A stack is a linear data structure in which the elements are added and removed only from one end, which is called the TOP. Hence, a stack is also called a LIFO (Last-In-First-Out) or FILO(First In Last Out) data structure, which means the element that was inserted last is the first one to be taken out and the element that was inserted First is the last one to be taken out.
For example, In a pile of plates where one plate is placed on top of another plate. Now, when you want to remove a plate from the pile of plates, first you remove the topmost plate. Similarly in Stack, you can remove elements only from the top.
Example of Stack
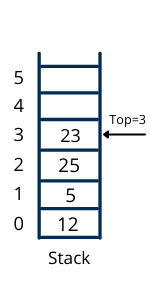
Stack Operations in Data Structure
There are below operations which we can perform on Stack
1). Push
2). Pop
3). Peek
1). Push Operation
Using push operation Individual items can be added to a stack. We can perform Push operation only at the top of the stack. However, before inserting an item in the stack we must first check if TOP=MAX–1 because if that is the case, then the stack is full and no more insertions can be done. If an attempt is made to insert a value in a stack that is already full, an OVERFLOW message is printed.
Lets see below example to understand push operation in data structure
We are going to perform PUSH operation on this stack
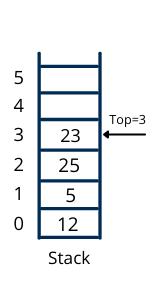
Now we want to insert an element with value 13, we first check if TOP=MAX–1. If the condition is false means there is some space in the stack, then we increment the value of TOP and store the new element at the position given by the stack[TOP]. After performing the PUSH operation our stack will look like this.
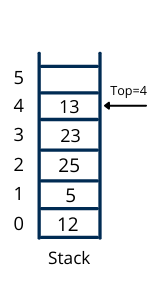
Code Snippet of PUSH Operation
public void push(int x)
{
if (top >= (MAX - 1)) {
System.out.println("Stack Overflow");
}
else {
a[++top] = x;
System.out.println("Item pushed into stack = "+x);
}
}
2). Pop Operation
Using pop operation Individual items can retrieved or removes from the stack. We can perform Pop operation only at the top of the stack. However, before deleting the value, we must first check if TOP=NULL because if that is the case, then it means the stack is empty and no more deletions can be done. If an attempt is made to delete a value from a stack that is already empty, an UNDERFLOW message is printed.
Lets see below example to understand pop operation in data structure
We are going to perform POP operation on this stack
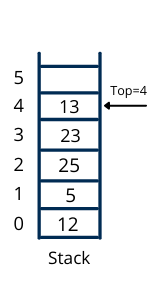
To perform pop operation first check if TOP=NULL. If the condition is false means there are some elements in the stack, then we decrement the value pointed by TOP. After performing POP operation our stack will look like.
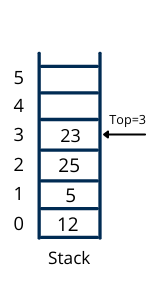
Code Snippet of POP Operation
public int pop()
{
if (top < 0) {
System.out.println("Stack Underflow");
return 0;
}
else {
int x = a[top--];
return x;
}
}
3). Peek Operation
Using peek operation topmost element of the stack will be retrieved without deleting it from the stack. However, the Peek operation first checks if the stack is empty, i.e., if TOP = NULL, then an appropriate message is printed, else the value is returned.
Code Snippet of PEEK Operation
public int peek()
{
if (top < 0) {
System.out.println("Stack Underflow");
return 0;
}
else {
int x = a[top];
return x;
}
}
When an item is added to a stack, it is placed on the top of all previously entered items. When an item is removed, it can be removed from the top of the stack. A stack in which items are removed the top is considered a “LIFO” (Last In, First Out) stack.
Stack Applications in Data Structure
1). Stack is used to tracking function calls for example Suppose we have two functions function A and function B. function A calls another function B and function B calls function C.
2). “undo” operation in text editors is an application of stack
3). Solving Infix, prefix, and postfix expression
4). Forward and backward feature in web browsers
5). Backtracking is also an application of Stack.
6). In Memory management.