In this tutorial, you will learn how to write Java program to find largest and smallest number in an array.
Below are the approach which we will be follow to write our program:
- At first we will take inputs from the users in array.
- Then we select first element as a largest as well as smallest. It is an assumptions.
- Now we will traverse each elements of an array and compare with largest one (which we have selected first element).
- Now if we found largest element then we will replace old largest value with new one and follow the same process until we traverse all elements of an array.
- And for the smallest one we follow the same approach. If we found next smallest then we will replace it from old one and follow the same approach until we traverse all array elements.
How our program will behave?
Our program will take one array and on the basis of logic it will print greatest and smallest number of an array.
Suppose if we give an array as 34, 22, 1, 90, 114, 87
Then our program should give output as:
Largest number is 114
Smallest number is 1
Java Program to find largest and smallest number in an array
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the size of array: ");
int n = sc.nextInt();
int arr[] = new int[n];
System.out.println("Enter " +n+ " array elements ");
for(int i=0; i<n; i++) {
arr[i] = sc.nextInt();
}
int largest = arr[0];
int smallest = arr[0];
for(int i=1;i<n;i++){
if(arr[i]>largest){
largest =arr[i];
}
if(arr[i]<smallest){
smallest = arr[i];
}
}
System.out.println(largest+" is largest and "+ smallest +" is smallest");
}
}
Output:
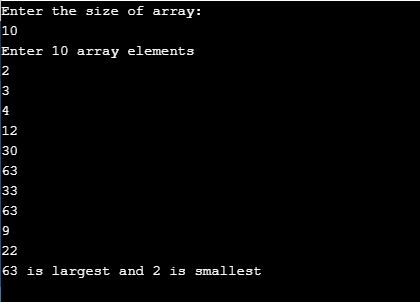