In this tutorial, We will learn writing java program to delete a given element of an Array and print the updated Array. Our program will delete the given element (which is specified by the user) from the array.
For Example
Case 1: if the given array is {1, 2, 3, 4} and the user gives input 3 to remove.
The output should be {1, 2, 4}.
Case 2: if the given array is {9, 2, 4, 8} and the user gives input 4 to remove.
The output should be {9, 2, 8}.
Java Program to Delete a given Element of Array
Output
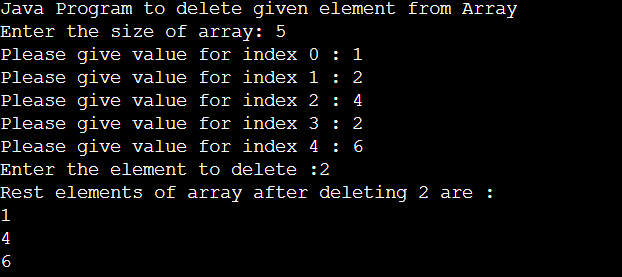
Explanation
For the input array [1, 2, 4, 2, 6], and element to be delete is 2 from the array. Our program first iterate through the array element to find 2, once 2 has been found it, it removes by shifting all elements (which comes after 2), to one left. So here after deleting 2 our array become [1,4,6]