In this tutorial, you will learn how to write C program to print the first duplicate number of an array.
This program is going to be very simple. Below are the approach which we will be follow to achieve our solution:
- In this program we have to check if any first number is duplicate then print that number and stop the program.
- To check it we will compare the one array element with the next element. If it matches then print it.
- We will use the concept of for loop and if-else statement to achieve our desired output.
How our program will behave?
As we have already seen above our logic to find the first duplicate number in C.
Our program will take an array as an input.
And on the basis of inputs it will compare each elements with the next. If match found then print that number as a duplicate number otherwise go to next index and perform same operation.
Program to find first duplicate number in C
#include <stdio.h>
#include<stdlib.h>
#define MAX 100
void duplicate(int *arr, int size)
{
int i, temp,j;
int ar[MAX] = {0};
for(i=0;i<size;i++){
for(j=i+1;j<size;j++){
if(arr[i]==arr[j]){
printf("first duplicate number is = %d",arr[i]);
exit(0);
}
}
}
}
int main()
{
int size, i, sum, *arr;
printf("Enter number of Elements in an array n");
scanf("%d", &size);
arr = (int *) malloc(sizeof(int) * size);
printf("Enter elements to arrayn");
for(i = 0; i < size; i++)
scanf("%d", &arr[i]);
duplicate(arr, size);
return 0;
}
Output:
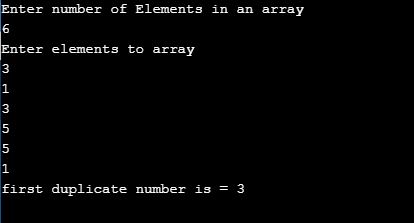
Explanation of above program for finding first duplicate number in array
- In the above program we have taken three int variable size, i and sum, and one integer pointer variable arr.
- size variable will take the size of array given by user and arr will be array which size is calculated by malloc function dynamically at run time.
- Now value will be assigned to each index of an array which is given by user.
- We have a function called duplicate which will take array and size of array as an input.
- And then it will find the duplicate element in an array and then print the duplicate value.
- In the body of duplicate function we have logic to compare the first element of the array with the next elements. If any matches found then print that element otherwise compare the next element with the rest all elements.
- For that comparison we have two for loop. One loop will have one element and next for loop will help to compare it with next elements.
This was the all logic behind checking the given two strings are anagram or not.
If any doubt just do comment 🙂
Thank you.