example
Case1: If the user input the 5.
then the output should be 120 ( 1*2*3*4*5= 120).
Case2: If the user input the 6.
then the output should be 720 (1*2*3*4*5*6 =720).
What is Factorial of a number?
The factorial of a number is the multiplication of each and every number till the given number except 0.
For example:
Case 1: factorial of number 4 is the multiplication of every number till number 4, i.e. 1*2*3*4
Case 2: factorial of number 3 is the multiplication of every number till number 3, i.e. 1*2*3
NOTE:
- The factorial of 0 is 1
- The factorial of any negative number is not defined.
- The factorial only exits for whole numbers.
What is recursion?
Recursion is a method that defines something in terms of itself, which means it is a process in which a function calls itself. A complete function can be split down into various sub-parts in recursion. It makes code look simple and compact. It is also defined as the process of defining a program or problem in terms of itself.
Let’s have a look at the simple diagram that explains the working of recursion.
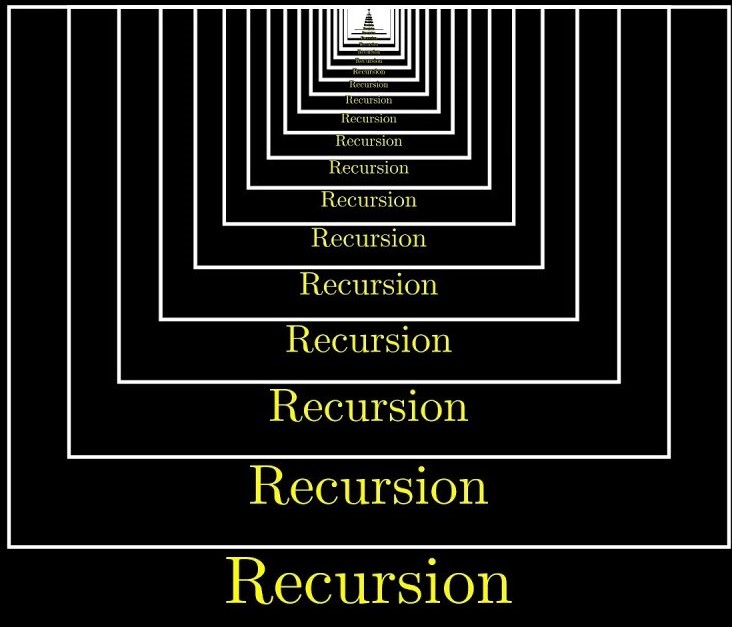
Our logic to find factorial of a number using recursion
- Our program will take an integer input from the user which should be a whole number.
- If the number input is negative then print ‘The factorial can’t be calculated’.
- Then use fact(num) user-defined recursive function that will return the factorial of the given number i.e. num.
Let’s understand through a diagram how our recursive function will work on input 7.
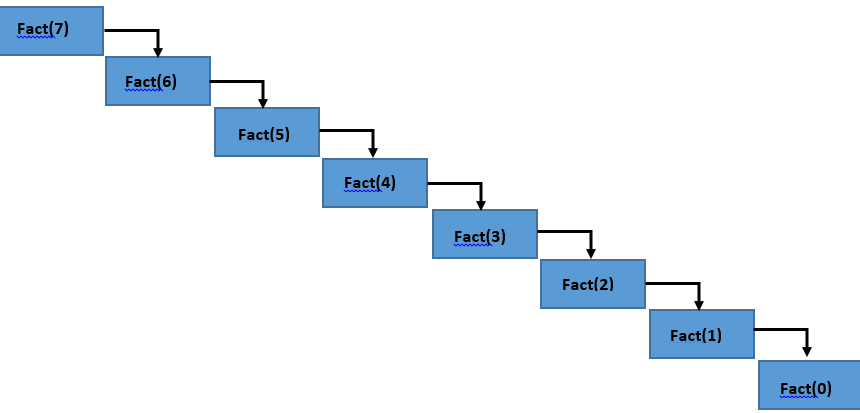
Python Program to find factorial of a number using recursion
def fact(n):
if n == 1:
return n
else:
#recursion
return n*fact(n-1)
num = int(input("Enter a whole number to find Factorial: "))
if num < 0:
print("Factorial can't be calculated for negative number")
elif num == 0:
print("Factorial of 0 is 1")
else:
print("Factorial of",num,"is",fact(num))
Output 1:
Enter a whole number to find Factorial: 5
Factorial of 5 is 120
Explanation:
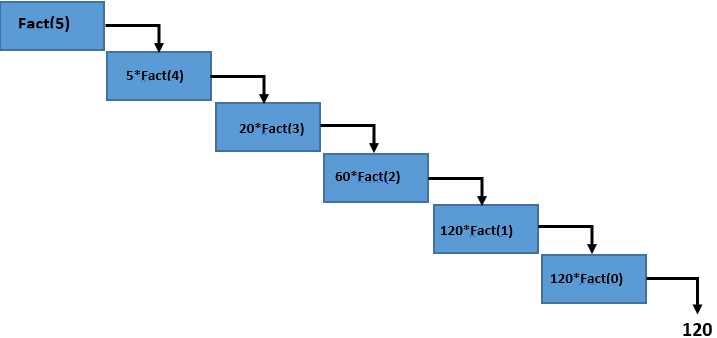
Output 2:
Enter a whole number to find Factorial: 6
Factorial of 6 is 720
Explanation:
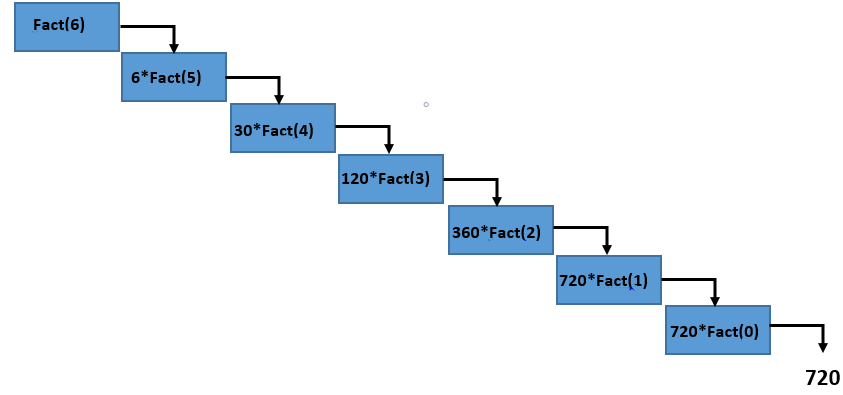