In this tutorial, we will learn to write the program to find the factorial of numbers using the C programming language. Here we are going to follow a recursive approach.
What is Factorial of a number?
Factorial of a whole number ‘n’ is the product of that number ‘n’ with its every whole number in descending order till 1.
For example
Factorial of 5 is 5*4*3*2*1 = 120
And factorial of 5 can be written as 5!.
Factorial Program in C using Recursion
#include<stdio.h>
long long fact(int num) {
if (num == 0)
return 1;
else
return (num * fact(num - 1));
}
int main() {
int i, num, factorial = 1;
printf("Enter a whole number to find Factorial = ");
scanf("%d", & num);
printf("Factorial of %d is: %llu", num, fact(num));
return 0;
}
Output
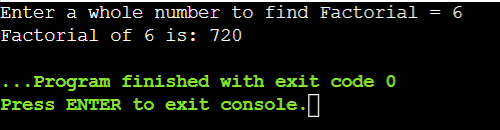