C Program to insert node at Beginning of linked list
#include<conio.h>
#include<stdio.h>
#include<stdlib.h>
struct node{
int data;
struct node *next;
};
struct node *head=NULL;
struct node* createNode(){
struct node *newNode = (struct node *)malloc(sizeof(struct node));
return (newNode);
}
void insertNodeAtBegin(){
struct node *newNode, *temp;
newNode=createNode();
printf("enter the data you want to insert at begining in the list:");
scanf("%d",&newNode->data);
if(head==NULL){
head=newNode;
}
else{
temp=head;
head=newNode;
head->next=temp;
}
}
void viewList(){
struct node* temp=head;
if(temp==NULL){
printf("list is empty");
}
else{
while(temp->next!=NULL)
{
printf("%d\t",temp->data);
temp=temp->next;
}
printf("%d \t",temp->data);
}
}
int menu(){
int choice;
printf("\n 1. Give value to insert at begining");
printf("\n 2. Travesre/View List");
printf("\n 3. exit");
printf("\n Please enter your choice: \t");
scanf("%d",&choice);
return(choice);
}
void main(){
while(1){
switch(menu()){
case 1:
insertNodeAtBegin();
break;
case 2:
viewList();
break;
case 3:
exit(0);
default:
printf("invalid choice");
}
getch();
}
}
Output
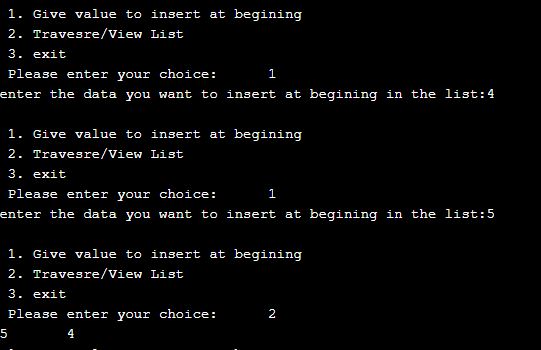
C Program to insert a node at end/tail of linked list
#include<stdio.h>
#include<conio.h>
#include<stdlib.h>
struct node{
int data;
struct node *next;
};
struct node *head=NULL;
struct node* createNode(){
struct node *newNode = (struct node *)malloc(sizeof(struct node));
return (newNode);
}
void insertNodeAtEnd(){
struct node *temp,*ptr;
temp=createNode();
printf("enter the data you want to insert:");
scanf("%d",&temp->data);
temp->next=NULL;
if(head==NULL)
head=temp;
else{
ptr=head;
while(ptr->next!=NULL){
ptr=ptr->next;
}
ptr->next=temp;
}
}
void viewList(){
struct node* temp=head;
if(temp==NULL){
printf("list is empty");
}
else{
while(temp->next!=NULL)
{
printf("%d\t",temp->data);
temp=temp->next;
}
printf("%d \t",temp->data);
}
}
int menu(){
int choice;
printf("\n 1.Add value to the list at end");
printf("\n 2.Travesre/View List");
printf("\n 3.exit");
printf("\n Please enter your choice: \t");
scanf("%d",&choice);
return(choice);
}
void main(){
while(1){
switch(menu()){
case 1:
insertNodeAtEnd();
break;
case 2:
viewList();
break;
case 3:
exit(0);
default:
printf("invalid choice");
}
getch();
}
}
Output:
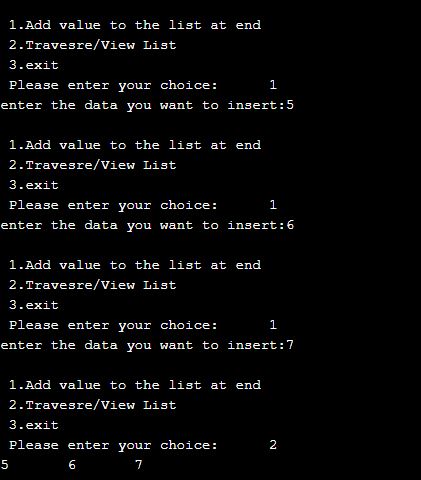
C Program to insert a node at a given location of linked list
#include<stdio.h>
#include<conio.h>
#include<stdlib.h>
struct node{
int data;
struct node *next;
};
struct node *head=NULL;
struct node* createNode(){
struct node *newNode = (struct node *)malloc(sizeof(struct node));
return (newNode);
}
void insertNodeAtEnd(){
struct node *temp,*ptr;
temp=createNode();
printf("enter the data you want to insert:");
scanf("%d",&temp->data);
temp->next=NULL;
if(head==NULL)
head=temp;
else{
ptr=head;
while(ptr->next!=NULL){
ptr=ptr->next;
}
ptr->next=temp;
}
}
void insertNodeAtLocation(){
int loc,i;
struct node *temp,*ptr, *tempprev, *tempnext;
temp=createNode();
printf("enter the data you want to insert:");
scanf("%d",&temp->data);
printf("enter the the location where you want to insert:");
scanf("%d",&loc);
temp->next=NULL;
if(loc<1 && head==NULL){
printf("invalid location");
}else{
ptr=head;
for(i=0; i<loc-1; i++){
tempprev=ptr;
ptr=ptr->next;
}
tempnext=ptr;
tempprev->next=temp;
temp->next=tempnext;
}
}
void viewList(){
struct node* temp=head;
if(temp==NULL){
printf("list is empty");
}
else{
while(temp->next!=NULL)
{
printf("%d\t",temp->data);
temp=temp->next;
}
printf("%d \t",temp->data);
}
}
int menu(){
int choice;
printf("\n 1.Add value to the list at end");
printf("\n 2.Travesre/View List");
printf("\n 3.Add value to the list at location");
printf("\n 4.exit");
printf("\n Please enter your choice: \t");
scanf("%d",&choice);
return(choice);
}
void main(){
while(1){
switch(menu()){
case 1:
insertNodeAtEnd();
break;
case 2:
viewList();
break;
case 3:
insertNodeAtLocation();
break;
case 4:
exit(0);
default:
printf("invalid choice");
}
getch();
}
}
Output
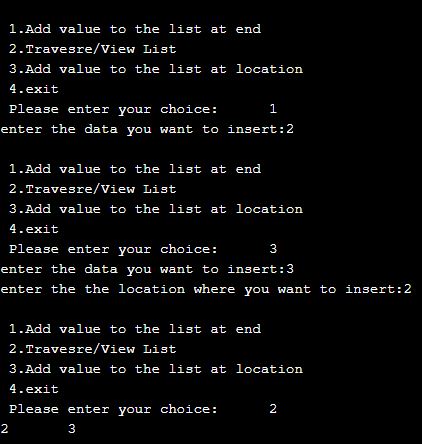