In this tutorial, you will learn writing a program, how to find the missing number in integer array of 1 to 100 in C programming language.
The Complete logic behind to find missing number in array is :
- As we know that the formula (n*(n+1))/2 to add a number series.
- where n is a number upto you want to add.
- Suppose you want to add number 1 to 10 then replace n with 10 and you will easily get the sum of 1 to 10.
- Same formula will be apply for to sum 1 to 100.
- Now we have to find the missing number between 1 to 100. So we will subtract the sum of given number by user from the sum of 1 to 100.
- For example: Here I am taking a small for 1 to 10. Sum of numbers from 1 to 10 is 55. and someone have entered number and their sum is 45.
- Now when subtract 55-45 = 10, means 10 is the missing number which is not entered by user.
How our program will behave?
As we have already seen our logic above.
This C program will take size of array and numbers as input from the user.
Then our program will add the number which is given by the users. and after addition subtract it with the sum of the number from 1 to n (where n is number given by user as size of array).
And after subtraction it will give the output as this is the missing number.
Program to find missing number in array in c
#include<stdio.h>
#include<conio.h>
void main(){
int i,n,sum=0,missing;
printf("enter the size of an array : ");
scanf("%d",&n);
int arr[n-1];
printf("please give value to insert in array: n");
for(i=0;i<n-1;i++){
scanf("%d",&arr[i]);
sum=sum+arr[i];
}
missing = (n*(n+1))/2 - sum;
printf("missing number is = %d", missing);
getch();
}
Output:
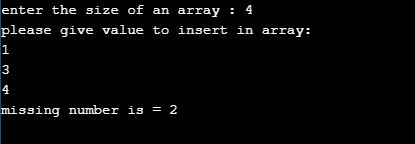
Explanation of above program to find missing number in array in c
- Our Program is very simple.
- We have 4 integer variables in above i, n, sum, and missing. And one array of integer type which name is arr.
- In this program we will ask to user to give the size of array as an input which will be total number of elements in array.
- Then we will ask to given the element in array for each index of the array which logic is written in for loop.
- In our logic, if user gives 10 as array size then user are allowed to insert only 9 elements in that array so that 1 element is missing and we will calculate it.
- In the for loop we are taking a input from a user and at the same time adding it and storing it in sum variable.
- And at last missing variable will store the missing value of the array after subtracting whole sum of the number from 1 to n with total sum of input elements by user.
- This program is only to find single missing element of an array.
This was the all logic behind to find missing number in an array.
I hope it has helped you to understand this concept.